The heart of any dynamic Flutter app lies in its state management. But with an array of approaches available, from built-in Stateful Widgets to Provider, Riverpod, GetX, MobX, Bloc, and Redux, choosing the right one can feel overwhelming.
This guide simplifies your decision-making by demystifying state management in Flutter and offering a comprehensive comparison of popular options. We'll explore their strengths, weaknesses, and ideal use cases, empowering you to confidently select the solution that best aligns with your project's complexity and your team's preferences.
>> Read more:
- Is Flutter Good For Web Development? Benefits & Drawbacks
- Flutter or React Native: What to Choose?
- The In-depth Guide for Mastering Navigation in Flutter
- Step-by-Step Tutorial to Master Flutter TextField Validation
What is State Management in Flutter?
In Flutter applications, state management refers to the practice of handling data that dictates the visual appearance and behavior of the user interface (UI). This data can change dynamically based on user interactions or external events. Effective state management is crucial for building dynamic UIs because it ensures the UI reflects the current state of the application accurately.
Consider a shopping cart app - the number of items displayed (state) should update as users add or remove products. Without proper state management, the UI might not reflect these changes, leading to a confusing and unresponsive user experience.
7 Popular Approaches for State Management in Flutter
Stateful Widgets
Flutter offers built-in Stateful Widgets as the fundamental building block for handling state within your application. These widgets are like containers that hold data (state) and a build method that determines the UI based on that data.
Here's a breakdown of Stateful Widgets in Flutter:
- Stateful Widget Class: This class inherits from the StatefulWidget base class, acting as the blueprint for your stateful widget.
- State Object: Each Stateful Widget instance creates a corresponding state object, responsible for holding and managing the state data. This object inherits from the State base class.
- build() Method: The build() method defines the UI of the widget. This method takes a BuildContext argument and returns a widget tree representing the UI. Importantly, the build() method can access and utilize the state data from the state object.
- setState() Method: The setState() method, available within the state object, allows you to update the state data. When you call setState(), the framework automatically rebuilds the widget tree, ensuring the UI reflects the latest state.
Code example:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: CounterPage(),
);
}
}
class CounterPage extends StatefulWidget {
@override
_CounterPageState createState() => _CounterPageState();
}
class _CounterPageState extends State<CounterPage> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Counter App'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.headline4,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
Built-in Stateful Widgets provide a solid foundation for state management in Flutter, particularly for simpler applications. However, for more complex scenarios with extensive data flow and UI updates, other state management approaches like Provider, Riverpod, or Bloc might be more suitable.
