In today's web development landscape, performance distinguishes exceptional user experiences from frustrating ones. Modern web applications must be instantaneous, responsive, and seamlessly efficient—yet traditional approaches often fall short of this standard.
Consider this:
- A mere 1-second delay can lead to a 16% drop in customer satisfaction and a 7% reduction in conversion rates.
- On mobile devices—now dominating global web traffic—every extra kilobyte of JavaScript creates noticeable lag, especially for users with slower connections or lower-powered devices.
This presents developers with an urgent, practical challenge: How do we deliver richer, more interactive web applications without compromising speed and performance?
The root cause lies in client-side JavaScript bloat. As applications grow more complex, the JavaScript sent to browsers increases exponentially. Each new library, component, and unnecessary re-render slows applications, frustrating users and hurting business outcomes.
We need a solution that optimizes this process at its core, that is React Server Components
>> Read more about React coding:
- Mastering React Higher-Order Components (HOCs)
- The Best React Design Patterns with Code Examples
- Demystifying React Redux for Centralized State Management
- Unlock the Power of GraphQL with React to Master Data Fetching
What are React Server Components?
React Server Components (RSCs) are a feature that revolutionize front-end development by enabling server-side rendering of UI components.
React Server Components (RSCs) transcend conventional optimization tools—they represent a fundamental shift in modern web application architecture. By moving rendering logic from client to server, RSCs create a leaner, faster, and more efficient architecture for React applications.
The JavaScript Complexity Trap
Traditional React applications heavily depend on client-side JavaScript for rendering, interactivity, and data fetching. This creates several critical challenges:
- Excessive Initial Load Times: Large JavaScript bundles delay the first meaningful paint of content.
- Device Resource Constraints: JavaScript parsing and execution drain battery and CPU resources, especially on mobile devices.
- Complex Data Management: Client-side data-fetching libraries like React Query or Axios add overhead and architectural complexity.
- Scalability Bottlenecks: Front-end optimization becomes increasingly challenging as applications grow.
React Server Components address these challenges directly by shifting rendering to the server and sending only lightweight HTML payloads to the client.
React Server Components Architecture
