As JavaScript applications grow in complexity, it can be challenging to maintain and scale the codebase. This is where SOLID principles, a set of 5 object-oriented design principles, come into play. By following these principles, you can write cleaner, more maintainable, and scalable JavaScript code.
In this blog, we'll unpack each of the principles in SOLID and look at how they might be applied to JavaScript.
>> Read more: What Can You Do With JavaScript? 8 Popular JavaScript Usages
What are SOLID Principles in JavaScript?
The SOLID principles in JavaScript encompass a set of five design rules that foster the development of cohesive, well-structured, and easily maintainable code. These principles advocate for clear separation of concerns, promoting code that is flexible and readily extensible through the addition of new features without modifying existing code.
By adhering to SOLID principles, JavaScript developers can construct classes and modules that are highly focused on specific functionalities, while also enabling expansion with new features. Furthermore, SOLID principles emphasize the use of abstractions over concrete implementations, leading to cleaner, more scalable, and more testable codebases.
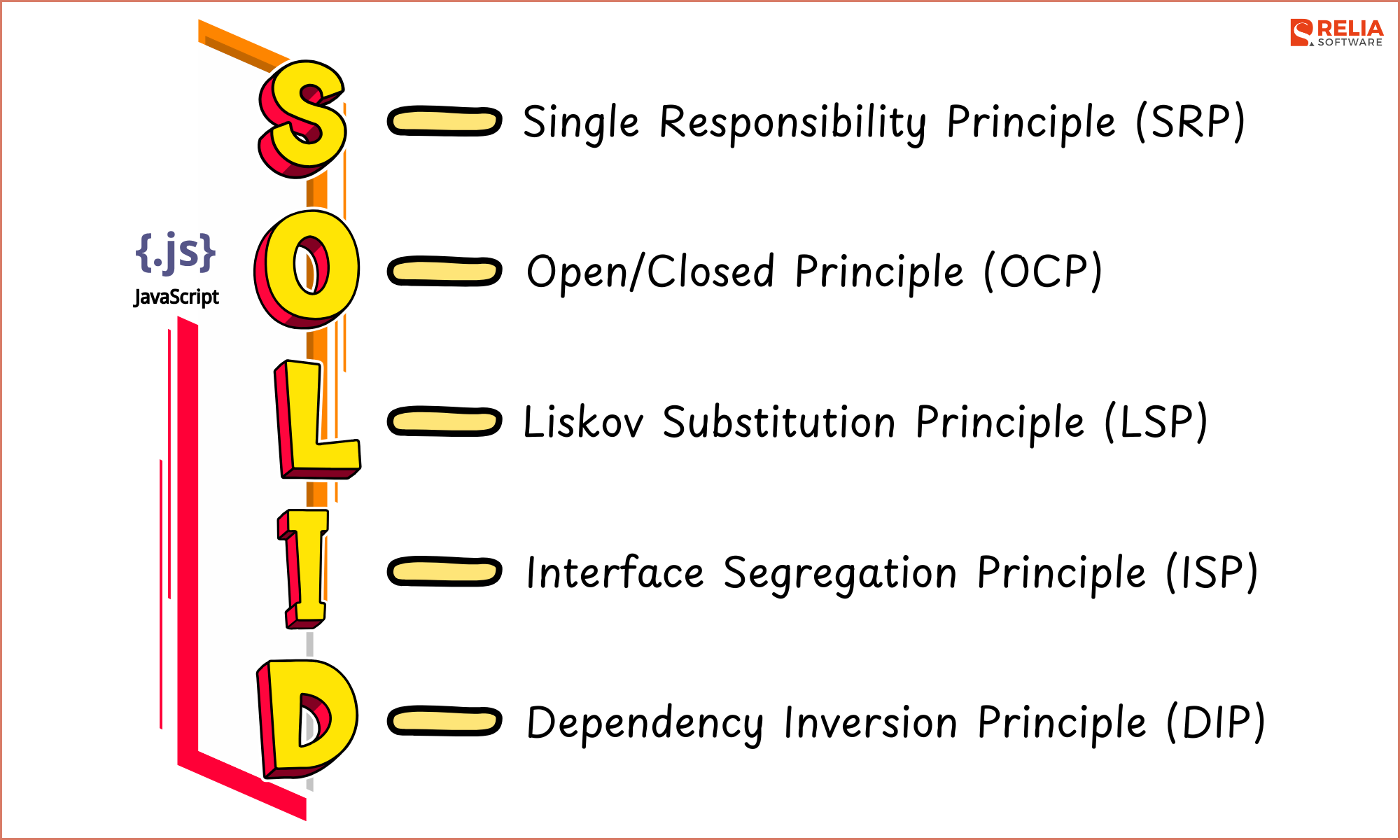
Applying Each of SOLID Principles in JavaScript Code
Single Responsibility Principle (SRP)
Single Responsibility philosophy (SRP) is a key object-oriented design philosophy that applies to the JavaScript framework. It requires classes and modules to have one clear purpose. This means the class should be good at one thing and avoid other functions. A good separation of concerns in classes makes code clearer and more maintainable.