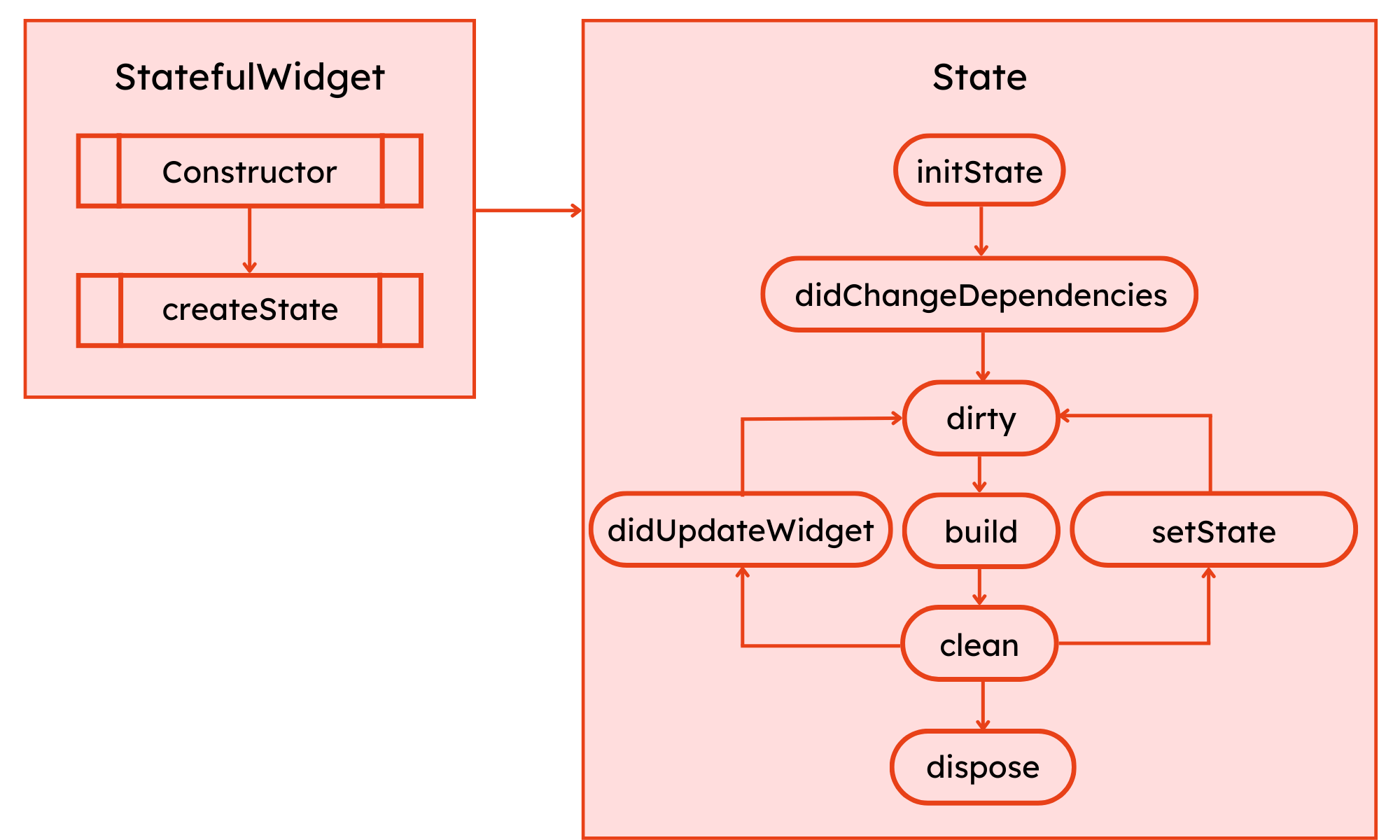
Provider
While built-in Stateful Widgets offer a basic approach to state management, Provider emerges as a popular option for projects requiring more organization and scalability. Provider acts as a dependency injection solution, streamlining how data (state) is accessed and shared across different parts of your Flutter application.
Here's a breakdown of Provider:
- Provider Package: This external package is integrated into your Flutter project to leverage Provider's functionalities.
- State Providers: These are classes or objects that hold and manage the state data you want to share with various widgets.
- Consumers: Widgets that depend on a particular state data act as consumers. They utilize the Provider.of method to access and utilize the state provided by a Provider.
- MultiProvider: For applications with multiple state providers, MultiProvider simplifies managing and providing them together.
Code example:
- Add
provider
to yourpubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
provider: ^6.0.0
- Create a
Counter
class for the state:
import 'package:flutter/foundation.dart';
class Counter with ChangeNotifier {
int _count = 0;
int get count => _count;
void increment() {
_count++;
notifyListeners();
}
}
- Update the
main.dart
file:
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
import 'counter.dart'; // Ensure this path is correct based on your project structure
void main() {
runApp(
ChangeNotifierProvider(
create: (context) => Counter(),
child: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: CounterPage(),
);
}
}
class CounterPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
final counter = Provider.of<Counter>(context);
return Scaffold(
appBar: AppBar(
title: Text('Counter App with Provider'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Text(
'${counter.count}',
style: Theme.of(context).textTheme.headline4,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: counter.increment,
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
Provider has advantages like:
- Flexibility: State can be accessed from anywhere in the widget tree.
- Testability: State providers are easily isolated and tested.
- Ease of Use: Provider offers a relatively straightforward approach compared to more complex state management solutions.
However, Provider can introduce some boilerplate code for complex state management scenarios. Other options like Riverpod address this by offering a more concise syntax.
Riverpod
Riverpod is considered as the next evolution of Provider in the realm of Flutter state management. Built upon the foundation of Provider, Riverpod streamlines the process with a focus on readability and reduced boilerplate code.
Here's a breakdown of Riverpod:
- Riverpod Package: Similar to Provider, Riverpod is an external package you integrate into your Flutter project.
- State Providers: Just like in Provider, state providers hold and manage the data you want to share with various widgets.
- Consumers: Widgets that rely on a specific state act as consumers. They utilize the Provider.of method (inherited from Provider) to access the state provided by a Riverpod provider.
