JavaScript is a versatile programming language used for web development. However, have you ever been curious about what you can do with JavaScript? This coding language can build mobile apps and games to complex web interfaces. It is a key web technology that lets developers build dynamic and interesting user experiences.
This article will analyze various capabilities and practical uses of JavaScript in web application development. Each usage will bring examples to make you understand clearly.
>> Read more: A Comprehensive Guide of SOLID Principles in JavaScript
Build Interactive Web Pages
The programming language JavaScript is responsible for the incorporation of responsiveness and vitality into static web pages that are generated via HTML and CSS. Web developers can build dynamic, interesting UIs that make the web possible for users to interact with it.
JavaScript runs directly in the user's browser, providing faster response times and a more fluid user interface (UI) than server-side languages. It does this by adding event listeners to buttons, forms, and links. These listeners activate JavaScript functions on clicks, typing, and hovering which change the web page's content, style, or behavior based on user activities.
JavaScript also dynamically changes text, styles, and elements in the Document Object Model (DOM). This lets users update search results as they enter or create interesting animations while hovering over an image. Overall, JavaScript connects user input to web page modifications, making them more interactive and responsive.
Tech Stack: Javascript with DOM Manipulation; Javascript Libraries (e.g., Anime.js, GSAP) and CSS Animations
-
JavaScript and the Document Object Model (DOM): Grant developers control over a web page's structure, content, and behavior. You can dynamically alter elements like headings, paragraphs, buttons, and images by manipulating the DOM. This allows for creating rich and interactive web experiences without relying solely on pre-built components offered by frameworks.
-
CSS animations: Include lightweight and direct code building blocks of webpage animation. But it is limited in how you can control them over time.
-
JavaScript libraries (Anime.js and GSAP): Grant finer control (timing, sequences), allow for user interaction triggers (clicks, scrolls), and ensure smooth animations across different browsers.
Example: Use JavaScript to change the text of a webpage including interactive animations for buttons, menus, or page transitions.
// Select the elements
const textElement = document.getElementById("text-to-change");
const buttonElement = document.getElementById("change-text-button");
// Function to change the text with a smooth transition
function changeText() {
textElement.style.transition = "opacity 0.5s ease-in-out"; // Add transition effect
textElement.textContent = "The text has been changed!";
}
// Add click event listener to the button
buttonElement.addEventListener("click", function() {
changeText();
// Add animation class to button on click (optional)
buttonElement.classList.add("button-animation");
});
// Optional animation style for button (add to your CSS)
.button-animation {
animation: button-bounce 0.5s ease-in-out;
}
// Optional animation keyframes for button bounce (add to your CSS)
@keyframes button-bounce {
0% { transform: scale(1); }
50% { transform: scale(1.1); }
100% { transform: scale(1); }
}
Develop Web Apps (SPAs & PWAs)
JavaScript libraries like React, Angular, and Vue.js can make Single-Page Applications (SPAs) without complicated functions. SPAs through JavaScript make the user experience smooth and responsive by loading one HTML page and updating the content automatically in the background. It can reduce page reloads, speed up interactions, and introduce compelling transitions.
Tech Stack: Javascript Frameworks such as React, Angular, and Vue.js
-
React: Provides a component-based, adaptable architecture that is appropriate for intricate and expansive applications.
-
Angular: Offers a more structured and assertive approach that is perfect for enterprise-level applications that require robust scalability and maintainability.
-
Vue.js: Has a gentle learning curve and ease of usage that is popular for smaller projects or developers who are unfamiliar with Javascript frameworks.
