As your React applications grow in complexity, performance optimization becomes critical. With an increased number of components, state changes, and prop updates, you may start noticing that certain parts of your UI are sluggish, or that some components are re-rendering more often than necessary. This is where memoization can play a pivotal role in enhancing performance.
React provides two powerful hooks, useMemo
and useCallback
, to help you implement memoization efficiently. While these hooks might appear similar, they serve different purposes, and knowing when to use each is key to writing high-performance React code. In this article, we’ll explore both hooks in depth, demonstrating how they can be leveraged to optimize advanced React applications.
>> Read more: Top 7 Best Performance Testing Tools for Developers
Understanding useMemo
useMemo
memoizes the result of an expensive computation by caching the function's output. This prevents unnecessary re-executions when the dependencies (inputs) remain unchanged, which can save significant computational resources. It's particularly useful for scenarios involving complex calculations or heavy data processing within your components.
Syntax
Here’s an advanced example of useMemo
in action:
const memoizedValue = useMemo(() => {
const result = complexCalculation(a, b);
return postProcess(result);
}, [a, b]);
In this scenario, useMemo
not only caches the result of complexCalculation
, but it also includes an additional postProcess
step that could involve further data manipulation or filtering. By wrapping both operations inside useMemo
, you ensure that neither is re-executed unless one of the dependencies (a
or b
) changes.
When to Use useMemo?
useMemo
is particularly useful for intermediate to advanced developers in scenarios like:
- Complex Data Structures: Imagine you're manipulating a deeply nested object or performing operations on a large array of data, such as filtering, sorting, or aggregating.
useMemo
can save time by avoiding repeated, costly calculations. - Optimizing Selector Functions: When using libraries like
reselect
in Redux, selectors that derive state or compute derived data from the Redux store can benefit fromuseMemo
, ensuring they only recompute when necessary. - Preventing Re-renders in Dependent Components: Components that rely on derived state or computed props can use
useMemo
to avoid redundant re-renders by memoizing these values.
Example
Here’s a practical example of useMemo
used in an advanced scenario, such as optimizing data processing in a high-frequency rendering environment:
import React, { useState, useMemo } from 'react';
const ExpensiveDataProcessing = ({ data }) => {
const processedData = useMemo(() => {
console.log('Processing data...');
return data.map(item => ({
...item,
derivedValue: computeHeavyOperation(item.value)
}));
}, [data]);
return (
<ul>
{processedData.map(item => (
<li key={item.id}>{item.derivedValue}</li>
))}
</ul>
);
};
const App = () => {
const [data, setData] = useState(generateInitialData());
return (
<div>
<ExpensiveDataProcessing data={data} />
<button onClick={() => setData(generateUpdatedData())}>Update Data</button>
</div>
);
};
export default App;
In this example, ExpensiveDataProcessing
handles a large dataset that requires heavy computation (computeHeavyOperation
) for each item in the list. Here’s how useMemo
optimizes this scenario:
- Data Processing: The
data.map
operation is expensive due to thecomputeHeavyOperation
function, which might involve intensive calculations or API calls. By memoizing this operation,useMemo
ensures that it only runs when thedata
prop changes, rather than on every render. - Optimizing Render Frequency: Without
useMemo
, any re-render ofExpensiveDataProcessing
(e.g., caused by a parent component re-render) would trigger the entire data processing step again, significantly impacting performance. By usinguseMemo
, the component only reprocesses the data when it has actually changed.
This approach is crucial in real-time or high-frequency rendering scenarios where performance is critical.
Understanding useCallback
useCallback
memoizes a function itself rather than its result. It’s particularly useful when you need to prevent unnecessary re-renders of child components that receive a function as a prop. This becomes especially important when using React.memo
to optimize performance.
Syntax
Here’s an example of advanced useCallback
usage:
const memoizedCallback = useCallback(() => {
performSideEffect(a, b);
executeAnotherTask(c);
}, [a, b, c]);
In this scenario, useCallback
memoizes a function that performs multiple operations, potentially interacting with various APIs or triggering side effects. By controlling when this function is redefined, you ensure that it’s only recreated when one of its dependencies (a
, b
, or c
) changes.
When to Use useCallback?
In advanced React development, useCallback
is particularly effective in:
- Event Handling in Complex Forms: When dealing with forms that include complex validation logic or dynamic field management, memoizing event handlers prevents re-creation of functions that might otherwise trigger unnecessary updates in child components.
- Optimizing Component Libraries: When building reusable component libraries,
useCallback
ensures that the internal handlers of components (such as button clicks or custom event hooks) remain stable, allowing users to integrate these components without worrying about performance overhead. - Managing Heavy Component Trees: In applications with deeply nested component trees where functions are passed down multiple levels,
useCallback
can prevent the propagation of unnecessary re-renders due to function references changing.
