JavaScript has been a key part of web development for years, used for everything from simple websites to advanced web applications. The flexible, easy-to-learn, and widely supported features make it a top choice for developers. However, when projects get bigger, managing JavaScript code can become more difficult.
That is why TypeScript was created. TypeScript is built on JavaScript but adds static typing, better tools, and improved code organization. More businesses and developers are choosing TypeScript, leading to an ongoing debate: Which one is better for your project, TypeScript or JavaScript? This blog will explain in detail each definition, pros and cons, key differences, and best use cases for both languages.
What is TypeScript?
TypeScript is a JavaScript-based programming language that adds static typing and extra features to enhance code quality and error detection. Microsoft introduced TypeScript in 2012 to solve JavaScript problems in large projects with type-checking functions and better development tools.
The static-typed style of TypeScript enables developers to detect errors before the program execution. This language also consists of interfaces, generics, and other advanced features while supporting older JavaScript versions.
Recently, popular web development frameworks such as Angular, Node.js, or Vue have adopted TypeScript as their primary development language. Large organizations also use TypeScript to scale and maintain their open-source projects.
Pros
- Fewer Bugs: Verifies typing errors before running the code, saving time on debugging and avoiding sudden crashes.
- Improved Code Organization: Makes code easier to read and manage, especially in complex projects.
- Basic Refactoring: Allows modifying code without breaking functionality, making updates easier.
- Quality IDE Integration: Integrates with the new development tools and adds capabilities like error checking, auto-completion, and suggestions for code.
Cons
- Needs Compilation: Has to be converted into JavaScript code before running on the browser or server, making the development time longer.
- Learning Curve: Developers have to spend time getting used to TypeScript's type system, interfaces, rules, etc.
- Setup & Configuration: Requires more setups, making it overload for small projects.
- Larger Codebase: A greater number of type definitions and special syntax make the files bigger and the codebase more complicated.
>> Read more:
- Tutorial on TypeScript Decorators with Real Examples
- Using Next.js with TypeScript to Upgrade Your React Development
- Mastering 8 TypeScript Utility Types For Better Code
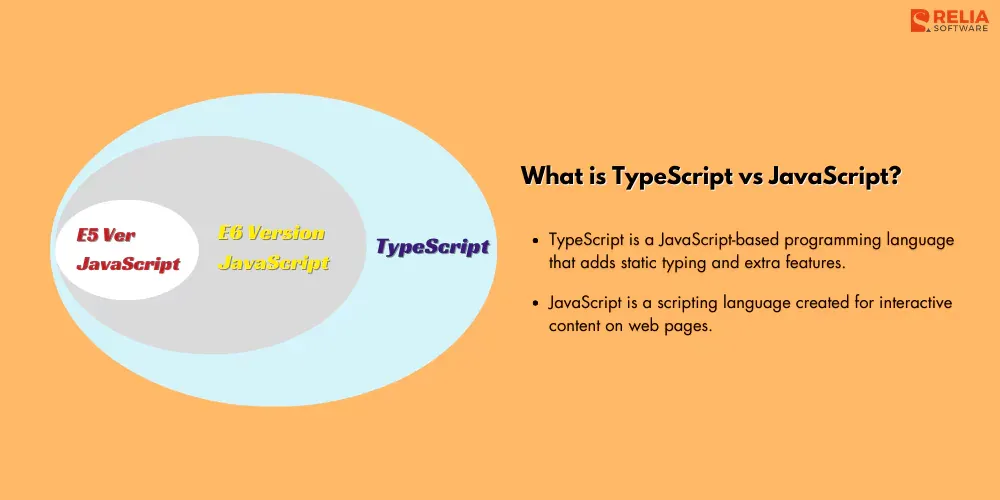
What is JavaScript?
JavaScript is a scripting language created for interactive content on web pages. The features of this language include interpretation, dynamic typing, and loose typing. Therefore, it can operate without compilation requirements and conduct type conversions automatically. This language acts as a cross-platform solution for the development of both frontend frameworks such as React and Vue, and backend frameworks like Node.js.
Beyond web development, JavaScript is also used to build mobile apps, games, and AI systems. Consequently, its flexibility makes it a top choice for developers. However, unexpected errors can appear due to its dynamic nature, so you need to carefully debug.
