Asynchronous operations are a fundamental part of modern web applications. From fetching data via API calls to handling delayed updates with setTimeout
, these operations ensure that our applications are dynamic and responsive. However, testing these asynchronous behaviors can be challenging and error-prone. This article will explore techniques and best practices for mastering asynchronous testing with React Testing Library (RTL).
Asynchronous testing ensures that your application behaves correctly under various conditions. Without proper testing, async operations can lead to unexpected bugs and a frustrating user experience. React Testing Library provides powerful tools to handle asynchronous interactions, making your tests more reliable and maintainable.
>> Read more:
- Mastering React Test Renderer for Streamlined Testing
- React Suspense for Mastering Asynchronous Data Fetching
Understanding Asynchronous Testing
Asynchronous testing involves a scenario where operations don't execute immediately but are complete at some point. It's like a puzzle where you must test API calls, timers, and user interactions that trigger asynchronous state updates.
This can be tricky because it faces common challenges, including:
- Timing Issues: Ensuring that tests wait for asynchronous operations to complete before asserting.
- Flaky Tests: Tests that sometimes pass or fail due to timing inconsistencies.
- Complexity: Managing multiple async operations and their outcomes in a single test.
To better understand these concepts, let's visually represent the typical flow of asynchronous operations in React components. This diagram illustrates the sequence from triggering an async operation to handling its results and re-rendering the component. It will help clarify the steps and challenges involved in asynchronous testing.
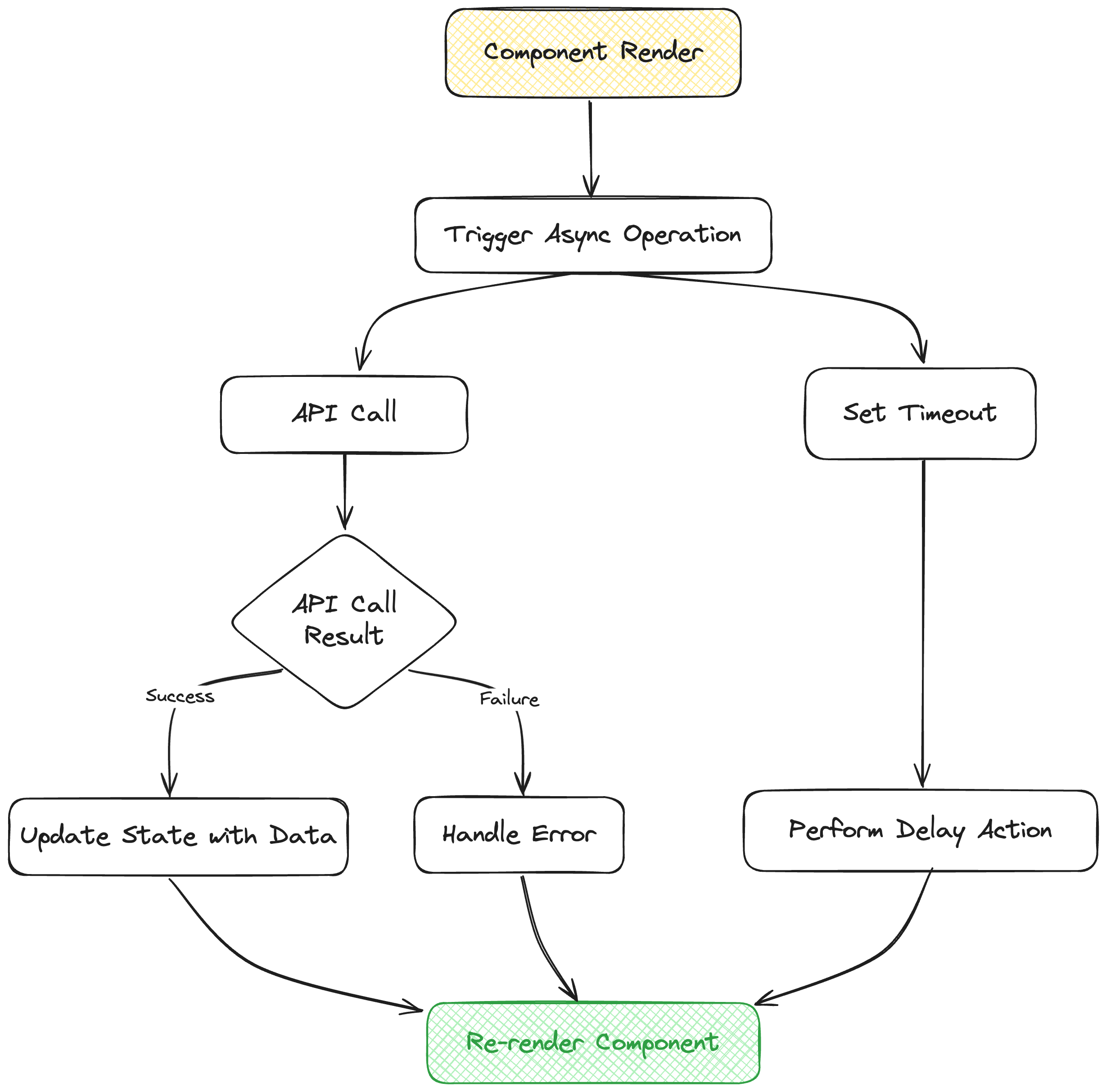
This diagram shows how various asynchronous operations interact within a React component, providing a clearer picture of the entire process. This understanding is essential as we dive deeper into the tools and techniques used to manage these operations effectively.
Key Concepts and Tools
waitFor
waitFor