React Server Components revolutionize component rendering through three primary innovations:
Server-Side Execution
React Server Components are rendered entirely on the server. This means they never get bundled or executed in the browser. The server processes the logic and generates the output—keeping heavy lifting away from the client.
Intelligent Payload Transmission
Only the essential serialized output reaches the client, often in lightweight HTML or a streamable format instead of sending the full JavaScript logic to the browser. This significantly reduces the amount of JavaScript users need to download and parse.
Hybrid Rendering
Server and Client Components work together seamlessly, balancing rendering logic and interactivity. This allows you to render static or data-heavy content on the server while still supporting full interactivity in the browser where needed.
Server Components Vs Client Components: The following table highlights the key differences:
Feature | Server Components | Client Components |
---|---|---|
Execution | Runs on the server | Runs in the browser |
JavaScript Payload | Minimal, serialized HTML | Full JavaScript logic required |
State Management | Not supported | Fully supported (useState ,useEffect ) |
Data Fetching | Direct server-side access | Requires client-side APIs |
Performance | Faster initial load, minimal JS | More JS, slower on load |
Diagram: A visual representation of the differences:
flowchart
A[React Server Component] -->|Rendered on Server| B[Server-Side HTML]
B -->|Sent to| C[Client Browser]
C --> D[Lightweight HTML Output]
E[React Client Component] -->|Rendered in Browser| F[JavaScript Bundle]
F -->|Executed| C
style A fill:#ffcccc,stroke:#333,stroke-width:2px;
style E fill:#ccffcc,stroke:#333,stroke-width:2px;
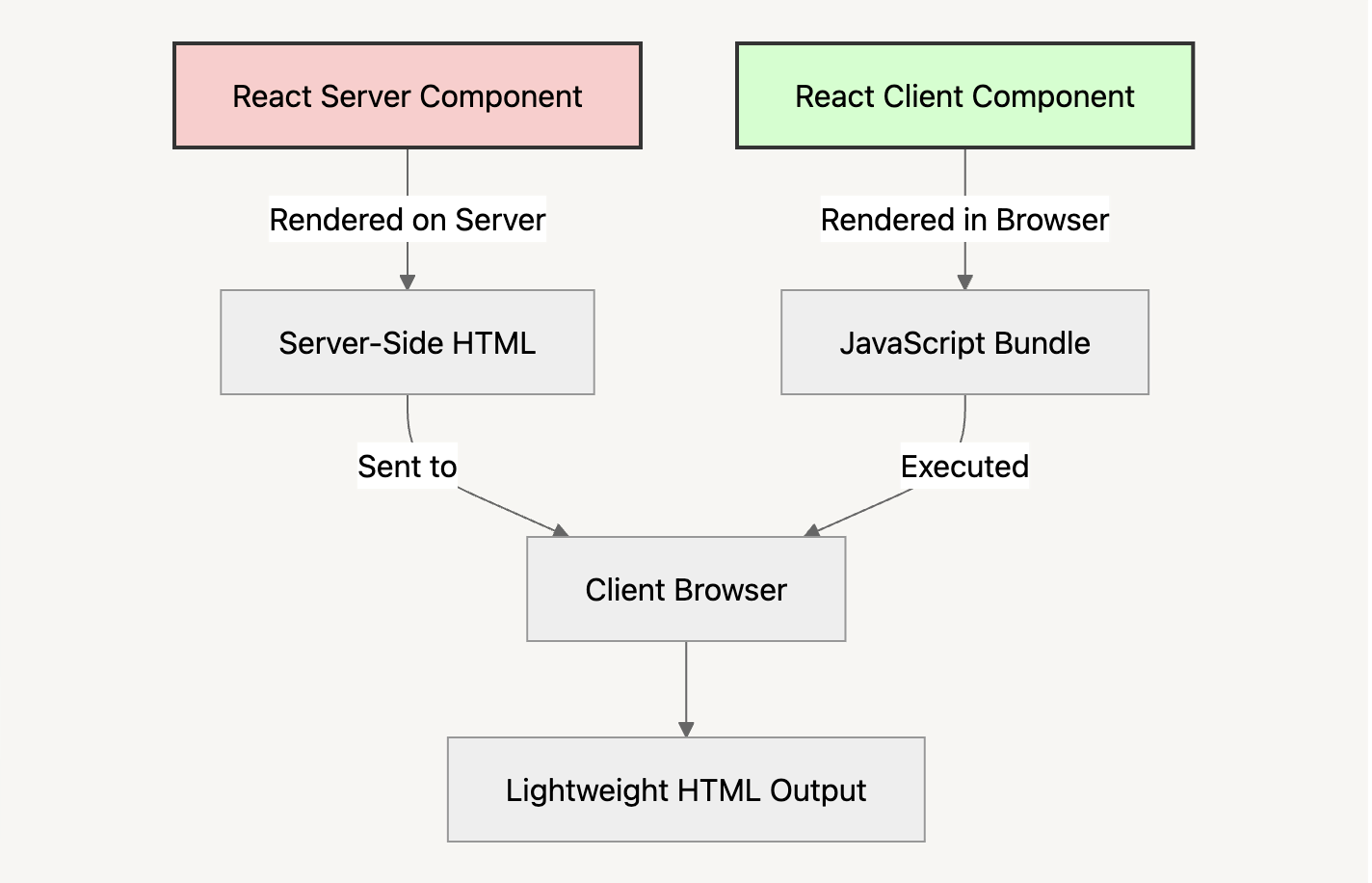
Benefits of React Server Components in Real World
Performance Optimization
-
Reduced JavaScript Payload: Since Server Components don’t run in the browser, there's no need to ship their JavaScript. This dramatically lowers the overall bundle size, resulting in faster page loads and less bandwidth usage.
-
Faster Initial Rendering: Server-rendered HTML is streamed to the browser as soon as it’s ready. This leads to instant content visibility, improving perceived performance and user experience, especially on slower networks.
-
Efficient Resource Utilization: Server-side rendering takes advantage of robust backend resources rather than relying on the limited power of the user’s device. This reduces the CPU and memory load on mobile devices and low-end machines, helping apps run more smoothly for everyone.
Simplified Data Fetching Paradigm
RSCs eliminate client-side complexities by handling data fetching on the server.
