Developers and company owners use the tech stack to quickly build and deploy dynamic web apps. Over the last few years, AI, machine learning, and other data analysis and manipulation tools have made the "MEAN stack" prevalent in computing.
So, exactly what is the MEAN stack? This post will examine what MEAN stack is, its parts, and how they work together to produce smooth end-to-end programs.
What is MEAN Stack?
MEAN Stack is a JavaScript framework that creates cloud-hosted web apps. Its name is an abbreviation for the stack's four technologies including MongoDB, Express.js, Angular, and Node.js. Let's look at MEAN layer by layer.
MongoDB
Web developers utilize MongoDB. It is a NoSQL database, a document-oriented, non-relational database management system. As a document-oriented database management system, it saves data in documents. This schema-less design offers greater adaptability, allowing documents to hold diverse data structures without predefined limitations.
Example code:
const MongoClient = require('mongodb').MongoClient;
const uri = "mongodb://localhost:27017"; // Replace with your connection string
MongoClient.connect(uri, function(err, client) {
if (err) {
console.error(err);
return;
}
const db = client.db("mydatabase"); // Replace with your database name
// Insert a document
const data = { name: "Alice", age: 30 };
db.collection("users").insertOne(data, function(err, result) {
if (err) {
console.error(err);
} else {
console.log("Document inserted:", result.insertedId);
}
});
// Close the connection
client.close();
});
Express.js
Express, often simply referred to as Express, is a backend web framework built on top of Node.js. Imagine it as a toolbox for efficiently constructing web applications and APIs. Unlike a full-fledged web development framework that dictates a specific structure, Express is known for its flexibility and minimalist approach.
Example code:
const express = require('express');
const app = express();
// Define a route
app.get('/', (req, res) => {
res.send('Hello from MEAN Stack!');
});
// Start the server
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
Angular
>> Read more: Top 7 Popular Approaches for State Management in Angular
Angular.js is a platform for JavaScript apps that are used to make websites. Its components manage certain functions and user interface elements.
Data binding keeps the user interface updated with data updates. Angular's modularity and dependency injection encourages clean, testable code. Angular helps web developers construct complex and scalable web apps using a big community and ample resources.
Example code:
var app = angular.module('myApp', []);
app.controller('MyController', function($scope) {
$scope.title = 'My AngularJS App';
$scope.message = 'Hello from AngularJS!';
});
>> Read more: Next.js vs Angular: What Are The Differences?
Node.js
Node.js is an open-source platform for executing JavaScript code. They are mostly used for back-end app development. Two sorts of apps exist web apps run on browsers and mobile apps on mobile devices.
Mobile and web apps are client apps that users use. These apps need backend services to store data, send emails, and push notifications. Node.js is perfect for scalable, data-intensive, real-time apps. It supports agile development and high-scale services. PayPal uses Node.js Java and Spring.
Example code:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello from Node.js server!');
});
server.listen(3000, () => {
console.log('Server listening on port 3000');
});
>> Read more:
MEAN Stack Architecture
MEAN stack architecture runs on the hosting server as a server-side JavaScript application. Client-side applications run on users' computers and mobile devices.
These things communicate between server-side JavaScript and client-side in the Mean Stack Architecture:
-
Front-end (UI): Built with Angular/AngularJS (or alternatives), the UI handles user interactions and displays information.
-
API Calls: The front-end makes API calls to the back-end server using JavaScript.
-
Back-end (Server):
-
Express.js: Handles incoming API requests and routes them to appropriate functions.
-
Node.js: Executes JavaScript code to interact with the database (MongoDB) and process data.
-
Database (MongoDB): Stores and manages the application's data.
-
Response: The processed data is sent back from the server to the front end for display or further manipulation.
