MEVN stack provides developers with a flexible solution to full-stack development, known as an alternative to MEAN and MERN stacks. MEVN utilizes Vue.js for backend development, while MEAN uses Angular, and MERN relies on React. Modern interactive web-based applications can be well developed through the lightweight design, growing community base, and exceptional flexibility of MEVN.
Developers need to know MEVN if they wish to optimize their workflow. While, businesses can apply MEVN to plan for their real-time applications and dynamic platforms. This article will explain the MEVN stack while discussing its advantages, setup requirements, common implementations, and comparison against MEAN and MERN.
>> Related Articles:
- What is MEAN Stack? A Detail Guide for Beginners
- What is MERN Stack? In-depth Explanation for Developers
What is MEVN Stack?
The MEVN stack is a JavaScript technology stack including MongoDB, Express.js, Vue.js, and Node.js, allowing to interactively create full-stack applications using a single programming language. This development stack is required to develop dynamic and scalable web applications besides MEAN and MERN stacks.
- MongoDB: An open-source and non-relational database using JSON platforms. This component is highly flexible for use in complex web-based apps.
- Express.js: This is a minimal web app development framework specifically designed for back-end development. It is ideal for fast API development and HTTP request management.
- Vue.js: A modern Javascript-based tool for developing UI and front-end. This tech stack is popular for its minimalist approach and active development.
- Node.js: An independent platform enabling JavaScript to run on the server-side which is separate from the web browser.
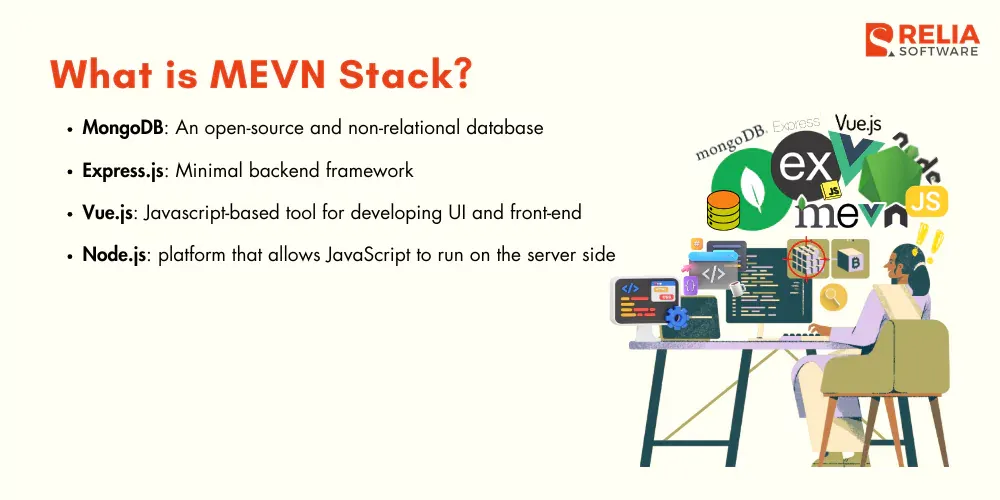
>> Read more:
- What Can You Do With JavaScript? 8 Popular JavaScript Usages
- Top 15 Node.js Projects for Beginners and Professionals
- Top 9 Best Node.js Frameworks For Web App Development
Advantages & Disadvantages of MEVN Stack
Advantages of MEVN Stack
- Unified Language: JavaScript is used across the whole stack of the system, making it easier to develop and learn than the traditional development method.
- Scalability: MongoDB and Node.js provide horizontal scaling to manage high traffic.
- Rapid Development: Since Vue.js has a component-based design, it allows for very fast prototyping and development.
- Full-Stack Capability: Frontend and backend are fully included in the stack together with a database, making it a comprehensive development solution.
Disadvantages of MEVN Stack
- Limited Use: This stack is less preffered by businesses compared to MEAN and MERN stacks. This can cause obstacles for enterprise-level support.
- Smaller Community: The Vue.js framework maintains a smaller user base compared to Angular or React, resulting in fewer third-party components and reduced educational materials.
- SEO Limitations: Vue.js requires the implementation of server-side rendering (SSR) to improve SEO function, which makes the development process more complex.
- Frequent Updates: The rapid updates in Vue.js lead to frequent updates to maintain compatibility with the latest version. These changes sometimes break their codebase.
Use Cases of MEVN Stack
- Web-Based Project Management Tools: MEVN provides the ideal environment for developers to create collaborative project management tools. They can monitor tasks and provide real-time team interaction. Moreover, this stack can efficiently deploy functionality such as task assignment, progress tracking, and real-time update capabilities.
- E-commerce Platforms: With the MEVN stack, developers can build interactive e-commerce solutions with dynamic features and scalable online store capabilities. During the development stage, programmers can integrate product lists, shopping carts, and protected payment gateways.
- Social Networking Sites: MEVN is an ideal technology for building social media platforms by its capability to manage real-time system changes. MEVN framework enables simple features, including user profiles, live chat, and activity feed functionality.