Example: Fetching Data in a Server Component
// Server Component: Fetch and Render Products
async function ProductCatalog() {
const products = await fetch('/api/products').then(res => res.json());
return (
<ul>
{products.map(product => (
<li key={product.id}>{product.name}</li>
))}
</ul>
);
}
Diagram: How data fetching works in RSCs:
sequenceDiagram
participant Server
participant Database
participant Browser
participant User
Server ->> Database: Fetch Data
Database -->> Server: Return Data
Server -->> Browser: Send Rendered HTML
Browser -->> User: Display Data (No JavaScript Execution)
User ->> Browser: Trigger Interaction
Browser ->> Server: Fetch Updated Data
Server -->> Browser: Send Updated HTML
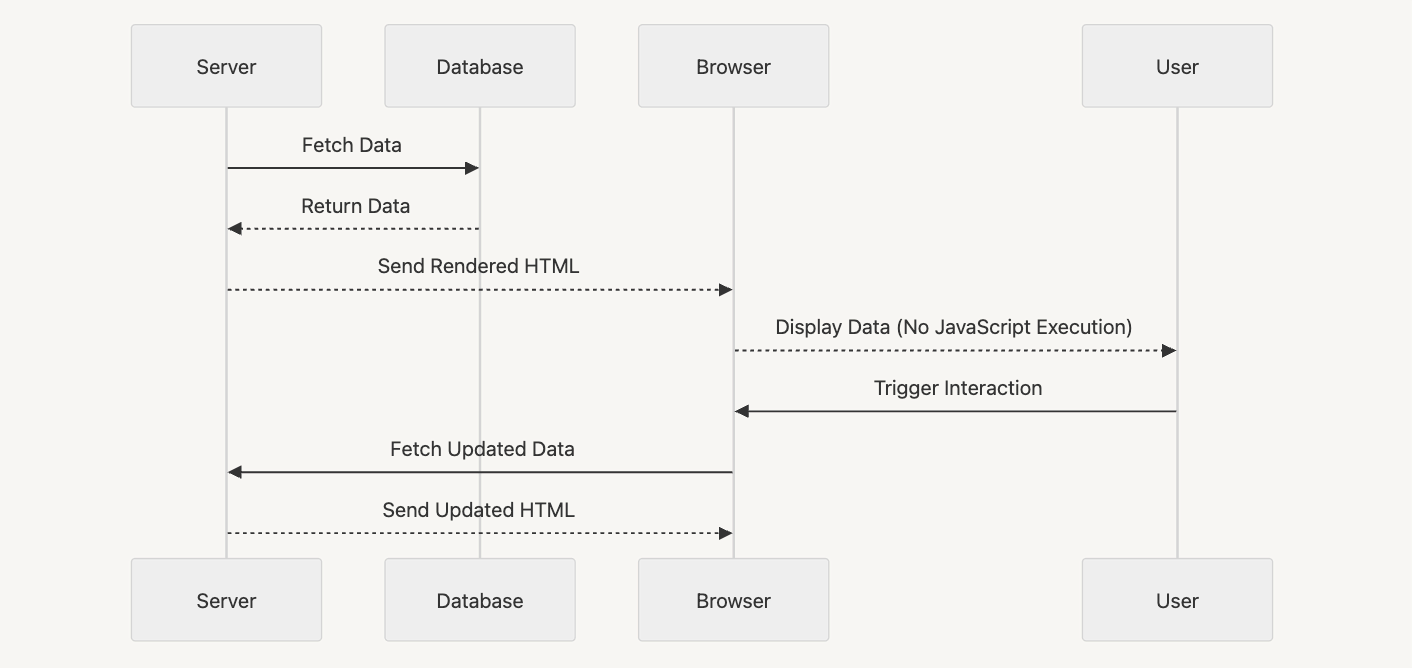
Implement Hybrid Rendering
React Server Components and Client Components complement each other perfectly in building modern, efficient web applications.
Diagram: Hybrid rendering workflow:
flowchart TB
S[Server] -->|Server Component| RSC[Server-Side Rendering]
RSC -->|HTML Streamed| Browser[Client Browser]
Browser -->|Render UI| UI[Final Web Page]
Browser -->|Interactive Features| C[Client Component]
C -->|State & Events| UI
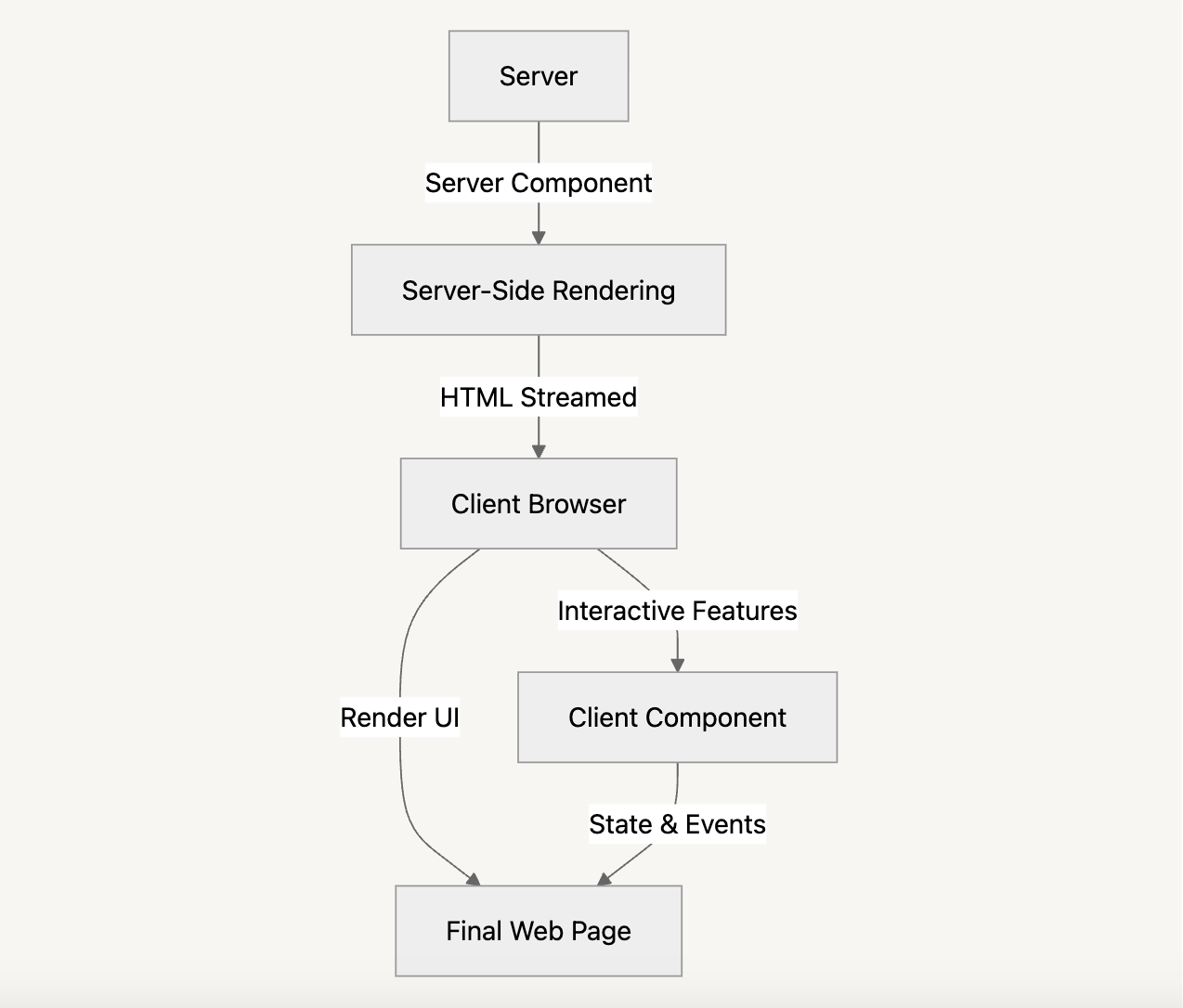
- Use Server Components for static and data-heavy content.
- Use Client Components for interactive and event-driven elements.
Using React Server Components with Next.js
Frameworks like Next.js are at the forefront of supporting RSCs. With Next.js 15 and the App Router, every component is a Server Component by default unless marked with 'use client'
.
Next.js Features Supporting RSCs:
- Streaming Support: Enables incremental rendering and partial hydration.
- Built-in Data Caching: Reduces duplicate fetches and speeds up loading.
- Enhanced Fetch API: Offers fine-grained control for revalidation and caching strategies.
- Improved Developer Experience: Better error overlays and performance tools.