is a utility provided by RTL that repeatedly calls a callback until it stops throwing an error or a timeout is reached. This is useful for waiting for async operations to complete.
import { render, screen, waitFor } from '@testing-library/react';
import '@testing-library/jest-dom/extend-expect';
import FetchComponent from './FetchComponent';
test('displays data after fetch', async () => {
render(<FetchComponent />);
// Wait for the data to be loaded and displayed
await waitFor(() => expect(screen.getByText('Data loaded')).toBeInTheDocument());
});
findBy Queries
findBy
queries are a combination of getBy
queries and waitFor
. They wait for an element to appear in the DOM and are more straightforward for common cases.
test('displays data after fetch', async () => {
render(<FetchComponent />);
// Wait for the data to be loaded and displayed
expect(await screen.findByText('Data loaded')).toBeInTheDocument();
});
Mock Service Worker (MSW)
MSW is a powerful tool for mocking API requests in tests. It provides a way to declaratively mock API responses and is highly recommended for testing async operations that involve network requests.
>> Read more: Mastering React Context API for Streamlined Data Sharing
Testing API Calls
API calls are a common source of asynchronous behavior in React applications. Properly testing these calls is crucial to ensure your components correctly handle data fetching and error scenarios. Here's how to test components that make API calls using React Testing Library (RTL) and Mock Service Worker (MSW).
Step 1: Setting Up MSW
First, install MSW to mock API requests in your tests.
npm install msw --save-dev
Next, create a server configuration file. This file defines the API endpoints and their responses, enabling you to control the behavior of your mocked server.
// src/mocks/server.js
import { setupServer } from 'msw/node';
import { rest } from 'msw';
export const server = setupServer(
rest.get('/api/data', (req, res, ctx) => {
return res(ctx.json({ message: 'Data loaded' }));
})
);
Step 2: Using MSW in Tests
To ensure that MSW is properly set up for your tests, configure it to start before all tests and reset handlers after each test. This configuration ensures a clean state for every test run.
