As a developer, we've all encountered massive codebases that feel really exhausted. But what if there was a way to structure software that's not only powerful but also intuitive?
Enter Object-Oriented Programming (OOP)! It's a paradigm that breaks down complex systems into manageable objects, mirroring real-world entities. This approach enhances code readability and promotes reusability and maintainability. Let's delve into the core concepts of OOP and see how it can transform your development workflow.
>> Read more: Differences Between Procedural & Object-Oriented Programming
What is Object-Oriented Programming?
Object-oriented programming (OOP) is a way of structuring software around self-contained objects that combine data (properties) and the actions (methods) that operate on that data.
Now that we've grasped the essence of OOP, let's delve into the fundamental building blocks that make it tick:
Objects
Objects act as the actors in your software play. Each object represents a real-world entity, like a car, a user, or a product. They encapsulate data (think properties or attributes) that define their characteristics, such as a car's color, a user's name, or a product's price.
But objects aren't passive bystanders! They also possess functionalities (methods or functions) that dictate how they interact with the world. A car object can accelerate, a user object can login, and a product object can be added to a shopping cart.
Classes
Classes act as blueprints for creating objects. They define the properties (data type) and methods (functionality) that all objects of a particular type will share.
Think of a class as a cookie cutter for objects – it specifies the shape (properties) and the actions (methods) they can perform. For instance, a "Car" class might define attributes like color and model, and methods like accelerate() and brake().
Attributes
These are the data points or characteristics that define an object's state. They provide the details about an object, such as a car's color being "red" or a user's name being "Alice". Attributes are essentially variables specific to an object, allowing you to store and manipulate its data.
Methods
These are the functions or actions that objects can perform. They dictate how objects interact with the world and other objects. A car object's accelerate() method might increase its speed, while a user object's login() method might verify their credentials. Methods operate on the object's attributes, allowing you to modify or utilize its data.
4 Main Principles of Object-Oriented Programming
Now that we have objects with their own properties and functionalities, let's explore how they can interact and build complex systems:
Inheritance
This allows you to create new classes (subclasses) that inherit properties and behaviors (methods) from existing classes (superclasses).
Suppose that there is a "SportsCar" class that inherits properties and methods from a more general "Car" class. This allows the "SportsCar" to reuse existing functionality (accelerate, brake) while adding its own unique features (turbo boost). Inheritance promotes code reusability and creates a hierarchy of related objects.
Encapsulation
This concept focuses on protecting an object's internal data (attributes) by controlling access through methods. Think of it as a secure vault for the object's data. Encapsulation ensures data integrity and prevents unauthorized modifications.
Polymorphism
This powerful concept allows objects of different classes to respond differently to the same message. For instance, a "draw()" method might display a car on the screen, but it could also display a user profile picture or a product image, depending on the object it's called on. Polymorphism adds flexibility and simplifies code.
Abstraction
This concept focuses on hiding unnecessary details and exposing essential functionalities. Methods act as the interface, allowing us to interact with objects without worrying about their internal workings. Abstraction promotes code clarity and maintainability.