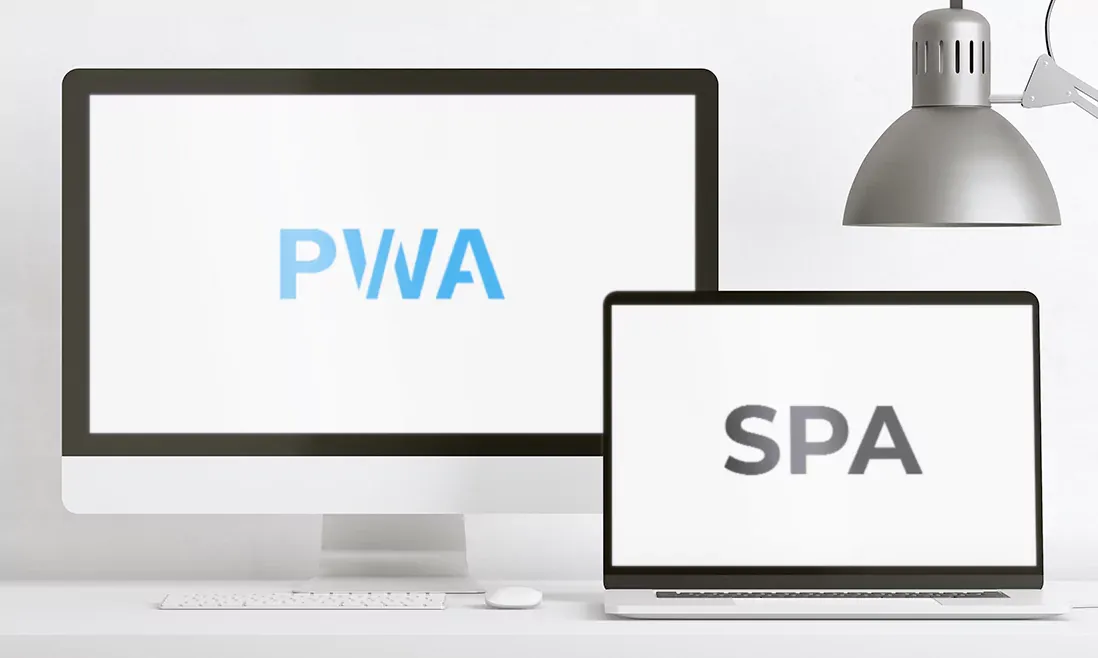
>> Read more:
-
Next.js vs React: How to Choose the Right Framework?
-
Next.js vs Angular: What Are The Differences?
-
React vs Angular: A Comprehensive Side-by-Side Comparision
Develop Mobile Apps
JavaScript can be used to make hybrid mobile apps with frameworks such as React Native and Ionic. Compared to making different native apps for iOS and Android, developers can develop code faster and reach more users by using a single codebase written in JavaScript. Programs can be deployed across platforms for faster mobile app development and broader reach.
Tech Stack: Javascript Frameworks (e.g., React Native, Ionic)
-
React Native: Develop the user interface by using JavaScript and React components. These components are then translated into native elements for each platform, such as views on iOS and Android.
-
Ionic: Provide tools and a collection of pre-built UI components to facilitate development. It also integrates with Capacitor or Cordova, which act as bridges between your JavaScript code and the device's native functionalities (e.g., camera, GPS).
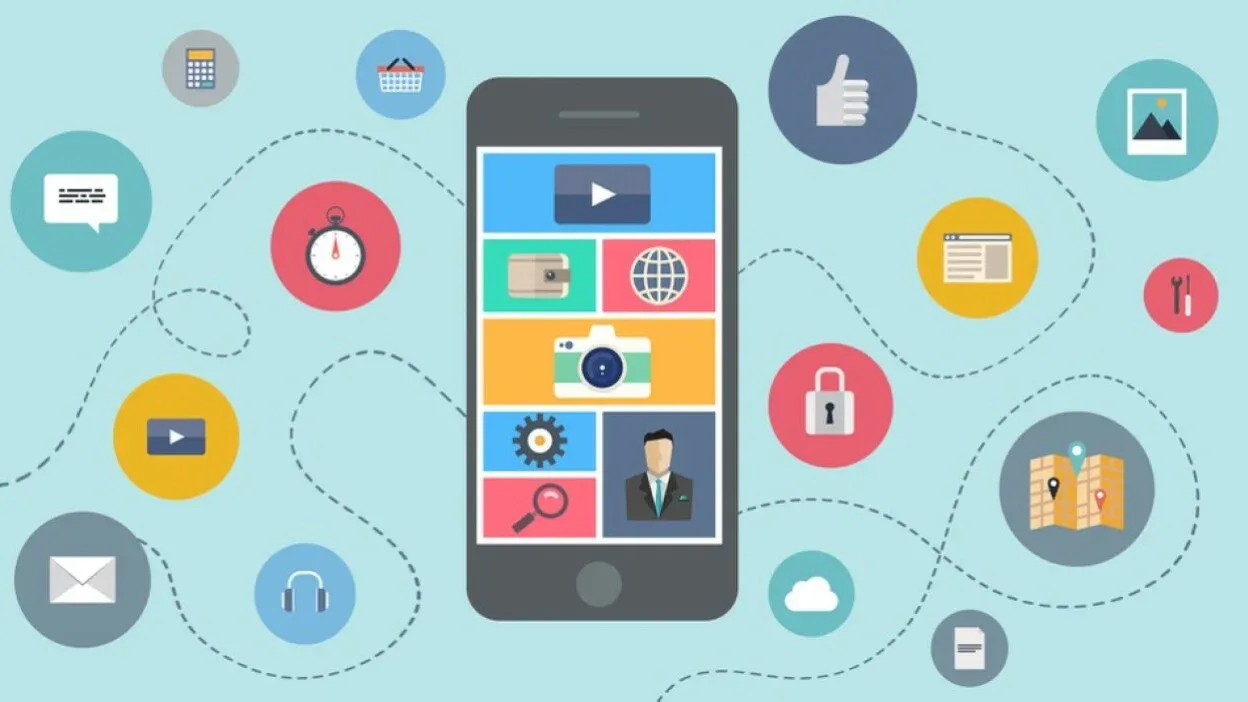
>> Read more:
-
22 React Project Ideas For Developers of All Levels
Create 2D and 3D Games
JavaScript is the foundation for establishing the logic and mechanics of a game. JavaScript Game Engines simplify game development by providing pre-built features and tools for common tasks like physics simulation, graphics rendering, animation, and collision detection. It allows developers to focus on creating the unique aspects of their game instead of building everything from scratch.
