When testing software, it's successful when all the individual components connect and work as a whole. To achieve that, developers use integration testing - an important step to ensure those connections are smooth, reliable, and ready for use. This blog will explore what integration testing is, its approaches, benefits, and more.
>> Read more: Exploring the Importance of Software Testing: 5 Key Benefits
What is Integration Testing?
Integration testing is a software testing process that checks how individual modules or components of an application work together as a group. Its goal is to find and fix defects in the interactions between these components, such as incorrect data exchanges, API mismatches, or interface issues. Thus, the system is more reliable and seamless.
Integration testing happens after unit testing and before validation testing. It groups modules that are passed unit testing into larger sets, and applies predefined test cases from the integration test plan to those groups. The output is then passed on for system integration.
Integration Testing Example
You’re developing an e-commerce application with the following modules:
- User Authentication Module: Handles user login and registration.
- Product Catalog Module: Shows product details and categories.
- Shopping Cart Module: Lets users add items and view totals.
- Payment Gateway Module: Processes payments through third-party services.
Test Case: Test if a user can log in, add items to their cart, and successfully complete a payment.
Steps:
- Log In: The user logs in using valid credentials. This triggers the authentication module, which generates a session token and passes it to other modules.
- Add to Cart: The logged-in user picks a product from the catalog, and the product details (e.g., price, ID) are sent to the shopping cart module.
- Checkout: The cart calculates the total and sends it to the payment gateway.
- Payment: The payment gateway processes the amount and sends back a success or failure response.
Expected Outcome:
- The session token is passed correctly between modules.
- Product details are accurately added to the cart.
- The payment gateway processes the correct total amount.
- The payment is processed successfully, and the order is confirmed.
Issues Can be Found:
- The session isn’t passed correctly, so the cart doesn’t update.
- The product price in the catalog doesn’t match the cart total.
- The payment fails because the data sent to the gateway is incomplete.
Why is Integration Testing Important?
Although each module is passed ịn unit testing, it’s still necessary to do integration tests for the following reasons:
- Different Coding Styles: Every developer has their own way of writing code. When modules from different developers are combined, these differences can create bugs.
- Changes in Requirements: Clients often update their requirements during development. When one module is adjusted to meet new needs, it can affect how it interacts with others. Unit testing often misses these issues.
- Problems with Data Transfer: As data moves between modules, it can sometimes get lost, formatted incorrectly, or end up in the wrong place. Integration testing makes sure data flows smoothly between different parts of the system.
- Issues with APIs and Third-Party Services: Many systems rely on external APIs or tools. If there’s a mismatch in how they exchange data, it can cause errors. Use integration testing to make services work well with the rest of the software.
- Unfinished Interfaces or Databases: At the integration stage, some modules might not have fully developed interfaces or complete database connections. This can cause errors when they try to interact.
- System-Level Compatibility: Sometimes, a module isn’t fully compatible with the overall system setup. Integration testing checks that everything fits into the system as a whole.
- Missed Error Handling: Developers plan for certain errors, but they can’t predict every issue until the modules are connected. Integration testing helps find unhandled problems and improves error handling across the system.
- Hardware-Software Issues: If the software interacts with hardware, there can be problems like incompatible drivers or data not being processed correctly.
Integration Testing Approaches
Big Bang Integration
Big Bang Integration integrates all modules or components of a system and tests at once as a single integrated unit. In this approach, integration testing just begins when all individual modules are fully developed.
How It Works:
- All the modules are developed independently and then brought together at once.
- After integration, the system is tested as a whole using predefined test cases.
Advantages:
- Ideal for smaller systems with fewer modules and their interactions are simple.
- Less time is spent on planning or setting up cause all modules are integrated and tested at the same time.
Disadvantages
- It’s challenging to define the exact module or interaction causing the problem if the test fails.
- The entire integration process gets delayed if one module is not ready.
- Waiting for all modules to be ready means errors are found later, making them harder to fix.
- Minor issues in the interface or interactions might be overlooked during testing.
- For big projects with many interconnected modules, this approach often leads to more errors and complexity.