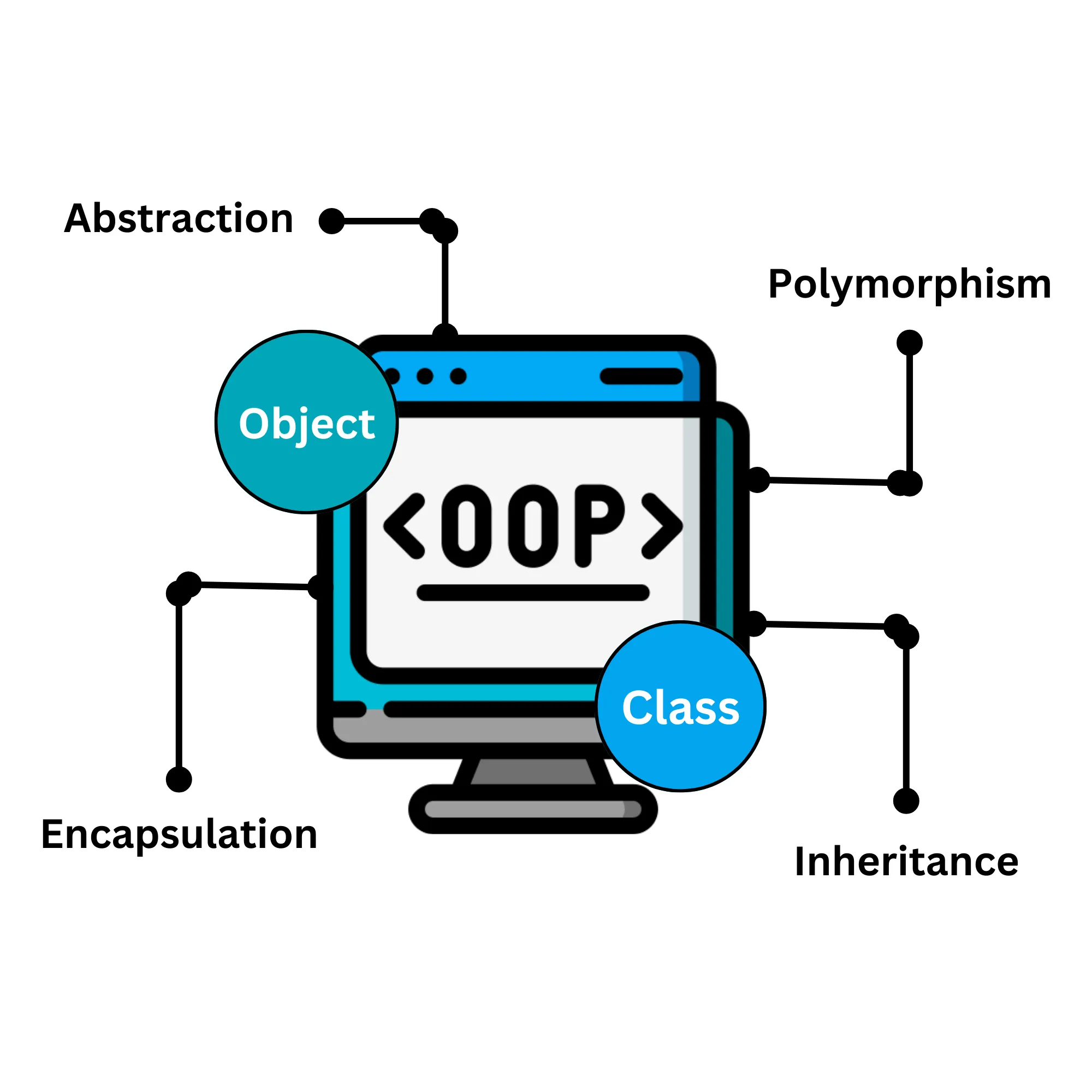
Implementing Object-Oriented Programming with A Practical Example
Let's see OOP in action! We'll use a relatable example to demonstrate how OOP principles translate into practical code.
Example: Suppose that we're creating a car simulation game.
Here's how OOP concepts can be applied:
- Class: We can define a
Car
class that acts as the blueprint for creating car objects. - Attributes: The
Car
class might have attributes likecolor
,model,
speed
, andfuelLevel
. - Methods: We can define methods like
accelerate()
,brake()
,turnLeft()
, andgetFuelLevel()
within theCar
class. These methods will operate on the car's attributes, simulating real-world car behavior.
Creating Car Objects: Using the Car
class, we can create individual car objects with unique properties. For example:
public class Car {
private String color;
private String model;
private int speed;
private int fuelLevel;
public Car(String color, String model) {
this.color = color;
this.model = model;
this.speed = 0;
this.fuelLevel = 70;
}
public void accelerate() {
this.speed += 10;
this.fuelLevel -= 2;
}
public void brake() {
this.speed -= 5;
}
public void turnLeft() {
System.out.println("Car turning left!");
}
public int getFuelLevel() {
return this.fuelLevel;
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car("red", "SportsCar");
myCar.accelerate();
System.out.println("Car speed: " + myCar.speed + " km/h");
myCar.turnLeft();
System.out.println("Car fuel level: " + myCar.getFuelLevel() + "%");
}
}
Via the example above, we can infer that:
- By using classes and objects, we create modular and reusable code. The
Car
class can be used to create various car objects with different properties while sharing the same functionalities. - Modifying car behavior becomes easier. We can update methods within the
Car
class without affecting other parts of the code that interact with car objects. - As our game grows, we can easily add new types of vehicles (trucks, motorcycles) by creating subclasses that inherit from the
Car
class.