Example
Here’s a practical scenario using useCallback
to manage event handlers in a dynamic form component:
import React, { useState, useCallback } from 'react';
const DynamicForm = ({ fields, onSubmit }) => {
const [values, setValues] = useState(() => initializeValues(fields));
const handleChange = useCallback((field, value) => {
setValues(prevValues => ({
...prevValues,
[field]: validateField(field, value)
}));
}, []);
const handleSubmit = useCallback(() => {
const isValid = Object.keys(values).every(key => validateField(key, values[key]));
if (isValid) {
onSubmit(values);
}
}, [values, onSubmit]);
return (
<form onSubmit={handleSubmit}>
{fields.map(field => (
<div key={field.name}>
<label>{field.label}</label>
<input
type={field.type}
value={values[field.name]}
onChange={e => handleChange(field.name, e.target.value)}
/>
</div>
))}
<button type="submit">Submit</button>
</form>
);
};
export default DynamicForm;
In this scenario, DynamicForm
is a form component that dynamically renders fields based on a fields
prop and manages validation logic. Here’s how useCallback
is effectively utilized:
- Handling Field Changes: The
handleChange
function is memoized usinguseCallback
, ensuring that the function reference remains stable across renders. This is particularly important in dynamic forms where fields might be added or removed, as it prevents unnecessary re-renders of the entire form or individual fields. - Managing Form Submission: The
handleSubmit
function is also memoized, taking into account the current form values and ensuring that the form’s validation and submission logic is only recalculated when necessary. This optimization is critical in forms with complex validation logic or in scenarios where form state is managed across multiple components. - Preventing Re-renders: By stabilizing function references,
useCallback
minimizes the risk of causing unnecessary re-renders in child components or fields, which is essential in forms with numerous fields or complex UI interactions.
>> Read more: 7 Best React State Management Libraries for Any Project Sizes
What is the Difference Between useMemo and useCallback?
Having explored both useMemo
and useCallback
, let’s now highlight the key differences between them:
Purpose
useMemo is used to cache the return value of a function or computation, whereas useCallback keeps the function itself stable across renders.
Use Case
Use useMemo for heavy computations or expensive operations that should not be recalculated on every render, like filtering or sorting large datasets. Use useCallback when you need to memoize functions, especially those passed as props to child components to avoid unnecessary re-renders.
What They Cache
useMemo stores and reuses the output of a function if its dependencies haven’t changed. Meanwhile, useCallback keeps the function reference stable, recreating it only when its dependencies are updated.
Performance Considerations
useMemo helps reduce the frequency of expensive calculations, improving performance in components with complex data manipulations. useCallback maintains stable function references, minimizing the re-renders of child components, especially in complex component hierarchies.
Dependencies and Rerender Triggers
Both hooks rely on dependencies to determine when to recalculate or recreate. useMemo recalculates the function result when its dependencies change, while useCallback recreates the function itself based on dependency changes. If useCallback is used without dependencies (empty array), the function won’t be recreated, which may lead to stale closures if the function relies on changing state.
Parallel Example
Here’s a side-by-side comparison of how you might use useMemo
and useCallback
in more complex cases:
- Using
useMemo
:
const expensiveValue = useMemo(() => {
const processedData = deepCloneAndSort(data);
return filterDataBasedOnCriteria(processedData, criteria);
}, [data, criteria]);
In the useMemo
scenario, a deep clone and sort operation is performed on data, followed by filtering based on specific criteria. This combination of operations is computationally expensive and would slow down the application if repeated unnecessarily. By using useMemo, these operations are only re-executed when data or criteria change, significantly optimizing performance.
- Using
useCallback
:
const memoizedFunction = useCallback(() => {
const filteredItems = items.filter(item => item.isActive);
performSideEffect(filteredItems);
}, [items]);
In the useCallback
example, a function that filters active items and triggers a side effect is memoized. This ensures the function is recreated only when items
changes, avoiding unnecessary re-renders in child components depending on this function.
Let's check the comparison table below for quick reference:
Feature | useMemo | useCallback |
What it Memoizes | Return value of a function | Function itself |
Primary Use Case | Expensive calculations | Stable function references |
Performance Impact | Prevents recalculating complex values | Prevents unnecessary re-renders in child components |
Overuse Risk | Can add complexity for trivial calculations | Adds overhead if functions are simple to recreate |
Re-runs When | Dependencies change | Dependencies change |
Using Both useMemo and useCallback in a Typical React Application
In advanced React applications, you’ll find that useMemo and useCallback can be used together to optimize different aspects of your components. useMemo helps you avoid recalculating expensive values, while useCallback ensures that you’re not needlessly recreating functions. Together, they provide a powerful toolkit for minimizing unnecessary work and improving performance in high-stakes scenarios.