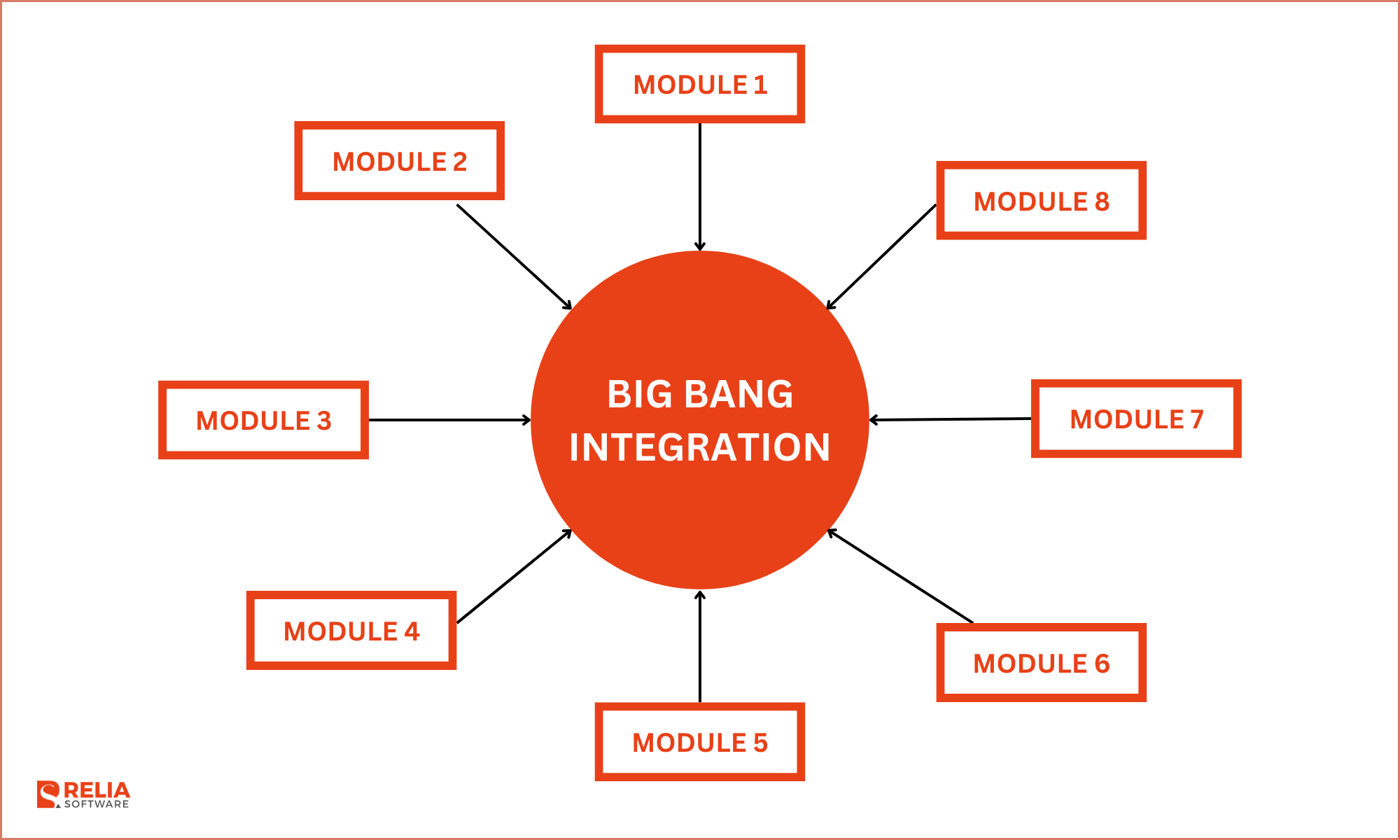
Incremental Integration
Incremental Integration is a testing approach where modules are integrated and tested in small, logical groups, rather than integrating all modules at once. Here’s how it work:
- 2 or more modules that are closely related or interdependent are grouped together.
- These grouped modules are integrated and tested to make sure they work well with each other.
- After the initial group is tested, additional modules are gradually added to the integrated group. Each time new modules are added, the entire integrated system is retested to check for errors or compatibility issues.
- This process ends when all modules in the system have been integrated and successfully tested together.
This approach uses stubs and drivers, which are temporary tools that help test modules that aren’t fully developed yet:
- Stub: Simulates a module that is called by the module being tested. It provides mock responses without implementing the full logic.
- Driver: Simulates a module that calls the module being tested, sending inputs and triggering functionality to validate its behavior.
Advantages:
- Bugs are detected early, thereby reducing the cost and effort.
- Testing in small groups is easier to isolate and resolve issues.
- Testing can begin as soon as a few modules are ready without delays by waiting for all modules to be completed.
- Ideal for complex systems with many interdependent modules.
Disadvantages:
- Additional code is required to simulate incomplete modules, leading to longer development time.
- Take longer compared to combining all modules at once.