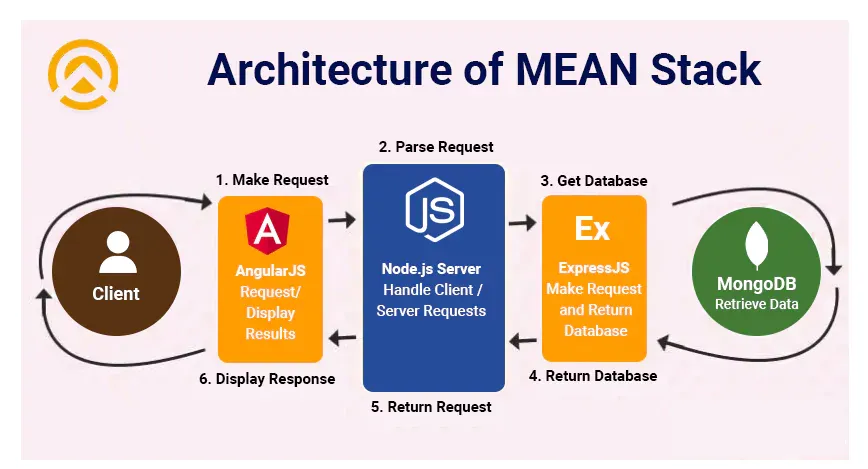
How Does MEAN Stack Work?
The MEAN stack brings together several powerful technologies to create a functional web application. Here's a breakdown of how it works in action:
Users Interaction
Imagine users interacting with a website, clicking buttons, submitting forms, or browsing through content. This initial user interaction happens entirely on the front end, typically built with a framework like Angular. This framework translates these actions into specific requests for the backend.
Backend Takes Charge
Requests will be received by Express, the central hub built on Node.js. As the maestro of the backend, Express deciphers the requests and figures out what needs to be done next.
Data Retrieval or Storage
Express might need to fetch information or store new data based on the user's request. This is where MongoDB, the NoSQL database, steps in. Express communicates with MongoDB using its drivers to access or manipulate data stored in flexible JSON-like documents.
Information Exchange
Once the data is retrieved or updated, Express prepares it for the front-end development. This data could be anything from web pages to specific details or confirmation messages. Express then sends this data back to the front end, completing the communication loop.
User Interface Updates
The front end (Angular) receives the data package from Express. Based on this data, Angular dynamically updates the application's user interface (UI). This might involve displaying new information, refreshing elements, or even a complete page reload.
Benefits of Using MEAN Stack
Open-Source
The MEAN stack uses free, open-source technology for each layer. Thus, developers can leverage public libraries and repositories. Regular stack updates are a benefit of open-source technology. Developers can also ask questions, acquire ideas, and solve problems in creating communities.
Switch Servers and Clients Easily
MEAN stack uses only JavaScript. The web applications are only in one language, so developers may easily swap between client and server. They can install web apps on a server without a stand-alone server.
Flexibility
Flexibility is a major benefit of MEAN development. One programming language (JavaScript) plus MongoDB makes the stack flexible. The server and client will run the universal code. This gives developers more flexibility to switch frameworks during development. You can cloud-host the project with MongoDB.
Additionally, developers can quickly add new features and modules during or after development. Without much hassle, you may build prototypes, customize to requirements, and scale web apps.
Cost-Friendly
The fact that MEAN is free benefits enterprises. One programming language is enough for tech-stack developers. The MEAN Stack allows companies to hire mostly JavaScript experts.
Time Savings
MEAN saves time and is ideal for busy schedules. Develop modules without starting from scratch. Instead, they can use Node.js' module library directory. You won't have to find errors. If a feature breaks, you'll be notified automatically, allowing you more time to fix it and continue your job.
MongoDB, Angular, and Node JS save data as JSON, thus no reformatting is needed. Traditional web apps require you to refresh to see server response outcomes. Single-page applications in MEAN eliminate the need to refresh web pages for server requests.
Drawbacks of MEAN Stack
Steeper Learning Curve
While JavaScript is a widely used language, mastering the entire MEAN stack requires proficiency in various frameworks and technologies. Beginners might struggle to grasp the intricacies of Angular (or alternatives), Node.js, and MongoDB simultaneously.
Limited Developer Pool
Compared to more common backend languages like Java or Python, the pool of developers with expertise in the entire MEAN stack might be smaller. This can pose a challenge for companies seeking to build and maintain MEAN stack applications.
Security Concerns
Relying solely on JavaScript requires extra attention to security measures. Since both frontend and backend logic reside in JavaScript, potential vulnerabilities in one layer could expose the entire application. Developers need to implement robust security practices to mitigate these risks.
Potential Performance Issues
While generally scalable, the performance of MEAN stack applications can be impacted by complex data structures or heavy workloads on the front-end development (Angular) or back-end one (Express). Careful optimization is crucial to ensure smooth application performance under high traffic.