Pros
- Easy to Learn: The basic syntax of this language makes it an ideal choice for new programmers.
- Runs Everywhere: JavaScript can run directly in modern web browsers without setup and be used with Node.js application on servers.
- Massive Ecosystem: Many frameworks, libraries, and tools make JavaScript development faster and more efficient.
- Rapid Development: Runs instantly, allowing developers to see changes immediately through interpretation/without compiling.
Cons
- Dynamic Typing Issues: Since JavaScript doesn’t enforce types, errors can appear only at runtime, so debugging is harder.
- Security Risks: Client-side execution makes JavaScript vulnerable to XSS attacks and security threats.
- Browser Inconsistencies: Some features run inconsistently between browsers so needing additional adjustments for platform compatibility.
- Performance Limitations: Not as fast as C++ or Rust, JavaScript is less suitable for high-performance applications.
>> Read more: What Can You Do With JavaScript? 8 Popular JavaScript Usages
TypeScript vs JavaScript: What’s the Difference?
Before digging deeper into detail, let’s have an overview of the differences between TypeScript and JavaScript in the table below.
Aspects |
TypeScript |
JavaScript |
Syntax |
Static typing |
Dynamic typing |
Compilation |
Compiles to JavaScript before running |
Runs directly in browsers without compilation |
Error Handling |
Detects errors before execution |
Errors appear only at runtime |
Modern Features Support |
Supports ES6+ features, plus extras like interfaces and decorators |
Supports ES6+ features but lacks TypeScript’s type safety |
Performance |
Longer development, extra compilation stage |
Faster development cycles, due to no compilation step |
Compatibility |
Compatible with JavaScript |
Cannot run TypeScript in JavaScript files |
Tooling & Ecosystem |
Strong IDE support, better debugging, and auto-completion |
Widely supported but lacks built-in static analysis |
Learning Curve |
Requires learning type annotations and extra setup |
Easier to start with as it has fewer rules and no setup |
Setup
TypeScript cannot run directly in browsers and must be compiled into JavaScript before execution. To use TypeScript, Node.js and npm must be installed first. TypeScript is installed via npm using: npm install -g typescript
After installation, the command tsc-v can be used to check the installed version. TypeScript files (e.g., example.ts) must be compiled using tsc before they can run, generating a JavaScript file (example.js) that gets executed.
Now, let’s look at the TypeScript vs JavaScript code comparison to see how the code of TypeScript vs JavaScript differs.
First, take a look at the TypeScript setup code.
class Vehicle {
name: string;
brand: string;
constructor(name: string, brand: string) {
this.name = name;
this.brand = brand;
}
start() {
return `${this.brand} ${this.name} is running.`;
}
}
let bugatti = new Vehicle("Veyron", "Bugatti");
console.log(bugatti.start());
Running the command tsc-v example.ts typescript will compile a js file of the same name. When running, this js file will run, not the example.ts file.
Here are code examples for JavaScript:
class Vehicle {
constructor(name, brand) {
this.name = name;
this.brand = brand;
}
start() {
return this.brand + " " + this.name + " is running.";
}
}
let bugatti = new Vehicle("Veyron", "Bugatti");
console.log(bugatti.start());
Through the TypeScript vs JavaScript code example above, you can easily recognize that the advantage of Typescript over Javascript is that TypeScript code looks much cleaner and easier to read than Javascript, making it fewer bugs or errors.
Syntax
TypeScript uses static typing, meaning you must define variable types when declaring them. This requirement ensures better code reliability and easier debugging through type-related error prevention. Static typing is useful for large applications, as it keeps the code consistent and easier to manage.
let message: string = "Hello, TypeScript!";
// message = 10; // ❌ Error: Type 'number' is not assignable to type 'string'
function add(a: number, b: number): number {
return a + b;
}
// console.log(add(5, "10")); // ❌ Error: Argument of type 'string' is not assignable to parameter of type 'number'
console.log(add(5, 10)); // ✅ Output: 15
On the other hand, JavaScript operates with dynamic typing, meaning variable types are determined during program execution. This makes development faster and the code shorter. However, it may cause unpredictable bugs that are harder to catch. This is due to’s JavaScript nature that doesn’t check for type errors before execution, issues often appear only when the program runs. Thus, debugging is more difficult, especially in large projects.
let message = "Hello, JavaScript!";
message = 10; // ✅ No error, but may cause unexpected behavior later
function add(a, b) {
return a + b;
}
console.log(add(5, "10")); // ⚠️ Output: "510" instead of 15
To sum up, in the TypeScript vs JavaScript syntax differences, TypeScript’s static typing improves code reliability while JavaScript’s dynamic typing speeds up development but can lead to more runtime errors.