Types of Incremental Integration:
Type |
Top-Down Integration |
Bottom-Up Integration |
Hybrid (Sandwich) Integration |
Definition |
Testing begins with the top-level (parent) modules and moves downward to the lower-level (child) modules. |
Testing starts with the lowest-level (child) modules and progresses upward to the top-level (parent) modules. |
Combines top-down and bottom-up approaches. Focus on 3 layers: Top (UI), Middle (business logic), and Bottom (database). |
How it Works |
|
|
|
Pros |
|
|
|
Cons |
|
|
|
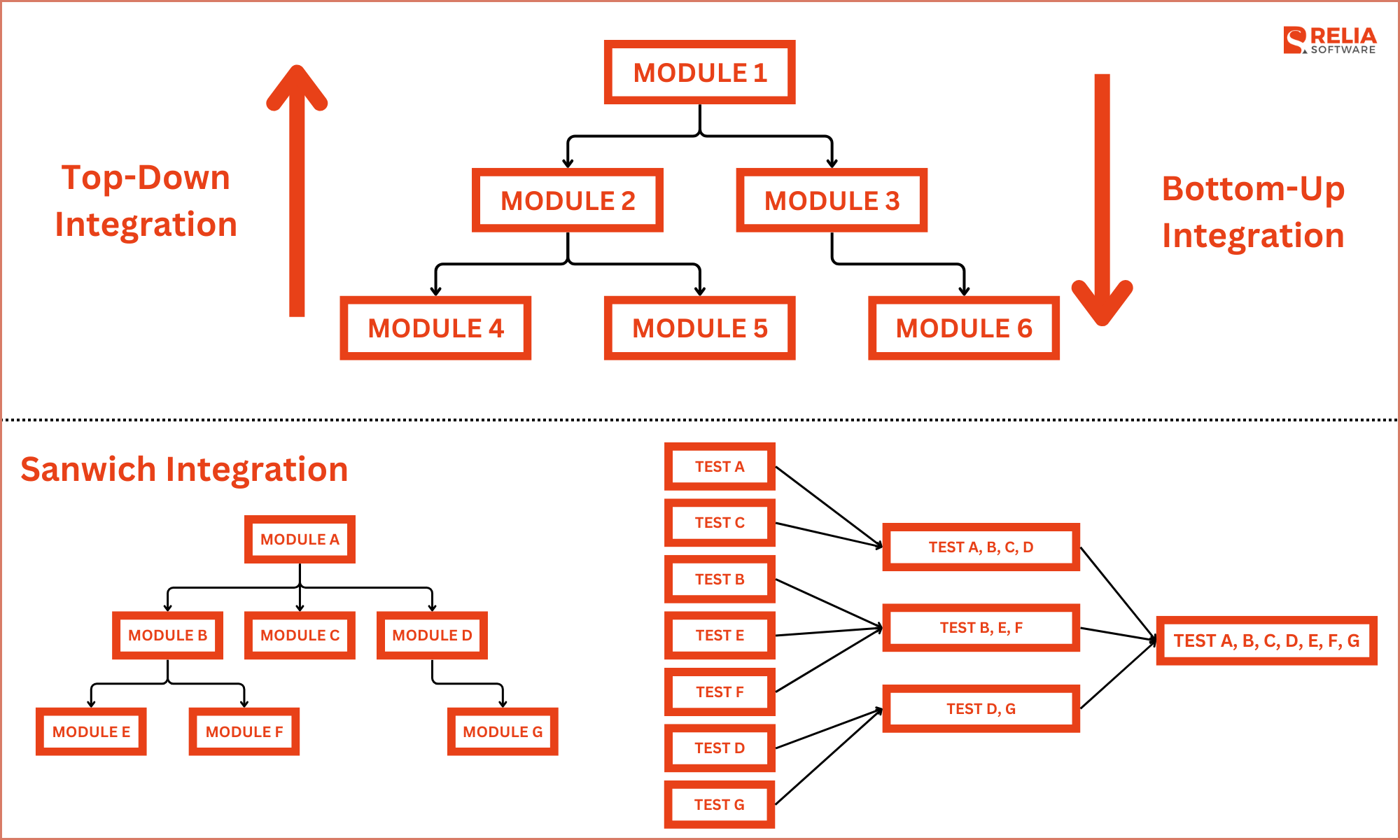
How to Implement Integration Testing?
Before implementing integration testing, make sure all the modules are unit tested. Then, follow these steps:
Review the Test Plan
- Choose testing approach (Top-Down, Bottom-Up, Hybrid, or Big Bang).
- Identify the modules to test and their dependencies.
Set Up the Testing Environment
- Prepare the tools, hardware, and software needed for testing.
- Create stubs and drivers if needed.
Create Test Scenarios and Cases
- Write test scenarios that focus on how the modules interact.
- Make detailed test cases to check data flow, error handling, and connections between modules.
- Prepare test scripts if using automated tools.
Run the Tests
- Combine and test modules step by step based on the plan.
- Record any bugs or problems found during testing.
Fix and Retest Issues
-
Report the issues to developers, have them fixed, and retest to confirm the fixes work.
Test All Scenarios
-
Keep testing all the remaining cases to ensure every module interaction is covered.
Do Final System Integration Testing
-
Test the fully integrated system to ensure all parts work smoothly together.
>> Read more:
- Detailed Explanation of 7 Software Testing Principles
- Manual Testing vs Automation Testing: Which is Better?
Conclusion
Integration testing is crucial to make all parts of a system work well together. By planning carefully, testing step-by-step, and fixing issues early, it helps create a reliable and smooth-running product. Besides, choosing an integration testing approach also saves time, reduces costs, and improves user experience because problems are all caught before the system is fully deployed.
>>> Follow and Contact Relia Software for more information!
- testing
- development