- Learning Management Systems (LMS): This stack enables developers to design platforms for online education and training. Users can efficiently stream videos, perform quizzes, and view their learning progress through the stack's adaptable features.
- Real-Time Applications: This is an ideal system for creating applications that need real-time updates, including chat systems and dashboards. It effectively builds apps that need real-time functionality, like trading systems and collaborative tools.
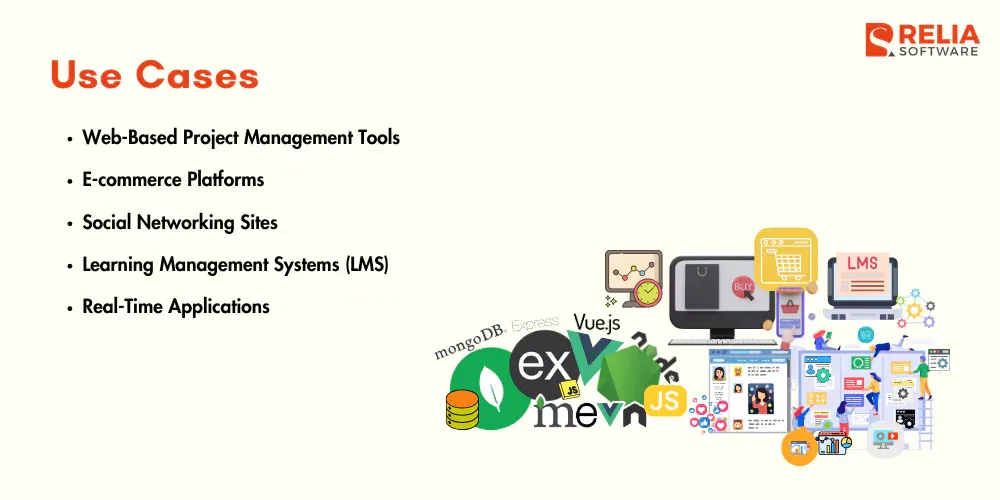
How To Set Up the MEVN Environment?
Before building an application using the MEVN stack, you have to set up the environment for developing this stack first. Setting up the MEVN stack environment is simple and involves preparing essential tools and configuring the project environment. Here’s how you can get started:
1. Required Conditions
To set up the MEVN stack, ensure you have the following:
- Node.js and npm: Needed for the execution of JavaScript on the backend and to manage dependencies.
- MongoDB: It acts as your database to hold application data based on Windows environment.
- Code Editor: Visual Studio Code is another preferred tool for rapid development.
2. Step-by-Step Installation Guide
-
Initialize Your Project: Begin by creating a directory for your project and initializing npm:
mkdir mevn-app && cd mevn-app
npm init -y
-
Install Dependencies: Install the necessary packages, including Express for the backend and Mongoose for database interactions.
-
Setup MongoDB: After installing, now you have to ensure the MongoDB server is running and create a new database for your project before starting to develop your app.
3. Configuration Tips
- Use environment variables to manage sensitive information like database credentials.
- Test the database connection after setting up MongoDB.
- Structure the project directory to separate backend and frontend files.
By following these steps, you can establish a solid foundation for your MEVN stack application.
Building a Simple Application with MEVN
When the environment has been set, now you can start establishing your application. This involves designing both the back and front end, applying the database, and combining the various components to make a functional system.
Step 1: Project Structure Overview
Developing the project plan is also significantly important in MEVN stack development. First of all, carefully define the application's functional layers or key features, such as authentication, product management, or notifications. After that, create a folder organization where each smaller directory is dedicated solely to the backend, frontend, and database algorithms. A common structure includes:
- Backend: Contains the files with Express.js server and API routes.
- Frontend: Stores Vue.js components and assets.
- Database: Responsible for configuring MongoDB and creating models.
Write down the structure and convention in a document so that you and the team can refer to it in the future. Lastly, deploy automation tools like NPM scripts that make it easy to handle frequent tasks such as testing, fixing, running linter, and other deployment tasks.
>> Read more:
- Top 22 Best DevOps Automation Tools For Businesses
- Top 10 Automated Code Review Tools For Developers
- Top 6 Automation Testing Tools for Businesses in 2025
Step 2: Backend Development with Node.js and Express.js
When building the backend with Node.js and Express.js, structure a solid server and decide on the APIs to apply in processing the application flow and access to data. First, let’s create an Express server and set it up to serve and handle requests.
const express = require('express');
const mongoose = require('mongoose');
const app = express();
app.use(express.json());
mongoose.connect('mongodb://localhost:27017/mevn-db', {
useNewUrlParser: true,
useUnifiedTopology: true
});
app.listen(5000, () => console.log('Server running on port 5000'));
Defining routes for basic CRUD operations is creating APIs for the application to communicate with the database. These routes are to retrieve, write, update, and delete data, which are the fundamental operations of the backend functionality. Furthermore, they can be planned to handle the data so that developers can implement the app smoothly.
app.get('/items', (req, res) => {
res.send('Retrieve all items');
});
app.post('/items', (req, res) => {
res.send('Create an item');
});
>> Read more:
- A Comprehensive Guide for Node.js Dependency Injection
- Guide To Node.js Enterprise Application Development
Step 3: Frontend Development with Vue.js
When building Vue.js applications, Vue CLI is used to make a form for a basic front-end application, establish template slots for common elements, and organize the app into directories of components and views. This approach makes the application maintainable and easy to scale up in the development process.