Compilation Process
TypeScript must be compiled into JavaScript before it can run. During this process, it checks for type errors, helping developers catch mistakes early. While this adds extra time, it makes debugging easier and more efficient since many issues are fixed before execution.
Meanwhile, JavaScript runs directly in browsers and Node.js without compilation. This makes development faster for small projects, but since errors only appear at runtime, debugging can be harder in larger codebases.
Error Handling
Through the compilation process, Typescript can analyze code to detect mistakes in the application before execution. This process helps avoid incorrect variable types, non-existent properties, and incorrect functions. Therefore, developers will spend less time repairing unexpected runtime errors and focusing more on developing the app.
Errors in JavaScript applications are only presented during the execution phase because they cannot be checked before running. This makes the debugging process more difficult, particularly in big applications.
Moreover, the flexible variable in JavaScript can also cause unexpected behaviors such as unintended type conversions and undefined values. Debuggers mostly need to conduct manual testing along with automation tools including console.log to locate and repair problems.
Modern Features Support
TypeScript improves the class-based programming feature of JavaScript by adding useful features like access modifiers, interfaces, and abstract classes. These help organize code better and make large projects easier to manage, while still supporting other JavaScript module systems. To ensure better type safety and a smoother development experience, this language also improves modern JavaScript features like async/await, optional chaining, and decorators.
>> Read more: Tutorial on TypeScript Decorators with Real Examples
JavaScript used class-based programming in ES6 but still relies on prototypes underneath. It supports ES6 modules, but older JavaScript versions still used CommonJS or global variables, which were less consistent. JavaScript also has async/await for handling asynchronous code more easily compared to callbacks and .then() chains. However, since it lacks strict type checks, it’s easier to run into unexpected issues.
>> Read more:
- Mastering Node.js Event Loop to Write Efficient Async Code
- Mastering Asynchronous Testing with React Testing Library
Performance
Although needing to compile into JavaScript before running, TypeScript does not slow down execution speed. However, the compilation step adds extra processing time, which can slightly slow down build times, especially in large projects. Despite this, TypeScript is still easier to manage complex applications and scale projects over time.
JavaScript runs immediately without compilation, making it quicker to start development. This allows for faster iteration cycles, especially in small projects where setup time matters. However, since JavaScript lacks static typing, debugging can take longer, and maintaining large applications becomes more challenging. As projects grow, JavaScript’s flexibility can lead to messy code, making scalability harder without additional tools or strict coding guidelines.
In general, there are also differences in TypeScript vs JavaScript performance. TypeScript requires compilation, adding processing time, while JavaScript runs instantly, making it faster for small projects. However, JavaScript’s flexibility speeds up coding but can cause debugging and scaling issues. TypeScript’s structured design improves maintainability and scalability but needs extra setup.
Compatibility
TypeScript is fully compatible with JavaScript since it is built on top of it. However, developers must compile TypeScript code into JavaScript before it can run. This extra step allows TypeScript to work with existing JavaScript projects while adding useful features. However, JavaScript cannot use TypeScript-specific features unless the TypeScript code is first converted into JavaScript.
In short, TypeScript can use JavaScript code, but JavaScript cannot run TypeScript without compilation.
Tooling & Ecosystem
TypeScript works well with modern development tools, making coding easier and more efficient. IDEs like Visual Studio Code provide auto-completion, real-time error checking, and better debugging, helping developers catch mistakes early. TypeScript also integrates with tools like ESLint and Prettier for cleaner, more consistent code. Many popular frameworks, including Angular and React, support TypeScript, making it a great choice for structured development.
On the other hand, JavaScript has a huge number of libraries and frameworks like React, Vue, and Node.js, making it highly flexible. It also has a massive community, so developers can easily find tutorials, support, and open-source tools. JavaScript runs directly in browsers, which makes it quick to test and deploy. However, debugging can sometimes be harder since it doesn’t have built-in type checking like TypeScript.