Some JavaScript Game Engines such as Phaser, PixiJS, and Babylon.js let developers create reasoning, animation, and interaction in 2D and 3D games. For more complicated games, WebGL, a JavaScript API, may be used with other programming languages like C# to make the 3D images run quickly.
Tech Stack: Javascript Game Engines
-
Phaser and PixiJS: Let developers focus on JavaScript game logic, which speeds up the process of making different types of 2D games.
-
Babylon.js: Write code that controls how the game works with realistic graphics and faster-complicated controls.
-
WebGL: Give smooth control over 2D and 3D graphics for the best performance, but it takes longer to use than pre-built engine functions.
Example: Develop a 2D game object like a platformer or puzzle game using a Javascript game engine.
function create() {
// Create the player sprite
this.player = this.physics.add.sprite(100, 100, "player");
// Create platforms (assuming you loaded a "ground" image)
const ground = this.physics.add.staticImage(400, 550, "ground");
// Set physics properties for player and platforms
this.player.setBounce(0.2); // Player bounces on collision
ground.setImmovable(true); // Ground is a static object
// Enable cursor keys for player movement
this.cursors = this.input.keyboard.createCursorKeys();
}
Visualize Data
JavaScript turns static charts into live playgrounds where users can automatically filter data, sort in on details, and make interactive charts and graphs animations. Moreover, JavaScript also lets changes happen dynamically based on user interaction to customize the chart design and work with other web techniques to update real-time data.
By prepping data and using charting libraries for different kinds of visualizations, it also transforms raw data into insights for web-based data exploration, but not for sophisticated activities.
Tech Stack: Javascript Libraries (e.g., Chart.js, D3.js, DataTables, Plotly.js)
-
Chart.js: Make common chart types (like bar, line, and pie charts) with customization options for colors, styles, and animations. It’s great for projects that don't need charts that are too complicated.
-
D3.js: Allow you to make charts such as network graphs and heatmaps. It is ideal for complicated jobs requiring extensive control over the visual representation of data.
-
DataTables: Help manage large datasets by offering features like sorting, filtering, pagination, searching, and data formatting, making it easier to explore and analyze vast amounts of information.
-
Plotly.js: Create visualizations such as zooming, panning, and hovering over data points, unlocking hidden details, and transforming exploration into an interactive story.