Case Study: Optimizing a Data-Driven ComponentHere’s an example of how you might use both hooks in a complex, data-driven application:
import React, { useState, useMemo, useCallback } from 'react';
import { fetchData, processLargeDataSet, performHeavyFiltering } from './dataUtils';
const DataAnalysisComponent = ({ parameters }) => {
const [data, setData] = useState(() => fetchData(parameters));
const processedData = useMemo(() => {
return processLargeDataSet(data);
}, [data]);
const handleFilterChange = useCallback((newFilter) => {
const filteredData = performHeavyFiltering(processedData, newFilter);
setData(filteredData);
}, [processedData]);
return (
<div>
<FilterComponent onChange={handleFilterChange} />
<DataDisplayComponent data={processedData} />
</div>
);
};
export default DataAnalysisComponent;
In this case study, DataAnalysisComponent
handles large-scale data processing and filtering, common in applications dealing with analytics, reporting, or real-time data manipulation. Here’s how useMemo
and useCallback
are applied:
- Data Processing with
useMemo
: TheprocessLargeDataSet
function is used to process the fetched data, which could involve complex operations such as aggregations, joins, or transformations. By memoizing this operation withuseMemo
, you ensure that this heavy processing is only re-executed when thedata
changes, thereby optimizing performance. - Handling Filter Changes with
useCallback
: ThehandleFilterChange
function manages the filtering of processed data based on user input or other criteria. UsinguseCallback
, this function is memoized to prevent unnecessary re-creation on every render, which is crucial when working with dynamic filters in a data-heavy application. - Minimizing Re-renders: Together,
useMemo
anduseCallback
work to minimize unnecessary computations and re-renders, ensuring that the component remains performant even as the dataset and user interactions grow in complexity.
Common Pitfalls and Best Practices
While useMemo
and useCallback
are powerful, they should be used judiciously. Overusing them can add unnecessary complexity to your code and, in some cases, even harm performance, especially in large-scale applications.
- Overuse and Misuse
One common pitfall is overusing useMemo
and useCallback
. Just because you can memoize something doesn’t always mean you should. Memoization adds an overhead—React has to track dependencies and manage the cache. If the cost of memoization outweighs the benefit (which can happen in simpler scenarios), it might actually slow down your app instead of speeding it up.
- Performance Considerations
It’s important to remember that memoization is a trade-off. You’re trading memory and computational overhead for potentially faster renders. If your component re-renders infrequently or if the calculations are cheap, memoization might not be worth it. Always profile your application to see if useMemo
or useCallback
is actually providing a performance benefit before adding them to your code.
- Example: A Scenario Where
useMemo
oruseCallback
Is Counterproductive
Consider a more advanced scenario where useMemo
or useCallback
might actually be counterproductive:
const SimpleListComponent = ({ items }) => {
return (
<ul>
{items.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
};
const App = () => {
const [items, setItems] = useState(generateItems());
return <SimpleListComponent items={items} />;
};
In this case, SimpleListComponent
simply renders a list of items. The operation of rendering these items is straightforward and does not involve any expensive calculations or reactivity that would benefit from memoization.
- Over-Optimization: Introducing
useMemo
oruseCallback
in this scenario would add unnecessary complexity. For example, memoizing theitems
array or the mapping function would likely have no tangible performance benefits and could actually slow down development by complicating the codebase. - Avoiding Premature Optimization: This scenario highlights the importance of avoiding premature optimization. Memoization should be reserved for cases where it provides a clear benefit, especially in more advanced applications where over-optimization can lead to maintainability issues.
useMemo vs useCallback: How to Choose?