This is just a basic example, but it showcases how OOP principles can be applied to structure real-world entities and their interactions in software development.
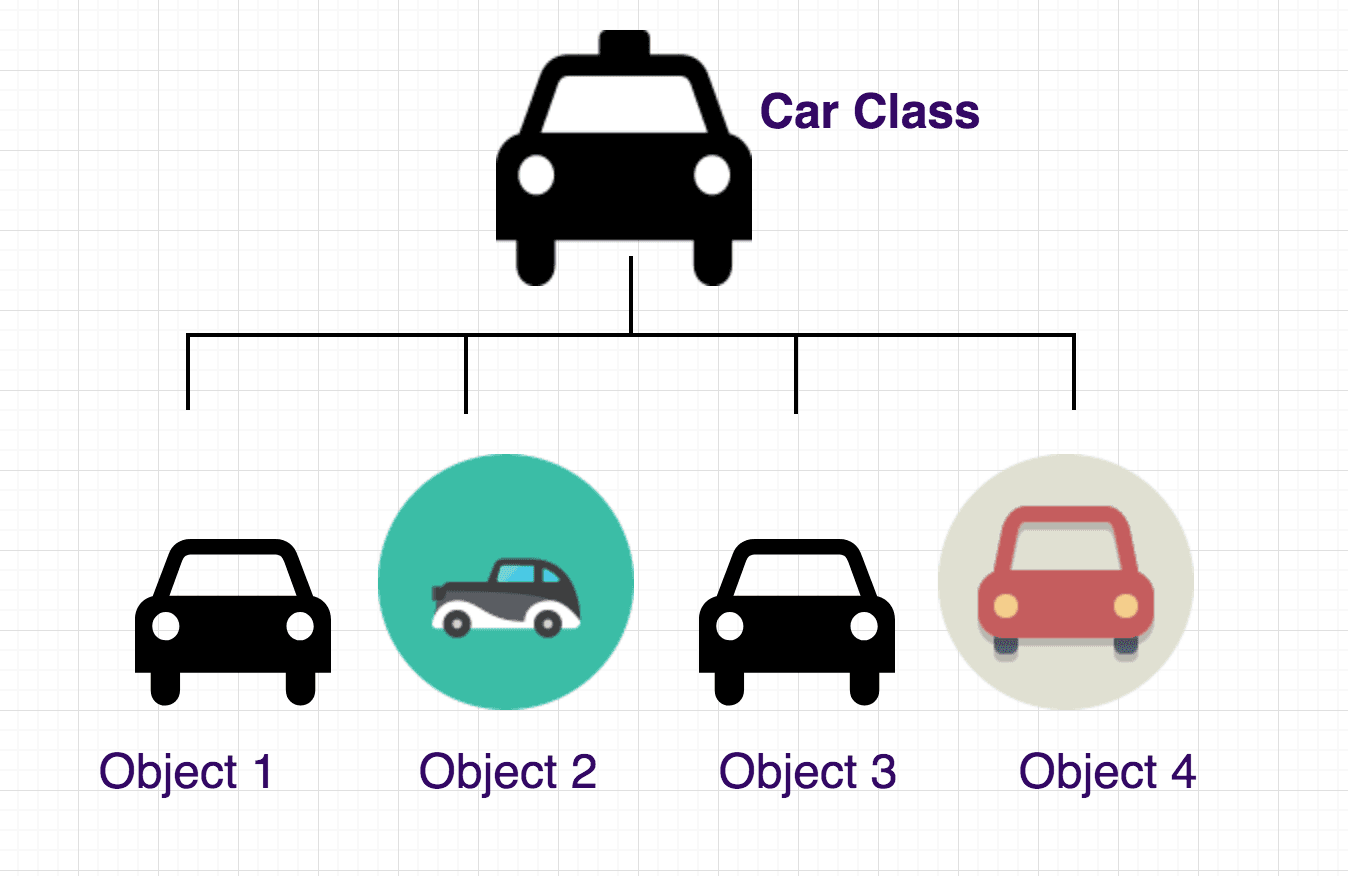
Benefits of Object-Oriented Programming
Object-Oriented Programming (OOP) offers a powerful approach to software development, bringing several advantages to the table. Here's a breakdown of some key benefits that make OOP a popular choice for many programmers:
Modular and Reusable Code
OOP promotes code modularity by organizing software around self-contained objects. These objects encapsulate data and functionality, making them reusable across different parts of your program.