This ecosystem enables developers to integrate React Server Components effortlessly, delivering immediate benefits.
// app/page.tsx
export default function Home() {
console.log('Server Component');
return (
<main>
<h2>server component</h2>
</main>
);
}
To define a Client Component:
"use client"
import { useState } from 'react';
export default function CreateButton() {
const [isLoading, setIsLoading] = useState(false);
return (
<button onClick={() => setIsLoading(true)}>
{isLoading ? 'Loading...' : 'New Post'}
</button>
);
}
>> Read more:
- Next.js: Empowering Web Development with React
- Optimize Next.js E-commerce Sites for Better SEO and Performance
Strategic Best Practices for Using RSCs
Adopt a Hybrid Approach
- Use Server Components for static and data-driven UI.
- Use Client Components for interactive elements.
Profile and Optimize
- Use performance profiling tools to identify bottlenecks and fine-tune your components.
Incremental Adoption
- Migrate parts of your application gradually to Server Components.
>> You may be interested in:
- Top 6 Best React Component Libraries for Your Projects
- Next.js vs React: How to Choose the Right Framework?
- Tailwind CSS for React UI Components: Practical Code Examples
- In-Depth Tutorial for Mastering React Error Boundaries
Conclusion
React Server Components represent more than incremental improvement—they mark a revolution in web development architecture. Through server-side execution, reduced JavaScript payloads, and streamlined data-fetching strategies, RSCs enable developers to build applications that are faster, leaner, and more scalable.
With frameworks like Next.js leading the charge, React Server Components are becoming essential tools for delivering next-generation digital experiences.
The web development landscape is evolving. Are you ready to embrace the server-first future?
>>> Follow and Contact Relia Software for more information!
- development