Learning Curve
TypeScript uses static typing for development, so developers who want to use TypeScript need to learn static typing, interfaces, and additional syntax before using it effectively. Due to the complexity of the TypeScript setup, it is harder for beginners to learn and the implementation process is longer than in JavaScript.
However, TypeScript has good documentation, official guides, and strong community support, making it easier to pick up with experience. Since TypeScript is widely used in frameworks like Angular and React, many learning resources are available for developers transitioning from JavaScript.
JavaScript is simpler for beginners because it has fewer rules and no need for type definitions. It’s widely used in web development, backend programming, and mobile apps, so there are tons of tutorials, courses, and guides available. The JavaScript community is one of the largest in the world. Developers can find support easily through forums, documentation, and open-source projects.
In short, TypeScript vs JavaScript popularity is also different. JavaScript is easier to learn, but TypeScript has a steeper learning curve, making it more challenging for newcomers. However, experienced developers can transition from JavaScript to TypeScript easily.
When to Use TypeScript?
TypeScript is ideal for developing extensive applications that need sustainable design, constant growth, and minimal errors. Projects that require long-term support also benefit from TypeScript’s structured approach, making future updates easier. Moreover, it ensures smooth work and prevents misunderstandings.
- Enterprise Applications: TypeScript remains the preferred choice of large corporations since it produces well-organized and flexible code.
- Team-Based Projects: Type-safety function promotes developer collaboration by minimizing confusing mistakes that can lead to technical bugs.
- Complex Frontend Applications: Frameworks like Angular, React, and Vue work well with TypeScript, improving code quality for front-end development.
- Backend Development: TypeScript is widely used with Node.js to build reliable APIs and server-side applications.
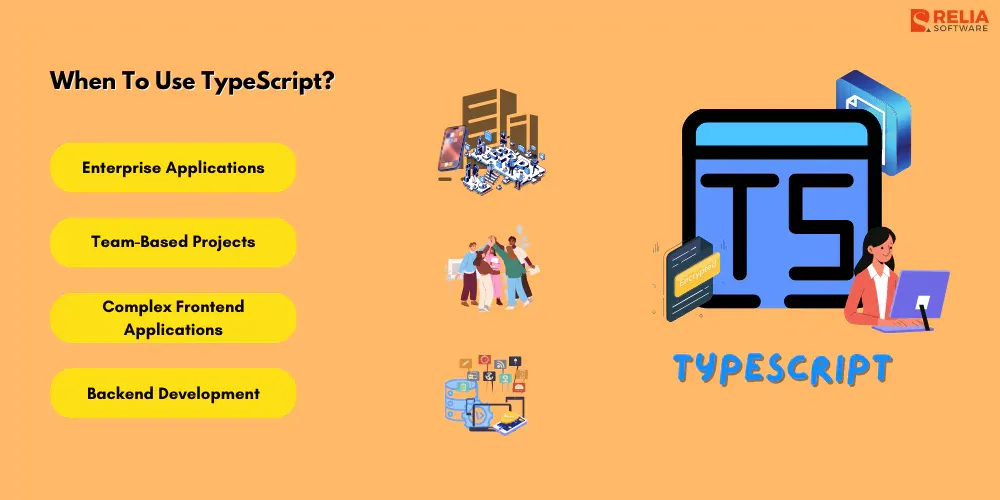
When to Use JavaScript?
JavaScript is ideal for small to medium-sized projects, where flexibility and quick development are more important than strict typing. Since it runs directly in browsers without compilation, JavaScript is perfect for fast prototyping, simple websites, and interactive web pages. It also works well for full-stack development with frameworks like Node.js.
- Simple Websites & Landing Pages: No need for extra setup, making development faster.
- Small to Medium Web Applications: JavaScript’s flexibility allows quick changes and updates.
- Real-Time Applications: Works well for chat apps, online games, and live updates.
- Full-Stack Development: Used with Node.js for both frontend and backend.