// src/setupTests.js
import { server } from './mocks/server';
import '@testing-library/jest-dom';
beforeAll(() => server.listen());
afterEach(() => server.resetHandlers());
afterAll(() => server.close());
Step 3: Writing the Test
With MSW set up, you can now write tests for components that make API calls. Use RTL's render
and screen
utilities to verify that your component behaves as expected when fetching data.
import { render, screen } from '@testing-library/react';
import FetchComponent from './FetchComponent';
test('displays data after fetch', async () => {
render(<FetchComponent />);
// Wait for the data to be loaded and displayed
expect(await screen.findByText('Data loaded')).toBeInTheDocument();
});
To better visualize these steps, refer to the flowchart below. This diagram illustrates the process of setting up MSW and writing a test for an API call, providing a clear overview of each stage involved.
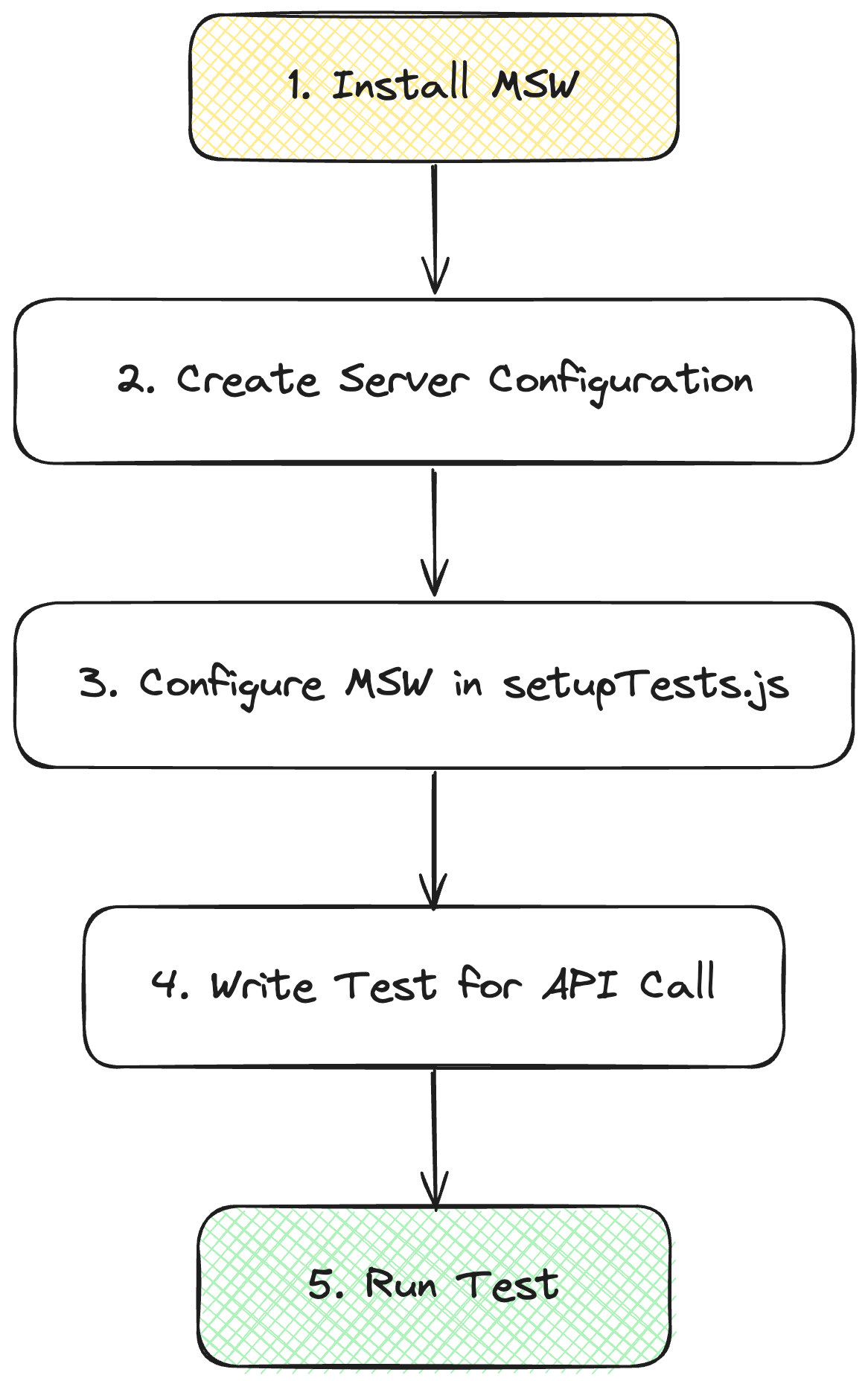
By following these steps and using the visual guide, you can effectively set up MSW and write robust tests for your React components that depend on asynchronous API calls. This ensures your components can handle various scenarios, improving the reliability and maintainability of your application.