Benefits
-
Improved Maintainability: Code with a single responsibility is simpler to comprehend, alter, and debug. Changes to one class component are less likely to disrupt other functions.
-
Enhanced Reusability: SRP encourages the design of smaller, more focused classes that can be reused across your application.
-
Reduced Complexity: Classes with a single responsibility are simpler and easier to reason, resulting in a more manageable code base.
Example of SRP in JavaScript
There is a class called UserManager that handles both registering users and proving their identity. This goes against SRP because it has two different duties. To make it follow SRP, here's how we can change it:
// Old Version (Violates SRP)
class UserManager {
registerUser(userData) {
// Registration logic
}
authenticateUser(username, password) {
// Authentication logic
}
}
// New Version (Following SRP)
class UserRegistration {
registerUser(userData) {
// Registration logic
}
}
class UserAuthentication {
authenticateUser(username, password) {
// Authentication logic
}
}
Open/Closed Principle (OCP)
Open/Closed Principle (OCP) emphasizes open expansion but closed change of software entities (classes, modules). Simply put, it means that you can add new functionality to your code without changing it. OCP encourages a design strategy that preserves basic functions while extending behavior through inheritance, composition, or other methods.
Benefits
-
Increased Flexibility: With OCP, you may simply add new features without rewriting current code, making your application more responsive to changing requirements.
-
Improved Maintainability: Code alterations are minimized, lowering the chance of introducing errors and simplifying maintenance tasks.
-
Improved Code Reusability: You can develop reusable components that can be upgraded with additional functionality without changing the basic logic.
Example of OCP in JavaScript
Let's look at a basic PaymentProcessor class that currently processes credit card payments. Following the OCP, we want to design it so that new payment methods (such as e-wallets) can be added without altering the present code. Here's how we can accomplish this:
// Old Version (Closed for Extension)
class PaymentProcessor {
processCreditCardPayment(cardDetails, amount) {
// Credit card payment logic
}
}
// New Version (Following OCP) - Using Interface
interface PaymentMethod {
processPayment(amount);
}
class CreditCardPayment implements PaymentMethod {
processPayment(amount) {
// Credit card payment logic
}
}
class PaymentProcessor {
constructor(private paymentMethod: PaymentMethod) {}
processPayment(amount) {
this.paymentMethod.processPayment(amount);
}
}
// Adding a new e-wallet payment method
class EwalletPayment implements PaymentMethod {
processPayment(amount) {
// E-wallet payment logic
}
}
const creditCardProcessor = new PaymentProcessor(new CreditCardPayment());
const ewalletProcessor = new PaymentProcessor(new EwalletPayment());
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle (LSP) requires superclass objects to be replaceable with subclass objects without affecting program correctness. In simpler terms, if you have a base class and derived classes, you can use a derived class wherever you would use the base class and expect the program to perform as intended.
Benefits
-
Improved Reliability: Code that adheres to LSP is more dependable since unexpected behavior caused by subclass substitutions is reduced.
-
Enhanced Code Maintainability: Classes developed with LSP in mind are easier to understand and maintain since subclasses consistently behave within the constraints set by the base class.
-
Increased Code Reusability: LSP encourages the development of well-defined base classes that can be consistently extended with subclasses, resulting in more reusable components.
Example of LSP in JavaScript
Imagine we have a base class Shape with a calculateArea() function that calculates shape area. Consider a Shape-derived class Square. If Square's calculateArea() method misses a multiplication by 2, utilizing a Square object instead of a Shape object will yield inaccurate results. LSP is violated.
class Shape {
calculateArea() {
// Implement area calculation logic for the specific shape
}
}
class Square extends Shape {
constructor(private sideLength) {
super();
}
calculateArea() {
return this.sideLength * this.sideLength;
}
}
// Using Square object where a Shape object is expected (Valid substitution)
const square = new Square(5);
const totalArea = square.calculateArea(); // totalArea will be 25
Interface Segregation Principle (ISP)
The Interface Segregation Principle (ISP) encourages smaller, more focused interfaces over big, general-purpose ones. It states that clients (classes or modules) shouldn't depend on unneeded methods. This idea recommends splitting huge interfaces into smaller, functional interfaces. Following ISP improves code organization and reduces application dependencies.
Benefits
-
Improved Code Maintainability: Smaller interfaces are easier to comprehend, maintain, and change as your program expands.
-
Reduced Coupling: Classes rely solely on the functions they require, resulting in a more loosely connected codebase. This streamlines the testing and debugging process.