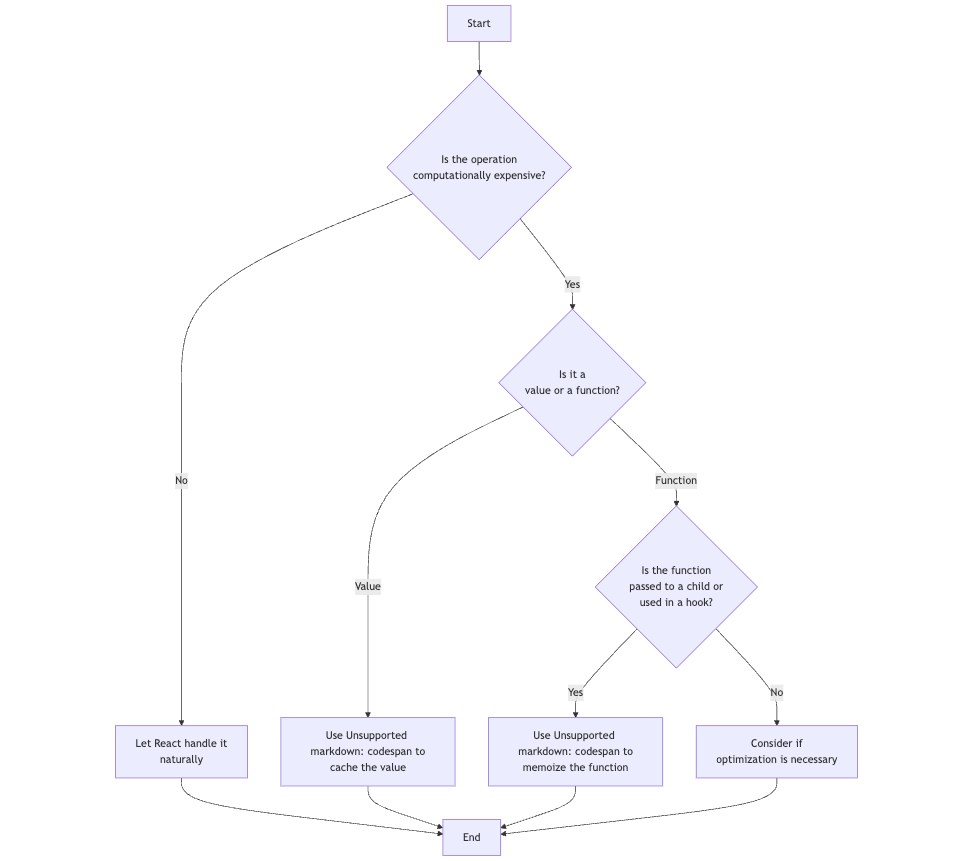
How to Use the Decision Tree?
- Is the operation computationally expensive?
- No → Let React handle it naturally without optimization.
- Yes → Proceed to the next step.
- Is the operation a value or a function?
- Value → Use
useMemo
to cache the computed value. - Function → Continue to the next question.
- Value → Use
- Is the function passed to a child component or used as a dependency in a hook?
- Yes → Use
useCallback
to memoize the function. - No → Consider if optimization is truly necessary or if it adds unnecessary complexity.
- Yes → Use
By following this streamlined decision tree, you can quickly determine when and how to use useMemo
or useCallback
, ensuring that you’re applying these hooks only where they’ll have the most impact.
FAQs
How do useMemo
and useCallback
behave differently when used with React.memo
?
useMemo
: Caches the result of expensive computations and prevents recalculations unless dependencies change, helpingReact.memo
avoid unnecessary re-renders.useCallback
: Keeps function references stable, ensuringReact.memo
doesn't re-render components due to changing function references between renders.
How do useMemo
and useCallback
behave differently when integrated into a server-side rendering (SSR) environment in React?
useMemo
: Offers little value in SSR, as components are rendered once on the server, and there are no re-renders to optimize with memoization.useCallback
: Similarly, doesn’t provide benefits in SSR since function references don’t need to remain stable across re-renders.
Are there any scenarios where useMemo
or useCallback
could interfere with lazy loading or code-splitting in React?
useMemo
: Typically no, but if memoizing values dependent on lazily loaded modules, outdated memoized values might occur if dependencies aren’t tracked correctly.useCallback
: Similar risks, where memoizing functions too early (before data is loaded) can lead to incomplete or faulty references, causing unexpected behaviors.
Are there any alternatives to useMemo
and useCallback
for optimizing React performance?
Yes, alternatives include:
React.memo
: Prevents unnecessary re-renders by memoizing the component itself.useReducer
: Efficient for complex state logic, reducing multipleuseState
calls.React.lazy
andSuspense
: Optimizes load times with code-splitting and lazy loading.useRef
: Stores mutable values without triggering re-renders.
>> Read more:
- React Suspense for Mastering Asynchronous Data Fetching
- Mastering React Server Actions for Optimizing Data Fetching
Conclusion
As we’ve seen, useMemo
and useCallback
are essential tools for optimizing performance in React. They serve different purposes: useMemo
is ideal for caching the results of expensive calculations, while useCallback
is best for maintaining stable function references. Together, they can help you write more efficient React code that avoids unnecessary re-renders and improves overall performance.
However, it’s important to use these tools judiciously. Memoization can introduce overhead and complexity, so it’s crucial to profile your application and ensure that you’re applying these hooks where they’ll have the most impact. By carefully considering when to use useMemo
and useCallback
, you can make your React applications faster and more efficient without falling into the trap of premature optimization.
>>> Contact Relia Software for more information!
- Web application Development
- development