Single-Language Focus
While JavaScript unification streamlines development, it can limit flexibility. If a specific project requires functionalities outside the capabilities of the MEAN stack components, developers might face challenges in integrating external libraries or frameworks.
Use Cases of MEAN Stack
Although there are some drawbacks above, the MEAN stack is still a useful development stack for some cases.
Social Media Platforms
The interactive nature and real-time updates make the MEAN stack ideal for building social media applications.
Features like user profiles, news feeds, chat functionalities, and instant updates can be efficiently implemented using the MEAN stack.
E-commerce Websites
MEAN stack can efficiently handle dynamic product listings, shopping carts, user accounts, and secure payment processing.
The ability to scale and handle large amounts of data makes it suitable for high-traffic e-commerce platforms.
>> Read more:
- Best Practices for Successful E-commerce Website Design
- Big Data E-commerce: Definitions & Best Practices
- Top 6 Vietnam Ecommerce App Development Company
Real-time Applications
Due to its asynchronous nature and ability to handle data updates in real-time, the MEAN stack is well-suited for applications like chat, collaboration tools, or live streaming services.
The real-time data exchange between the front end and back end allows for instant updates and a seamless user experience.
Single-Page Applications (SPAs)
The combination of Angular (or similar frontend framework) and Node.js in the MEAN stack makes it a popular choice for building SPAs.
SPAs load only a single HTML page and update content dynamically without full page reloads, providing a faster and more fluid user experience.
Content Management Systems (CMS)
MEAN stack can be used to build user-friendly CMS platforms where administrators can easily create, edit, and publish content.
The ability to manage user roles, permissions, and content efficiently makes it suitable for various content management needs.
Other Web Apps in Real-time
The MEAN stack is suited for real-time web apps like gaming, chat, and collaboration. Asynchronous I/O in Node.js allows real-time data processing. It also offers web sockets for client-server data transfer without HTTP requests.
Applications can send live messages and updates via this persistent connection. The permanent connection benefits a chat user when the server promptly broadcasts their message to the intended recipient.
Alternatives to MEAN Stack Framework
MERN Stack
This variation trades Angular for React, another popular JavaScript library excelling in building user interfaces. This maintains a consistent JavaScript experience throughout the development process. React's virtual DOM (Document Object Model) enhances performance and simplifies UI updates, making MERN a strong contender for building dynamic web applications.
MEVN Stack
This alternative ventures outside the realm of Angular and React, focusing on Vue.js for the front end. Vue.js offers a lightweight and approachable approach to building user interfaces, striking a good balance between flexibility and structure. While its developer community might be smaller compared to React or Angular, Vue.js presents a viable option for projects seeking an easier learning curve.
LAMP Stack
A veteran in the web development landscape, LAMP combines established technologies: Linux (operating system), Apache (webserver), MySQL (database), and PHP (server-side scripting language). This mature stack boasts extensive resources, tutorials, and a vast developer community. LAMP's suitability ranges from simple websites to complex applications, making it a dependable choice for various web development needs.
PERN Stack
This option builds upon the strengths of the MERN stack (React, Express, Node.js) by incorporating PostgreSQL, a robust relational database. PostgreSQL offers advantages like strong data integrity, complex query capabilities, and advanced features. While slightly more complex to set up and manage compared to MongoDB, PostgreSQL provides a powerful database foundation for data-driven applications.