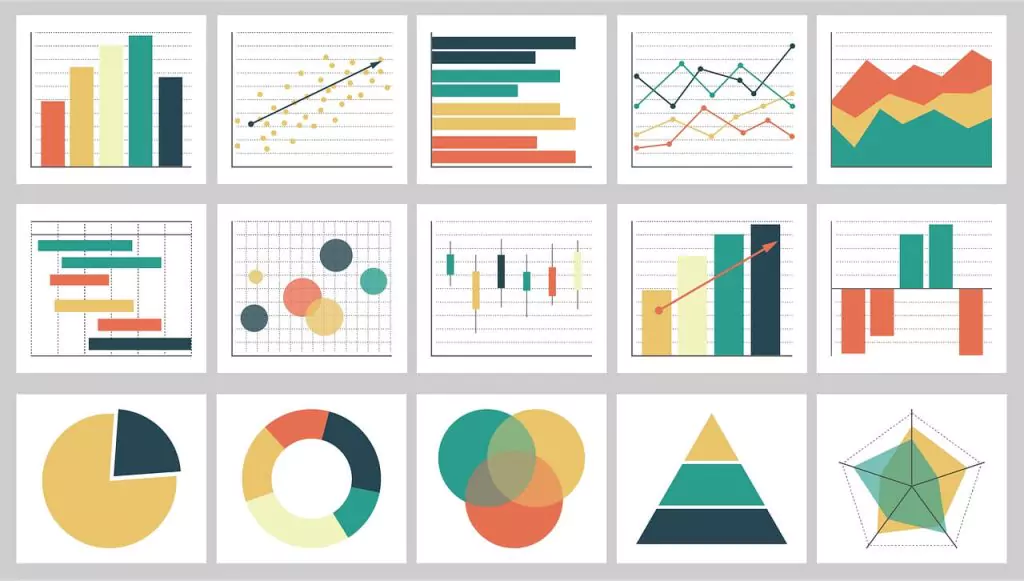
Design Smart Watches
Simple JavaScript frameworks such as Pebble.js and Bangle.js are open-source frameworks that help programmers create apps for smartwatches. Moreover, you can wirelessly upload programs or document code to your watch on Web Browser like Chrome, Opera, or Edge. You can write code using JavaScript or Blockly (a visual programming language) and wirelessly upload programs directly from your web browser.
Tech Stack: JavaScript frameworks (e.g. Pebble.js, Bangle.js)
-
Pebble.js: Let developers utilize JavaScript for user interfaces and a core C application on the watch for key functions.
-
Bangle.js: Give developers more control and works with always-on displays, built-in sensors, and lower power consumption smartwatches than some Pebble models.
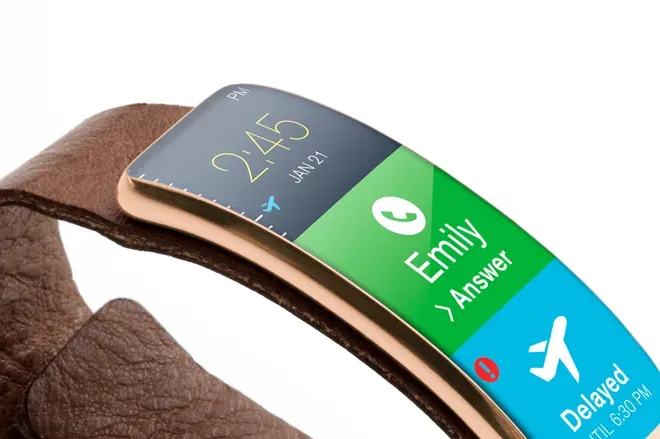
Create Digital Art
JavaScript isn't just for building websites - it can also be a powerful tool for artistic expression! Using HTML5 in JavaScript, generating images on a web page has gotten much easier. All two-dimensional and three-dimensional shapes can be readily sketched on a canvas, allowing the browser to serve as a new platform for a wide variety of digital art projects.
The JavaScript library p5.js enables programmers to make stunning artwork using only code. The library allows us to create everything from simple sketches to incredibly complex art pieces.
Tech Stack: JavaScript library (p5.js)
Make Flying Quadcopter
If you're interested in robotics or drones, JavaScript can also construct a web-based drone using a rudimentary operating system with Node.js. With Node.js and JavaScript, you can fly and control your drone using a device or computer using demos online.
On the other hand, shortcomings of JavaScript such as real-time performance difficulty and hardware access limit make it unsuitable for quadcopter control.
-
Real-time Performance: JavaScript mostly works in web browsers, which are not designed for real-time processing to handle a quadcopter precisely. If you wait too long between sending commands and getting a reaction from the quadcopter, it could be unstable and unsafe for a flying quadcopter.
-
Limited Access to Hardware: JavaScript in web browsers doesn't have full access to hardware like the flight controls and sensors (gyroscope, accelerometer) needed to fly a quadcopter. Several flight controls have firmware that can be customized for flight behavior. This might be done with C or Python.
However, there are alternatives such as Arduino Raspberry Pi C++ or Python microcontrollers for real-time control. Flight controller firmware can be customized for flight behavior using C or Python.
Tech Stack: Node.js framework, C/C++ or Python (for alternative)
>> Read more: Top 15 Node.js Projects for Beginners and Professionals
Conclusion
In conclusion, the blog has given answers to the question “What can you do with JavaScript?”. In short, JavaScript can construct dynamic and interactive web experiences for user-friendly interfaces, data visualization, and smart applications. Using JavaScript's features effectively can help your web experiences stand out in today's digital world.
>>> Follow and Contact Relia Software for more information!
- development