>> Read more:
- Mastering Axios in React.js for Effective API Management
- What is tRPC? Building Robust APIs with TypeScript and tRPC
Handling Delayed Updates
Components often need to handle delayed updates, such as showing a loading spinner while waiting for data or performing an action after a timeout.
Testing setTimeout
To test delayed updates, you can use jest.useFakeTimers
to control the timing of your tests.
import { render, screen } from '@testing-library/react';
import DelayedComponent from './DelayedComponent';
test('displays message after delay', () => {
jest.useFakeTimers();
render(<DelayedComponent />);
jest.advanceTimersByTime(5000);
expect(screen.getByText('Delayed message')).toBeInTheDocument();
jest.useRealTimers();
});
Testing Loading States
Testing components that show a loading state while waiting for async operations can be done using waitFor
and findBy
queries.
import { render, screen } from '@testing-library/react';
import LoadingComponent from './LoadingComponent';
test('displays loading spinner and then data', async () => {
render(<LoadingComponent />);
// Check for the loading state
expect(screen.getByText('Loading...')).toBeInTheDocument();
// Wait for the data to be loaded and displayed
expect(await screen.findByText('Data loaded')).toBeInTheDocument();
});
Best Practices for Asynchronous Testing
Use Appropriate Queries
findBy
Queries: UsefindBy
for elements that will appear asynchronously.waitFor
: UsewaitFor
when you need more control over when to make assertions.
>> You may consider: Mastering React Query: Simplify Your React Data Flow
Avoid Flaky Tests
- Ensure Deterministic Behavior: Control async operations using tools like MSW and fake timers.
- Use Timeouts Wisely: Adjust timeouts based on the expected duration of async operations.
Keep Tests Maintainable
- Abstract Repetitive Code: Use custom render functions to encapsulate standard setups.
const customRender = (ui, options) =>
render(ui, { wrapper: MyContextProvider, ...options });
export * from '@testing-library/react';
export { customRender as render };
- Mock External Dependencies: Use MSW to mock API responses consistently.
Debugging Async Tests
- Use
screen.debug()
: Print the current state of the DOM to help diagnose issues. - Check Timing Issues: Ensure assertions are made after completing async operations.
Conclusion
Mastering asynchronous testing with React Testing Library can significantly enhance the reliability and maintainability of your tests. Understanding the common challenges and leveraging tools like waitFor
, findBy
queries, and MSW makes your testing process smoother and boosts your confidence in handling asynchronous operations effectively.
If you're ready to elevate your async testing skills, start by integrating React Testing Library and MSW into your test suite. Experimenting with waitFor
and findBy
queries will enable you to manage asynchronous operations more efficiently. Additionally, the official documentation is always available for more advanced techniques and best practices, providing a reassuring safety net.
Implement these techniques in your projects to see a noticeable improvement in your testing outcomes. Ensuring that your applications are functional, resilient, and user-friendly will significantly contribute to the success of your projects. Happy testing!
>>> Follow and Contact Relia Software for more information!
- coding
- Web application Development