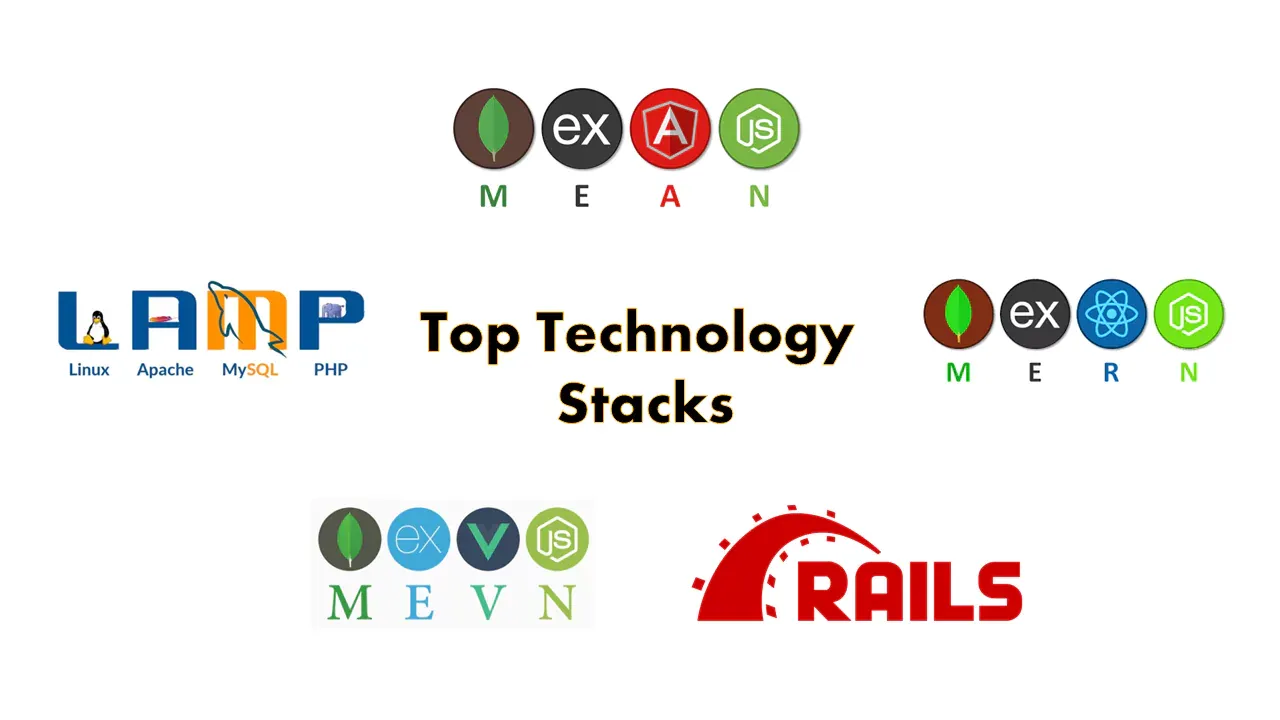
Comparison of MEAN Stack with Other Tech Stacks
Feature |
MEAN Stack |
LAMP Stack |
MERN Stack |
JAMstack |
Language(s) |
JavaScript |
PHP |
JavaScript |
JavaScript |
Database |
MongoDB |
MySQL |
MongoDB |
Various |
Full-Stack |
Yes |
Yes |
Yes |
No |
Popularity |
High |
High |
Very High |
Growing |
Learning Curve |
Moderate |
Moderate |
Moderate |
Lower |
Performance |
Good |
Good |
Good |
Excellent |
Scalability |
Good |
Good |
Good |
Good (requires planning) |
Remember, the best tech stack isn't always a one-size-fits-all solution. Carefully evaluate your project requirements, team expertise, and desired outcomes to make an informed decision.
>> Read more: MEAN vs. MERN Stack: What is the Difference?
MEAN Stack Development Case Studies
Netflix
Netflix is a popular media streaming service. Early on, they used Java on the server and JavaScript on the front. For error handling, activity tracking, and debugging, developers had to write everything twice. It was ineffective and difficult for the team to manage two programming languages.
Netflix used the MEAN stack to fix this. It lets development teams use JavaScript for front-end and back-end development. It greatly increased production. It improved the Netflix app's speed and functionality. Node.js helped the team handle high data loads. They could handle hundreds of queries per second with little latency. So, the streaming service provided a terrific user experience.
Due to enhanced user experience, user numbers skyrocketed. Netflix is the world's largest streaming service with 222 million customers.