>> Read more:
- An In-Depth Guide for Front-End Development with React
- 12 Frontend Technologies and 8 Development Trends in 2025
Step 4: Database Integration with MongoDB
Getting data into a database through MongoDB integration makes developers manage the app effectively. Mongoose allows the integration of the backend to the MongoDB easily. Mongoose provides solutions for all interactions with databases in the form of schemas, which ensures structure and data validation.
Moreover, you should also use CRUD operations, including models and routes for the creation, reading, updating, and deletion of data. It not only improves the data handling ability of the program but also guarantees that as the application progresses, it can manage the data systematically.
Advanced Concepts of MEVN Stack
State Management with Vuex
Vuex is a data management library that is specifically built for Vue.js application development. It can collect common data into a state store, which delivers an effective way to manage the complicated app states within multiple components.
This feature is useful in large applications where different parts of the application require access to the same information. For instance, managing the user session data in Vuex makes the page’s component stable and manages the app changes easier.
Authentication and Authorization with JWT
JWT is one of the secure and reliable methods for user authentication and authorization in web applications when working on projects. It works by issuing a token when a user logs in, which is then included in each request to verify their identity.
In Express.js, middleware can validate these tokens to protect sensitive routes from unauthorized access. JWT also enhances security and supports scalable authentication, making it a suitable choice for modern application development.
Real-time Functionality with WebSockets
WebSockets through Socket.IO enables applications to receive regularly updated data in real time. This function provides a communication channel between users and servers without requiring many requests for exchange. Real-time functionalities exist in applications such as live chat programs, co-creation platforms, and instant real-time alerts relating to database modifications. Applications can become more responsive when providing immediate user feedback.
Deploying the application with Docker guarantees high-consistent performance whether it is deployed locally or in cloud while still maintaining the ease of scale. This platform enables developers to put into practice suitable strategies for establishing and maintaining strong applications.
Common Challenges and Solutions of MEVN Stack Development
Challenge 1: Debugging Complex Applications
Current applications have complex structures with many dependencies, making debugging challenging. Issues like unexpected component interactions or asynchronous errors can slow development and cause hidden bugs. These require extra time and effort to fix.
Solutions:
- Through Chrome DevTools, programmers can quickly find and fix frontend issues.
- Developers often employ Postman at the same time to test and debug APIs.
- For backend error tracking, frameworks like Winston help developers monitor and log errors efficiently.
Challenge 2: Performance Optimization
Large-scale applications can have poor performance because they contain low-optimized code and weak database queries. This excessive and long load time problem can cause negative problems for user experience and satisfaction.
Solutions:
- Minimizing both JavaScript and CSS files to reduce loading times.
- Multiple forms of caching including browser and server-side caching should be implemented to enhance system response speed.
- The effective use of both proper database indexing and efficient modeling techniques helps optimize MongoDB performance.
Challenge 3: Securing the Application
There are 3 major security risks in web apps, which are SQL injection, XSS vulnerabilities, and data security breaches. These security threats lead to user data exposure, damaged trust relationships, and operational disruptions. To keep the application and users safe, it's important to clean input data and use secure encryption methods.
Solutions:
- Data transmission encryption protocols should use HTTPS.
- All applications must sanitize their inputs to stop XSS attacks.
- JWT authentication and role-based access control protect API endpoints.
>> You may interested in: 10 Common Software Development Challenges & Solutions
Comparison of MEVN, MEAN, and MERN Stacks
A comparison of the MEVN, MEAN, and MERN stacks helps developers choose the most suitable stack for their projects based on specific requirements. The table below highlights key differences:
Feature |
MEVN Stack |
MEAN Stack |
MERN Stack |
Frontend Framework |
Vue.js |
Angular |
React |
Ease of Learning |
Simple and beginner-friendly |
Steeper learning curve |
Intermediate, due to React’s flexibility |
Performance |
High, suitable for lightweight apps |
High, optimized for enterprise apps |
High, ideal for dynamic UIs |
Use Cases |
Real-time apps, dashboards |
Enterprise-level web applications |
Social media platforms, SPAs |
Community Support |
Growing, smaller than React/Angular |
Established, large community |
Large, active community |
>> Read more: MERN Stack vs MEAN Stack What is Difference?
Conclusion
MEVN stack system is a modern approach to full-stack web development. This stack includes MongoDB data storage, Express.js for the backend, and Vue.js for the front end running on Node.js as the server platform. MEVN helps businesses to develop flexible and responsive website applications efficiently. However, a company that wants to maximize MEVN stack value should obtain services from skilled teams to deliver best practices and optimize performance. Therein, Relia Software offers customizable and scalable solutions, helping businesses grow and stay competitive with high-quality development and expert support.
>>> Follow and Contact Relia Software for more information!
- development
- Web application Development
- Designing an application
- web development