-
Enhanced Flexibility: Smaller interfaces allow you to design more flexible components that can be combined to achieve a variety of functions.
Example of ISP in JavaScript
Consider a huge interface called Logger that includes methods for logging messages with varying severity levels (debug, info, warn, error). Now, a class may just need to log informational messages. Following ISP, we can divide the logger interface into smaller ones.
// Old Version (Large Interface)
interface Logger {
debug(message);
info(message);
warn(message);
error(message);
}
// New Version (Following ISP) - Smaller Interfaces
interface InfoLogger {
info(message);
}
interface ErrorLogger {
warn(message);
error(message);
}
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle (DIP) is an object-oriented programming principle in Javascript asserting that high-level modules should not rely on low-level modules directly, but rather on abstractions which should determine concrete implementations.
This philosophy promotes high-level modules to declare dependencies based on functionality, not implementation. Abstractions provide a compromise for switching concrete implementations.
Benefits
-
Enhanced Testability: Using abstractions allows you to quickly simulate or inject dependencies during testing, making unit testing of high-level modules more efficient.
-
Loose Coupling is Improved: Since high-level modules are no longer connected to specific implementations. This streamlines code upgrades and maintenance.
-
Increased Code Reusability: Abstractions encourage the development of reusable components that can be readily merged into various implementations based on requirements.
Example of DIP in JavaScript
Let's say that an EmailService class needs a real SMTPSender class to send emails. This goes against DIP because the EmailService is tightly connected to a certain application. Using DIP, here's how we can change it:
// Old Version (Violates DIP)
class EmailService {
constructor(private smtpSender = new SMTPSender()) {}
sendEmail(emailData) {
this.smtpSender.send(emailData);
}
}
// New Version (Following DIP) - Using Interface
interface EmailSender {
send(emailData);
}
class SMTPSender implements EmailSender {
send(emailData) {
// Send email using SMTP
}
}
class EmailService {
constructor(private emailSender: EmailSender) {}
sendEmail(emailData) {
this.emailSender.send(emailData);
}
}
// Now you can easily inject different EmailSender implementations
const transactionalEmailService = new EmailService(new TransactionalEmailSender());
Relationships Between SOLID Principles
These SOLID principles are interrelated and work together to create a robust and maintainable codebase. For instance, the SRP ensures focused classes, which can then be more easily extended following the OCP. Similarly, adhering to LSP promotes the creation of reusable components that can be utilized interchangeably, aligning with the goals of ISP and DIP.
By understanding these principles and their interactions, you can write cleaner, more maintainable, and adaptable JavaScript code.
5 Tips For Applying SOLID Principles to JavaScript Projects
SOLID principles can significantly improve the maintainability, readability, and testability of your JavaScript codebase. However, applying them to existing projects can seem daunting. Here are five practical tips to help you gradually integrate SOLID principles into your current code:
1. Start Small and Focus on Pain Points: Don't attempt a complete overhaul at once. Identify areas within your codebase that are challenging to maintain or have become overly complex due to multiple functionalities. Prioritize refactoring these sections to adhere to SOLID principles.
2. Refactor Incrementally: If you encounter large classes or code segments that violate SOLID principles, break them down into smaller, more manageable components that comply with these principles. Begin by refactoring smaller sections in isolation and gradually work towards a more comprehensive implementation of SOLID principles throughout your codebase.
3. Balance Improvement with Maintainability: The primary objective is not to achieve code perfection but to enhance maintainability and testability. Refactor in small, manageable chunks and prioritize areas that will yield the most significant impact.
4. Test Thoroughly After Refactoring: Following any code refactoring, conduct rigorous testing to ensure no accidental functionality breakage. Unit tests become even more valuable when using JavaScript frameworks and dependency injection (DIP).
5. Continuous Improvement: Regularly conduct code reviews with a focus on identifying opportunities to further enhance adherence to SOLID principles.
By following these tips and gradually incorporating SOLID principles, you can significantly improve the maintainability, flexibility, and overall quality of your JavaScript codebase.
>> Read more information about Javascript frameworks:
- React vs Angular: A Comprehensive Side-by-Side Comparision
- Next.js vs React: How to Choose the Right Framework?
- Next.js vs Angular: What Are The Differences?
- Mastering React Test Renderer for Streamlined Testing
- Top 10 Best Open Source React & React Native IDEs
- An In-Depth Guide for Front-End Development with React
- Top Frontend Technologies and Development Trends in 2025
Conclusion
You can build durable, adaptive, and future-proof apps by following SOLID principles in JavaScript. These concepts may need initial restructuring and coding attitude changes. SOLID principles in JavaScript mastery is a lifelong process, but the benefits are worth it!
Hope this guide helps you start using SOLID principles in JavaScript development better!
>>> Follow and Contact Relia Software for more information!
- development