Maintainability
Since objects are self-contained units, modifying code becomes easier. Changes made within an object are less likely to break other parts of the program, promoting cleaner and more maintainable codebases. As your project grows, OOP structures make it easier to understand and update individual components.
Scalability and Flexibility
OOP allows for easier extension and growth of your software. Inheritance, a core OOP concept, lets you create new classes (subclasses) that inherit properties and behaviors from existing ones (superclasses). This promotes code reusability and enables you to build complex systems by layering functionalities on top of each other.
Data Protection and Encapsulation
OOP promotes data protection through encapsulation. Data within an object is often hidden (private) and accessed through controlled methods (public). This prevents accidental modification of crucial data, ensuring the integrity and consistency of your program's state.
Real-World Modeling
OOP allows you to model real-world entities using objects. This makes your code more intuitive and easier to understand, especially for complex systems that mirror real-world interactions.
Improved Code Readability
By separating data and functionality into well-defined objects and classes, OOP promotes cleaner and more readable code. This improves code clarity for both you and other developers working on the project, making it easier to understand and maintain in the long run.
7 Popular Object-Oriented Programming Languages
Let's delve into the world of programming languages that bring OOP concepts to life. Many popular languages embrace OOP, each with its own strengths and weaknesses:
Java
A versatile and widely used language known for its robust OOP features. Java enforces strict object-oriented principles, making it a great choice for large-scale enterprise applications.
Python
A popular and beginner-friendly language that also excels in OOP. Python offers a clear and concise syntax, making it easier to grasp object-oriented concepts while still providing powerful features like inheritance and polymorphism.
C++
A powerful and mature language offering a high degree of control for experienced programmers. C++ allows for fine-grained object-oriented design, but with this power comes complexity. It might be a steeper learning curve for beginners compared to Java or Python.
C#
Developed by Microsoft, C# is another strong contender in the OOP arena. It shares similarities with Java and C++, offering a balance between flexibility and control for building various software applications.
JavaScript
While traditionally known for web development, JavaScript has embraced object-oriented concepts in recent years. Modern JavaScript frameworks heavily utilize objects and classes, making it a relevant choice for building interactive web applications.
Ruby
A popular language known for its elegant syntax and focus on developer productivity. Ruby provides a clean and expressive way to implement object-oriented principles, making it a favorite among web developers.
PHP
A widely used language for server-side scripting, particularly web development. PHP offers a robust set of OOP features, making it suitable for building dynamic and scalable web applications.
This section provides a springboard for further exploration. Many other languages, like Swift (for iOS development), Kotlin (for Android development) and Go, also incorporate object-oriented features. There's no single "best" language for OOP. The optimal choice depends on your specific needs and experience level.
>> Read more:
- Android vs IOS Development: Which Is The Best Choice?
- Top 10 PHP Web Development Companies in Vietnam
Conclusion
Congratulations! You've explored the fundamental concepts of Object-Oriented Programming (OOP). OOP offers a powerful paradigm for tackling complex problems. By organizing code around self-contained objects, you can promote code reusability, improve maintainability, and build scalable software systems.
This journey into OOP has just begun! As you delve deeper, you'll discover more advanced concepts and explore powerful languages that implement these principles. Keep practicing, keep exploring, and happy coding!
>>> Follow and Contact Relia Software for more information!
- development
- Mobile App Development
- web development