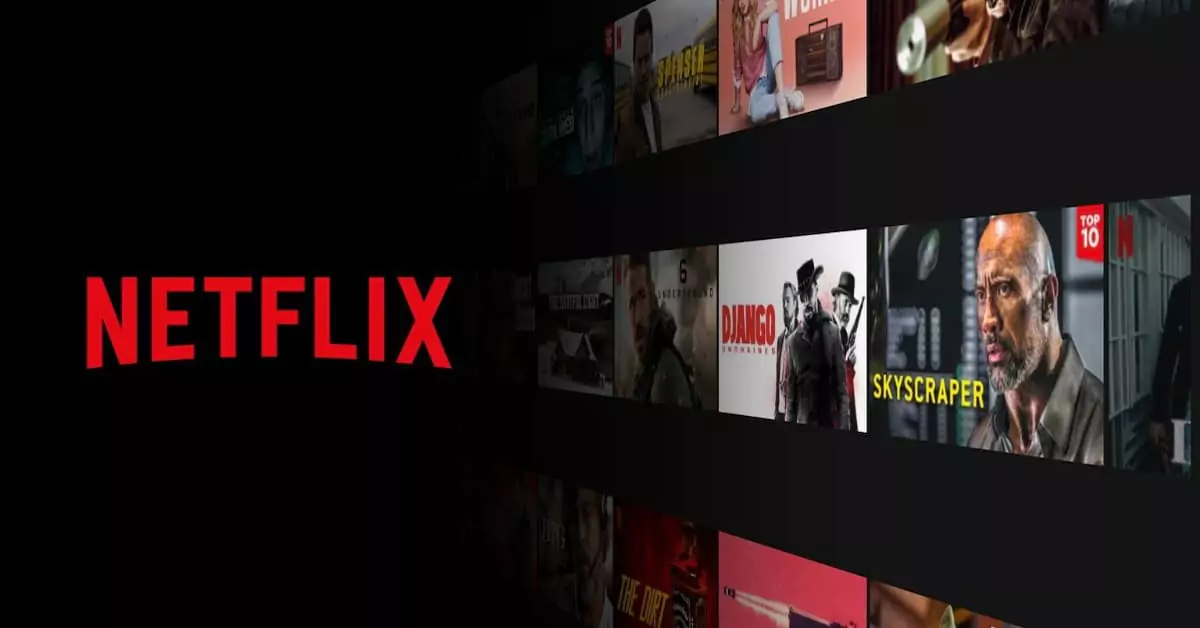
PayPal
PayPal is famous for online payments. At first, it used Java on the front-end development and JavaScript on the back. Then, the MEAN stack was used.
PayPal tried a server-side Node.js and Java prototype. A 2-person Node.js team was assigned. Five Java team members were chosen. A code sprint between the two teams saw Node.js catch up to Java engineers swiftly. The JavaScript runtime environment accelerated prototype development. Finally, the development teams chose MEAN stack technology to build the payment system.
PayPal improved performance with Node.js. Average webpage response time dropped 35%. Pages loaded 200ms faster. In addition, Angular made component styling easy. For secure transactions, they created classes and unique HTML elements using the front-end JavaScript framework.
Due to its improved performance and safe transactions, more individuals chose PayPal over other online payment options. The corporation has 325 million active accounts.
These case studies above significantly highlighted the effectiveness of the MEAN stack approach in building high-performance, scalable, and user-friendly web applications.
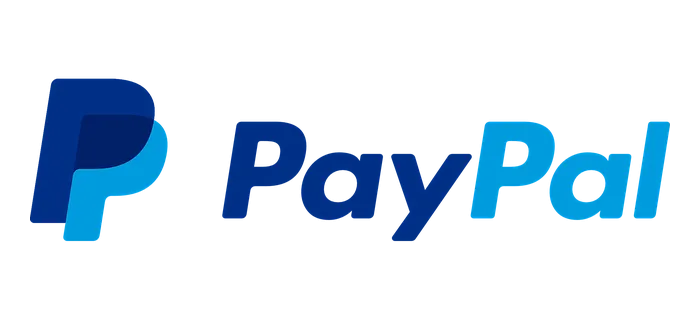
>> Read more:
- MERN Stack vs MEAN Stack: what is the difference?
-
What is Full-Stack Development? Vital Techniques & Tech Stack
-
What is .NET? A Comprehensive Guide for Developers
Conclusion
MEAN stack speeds up dynamic application development. Highly expandable and maintainable. Its layers of open-source technologies enhance performance dramatically. The MEAN stack framework improves web development workflow, saving developers time. If you're unsure which technology stack to use for dynamic web apps, consider MEAN.
Relia Software, a trustful development apps company, has solid experience in using the MEAN stack for years to create successful applicants for our customers. Do not hesitate to contact us for your application development projects!
>>> Follow and Contact Relia Software for more information!
- development