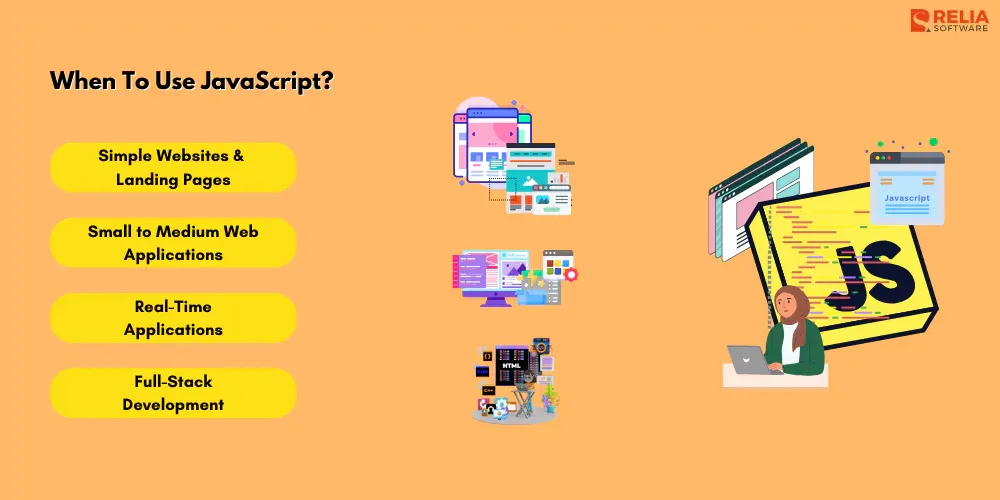
Why Do We Need TypeScript When We Already Have JavaScript?
In JavaScript, errors only appear when the code runs, which can make debugging harder and more time-consuming. TypeScript helps solve this problem by adding static typing, better tools, and improved maintainability to JavaScript.
One of TypeScript’s biggest advantages is catching errors before execution. With static typing, developers can define variable types, making the code easier to read, manage, and debug. This is especially helpful for large projects, where multiple developers work on the same codebase.
JavaScript is great for small projects and fast development, but TypeScript is better for large applications that need better organization and long-term stability. While TypeScript requires some setup and learning, it saves time in the long run by reducing bugs and keeping code more structured. It doesn’t replace JavaScript—it makes it better for developers working on complex applications.
FAQs
1. Can I use TypeScript and JavaScript together?
Yes, TypeScript and JavaScript can work together in the same project. Developers can gradually switch by renaming .js files to .ts and adding type definitions where needed. Most teams start with JavaScript and slowly implement TypeScript without rewriting all code.
2. Is TypeScript harder to learn?
If you already know JavaScript, learning TypeScript isn’t too difficult. The biggest difference is static typing, which takes some time to get used to. However, better debugging, autocomplete, and improved code structure make the learning process worth it.
3. Is TypeScript always better?
Actually not, TypeScript is great for large projects, team development, and long-term maintenance, but it's not always the best choice. For small projects, quick coding, or simple web pages, JavaScript is a better option since it runs immediately in the browser without any setup.
4. Is TypeScript too slow?
No, it isn’t. TypeScript only adds a compilation step, which doesn’t affect how fast the app runs once it’s converted to JavaScript. Although compilation takes extra time during development, it helps catch bugs early and improves code structure, making it a great choice for large projects.
5. Is JavaScript outdated?
TypeScript is becoming more popular, but JavaScript remains the foundation of the web. It powers React, Vue, Angular, Node.js, and almost all modern websites. Since TypeScript is built on JavaScript, it’s clear that JavaScript will continue to be a key technology for years to come.
>> Read more:
- Next.js vs React: How to Choose the Right Framework?
- React vs Angular: A Comprehensive Side-by-Side Comparison
- Kotlin vs Java: Which One is Right for Your Project?
Conclusion
JavaScript and TypeScript are both great languages, but they serve different purposes. JavaScript is ideal for quick development, small projects, simple websites, and real-time applications. TypeScript, on the other hand, is better suited for large, long-term projects and team-based development.
For businesses, the decision of TypeScript vs JavaScript depends on project complexity and scalability. Remember to align your project requirements and business needs for deciding which one to choose or even use both languages. Choosing the right development strategy will help ensure a smoother, more efficient development process.
>>> Follow and Contact Relia Software for more information!
- development