- Automatic Dependency Resolution: Riverpod offers a significant advantage with automatic dependency resolution. This means you don't need to explicitly pass providers down the widget tree, simplifying the code structure.
Code example:
- Add
flutter_riverpod
to yourpubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
flutter_riverpod: ^2.0.0
- Create a
counter_provider.dart
file:
import 'package:flutter_riverpod/flutter_riverpod.dart';
final counterProvider = StateProvider<int>((ref) {
return 0;
});
- Update the
main.dart
file:
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
import 'counter_provider.dart'; // Ensure this path is correct based on your project structure
void main() {
runApp(
ProviderScope(
child: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: CounterPage(),
);
}
}
class CounterPage extends ConsumerWidget {
@override
Widget build(BuildContext context, WidgetRef ref) {
final counter = ref.watch(counterProvider);
return Scaffold(
appBar: AppBar(
title: Text('Counter App with Riverpod'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Text(
'$counter',
style: Theme.of(context).textTheme.headline4,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: () => ref.read(counterProvider.notifier).state++,
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
Riverpod offers benefits like:
- Concise Syntax: Less boilerplate code compared to Provider, making code cleaner and easier to maintain.
- Automatic Dependency Resolution: Simplifies managing dependencies within your widget tree.
- Familiarity: If you're already familiar with Provider, the transition to Riverpod is relatively smooth.
However, Riverpod is a relatively new solution compared to Provider, and its community and ecosystem might still be under development.
GetX
GetX is an ideal choice for projects seeking a balance between simplicity and performance. It offers a lightweight and efficient approach to state management, streamlining data handling within your Flutter application.
Here's a breakdown of GetX:
- GetX Package: This external package integrates with your Flutter project, providing functionalities for state management and beyond (routing, dependency injection).
- Observables (Observables & GetXController): These are core concepts in GetX. Observables are data sources that can notify dependent widgets whenever their value changes. GetXController acts as a container for holding your application state and associated logic (similar to BLoC or Provider).
- GetBuilder & Obx: These widgets are used to listen to changes in observables and rebuild the UI accordingly. GetBuilder offers a more traditional approach, while Obx leverages Flutter's reactivity system for more efficient updates.
- Dependency Injection: GetX also provides a basic dependency injection system, allowing you to manage dependencies within your GetXControllers and throughout your application.
Code example:
- Add get to your
pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
get: ^4.6.1
- Create a
counter_controller.dart
file:
import 'package:get/get.dart';
class CounterController extends GetxController {
var count = 0.obs;
void increment() {
count++;
}
}
- Update the
main.dart
file:
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'counter_controller.dart'; // Ensure this path is correct based on your project structure
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GetMaterialApp(
home: CounterPage(),
);
}
}
class CounterPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
final CounterController counterController = Get.put(CounterController());
return Scaffold(
appBar: AppBar(
title: Text('Counter App with GetX'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Obx(() => Text(
'${counterController.count}',
style: Theme.of(context).textTheme.headline4,
)),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: counterController.increment,
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
Benefits of GetX:
- Performance: GetX is known for its lightweight design and efficient updates.
- Ease of Use: GetX offers a simpler syntax compared to some other state management solutions.
- Versatility: GetX goes beyond state management, providing functionalities like routing and dependency injection.
However, GetX might have a slightly steeper learning curve compared to Provider, and its approach to state management might not be suitable for all project types. Additionally, the debugging and testing experience might be less mature compared to established solutions like Bloc or Redux.
MobX
MobX is commonly used for intermediate and complex projects. It offers a compelling alternative with its reactive approach and efficient handling of state changes.
Here's a breakdown of MobX:
- Reactive Programming: MobX leverages reactive programming principles. This means your UI automatically updates whenever the underlying data (state) changes.
- Observables: These are the core concept in MobX, acting as containers for your application state, similar to variables. However, changes made to observables automatically trigger updates in widgets that depend on them.
- Actions: These functions modify the state within your observables. MobX ensures actions are executed in a predictable order, preventing unexpected behavior due to concurrent state changes.
- Reactions: These functions automatically run whenever an observable they depend on changes. This allows your UI to react to state updates without manually checking for changes.
Code example:
- Add
flutter_mobx
andmobx
to yourpubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
mobx: ^2.0.4
flutter_mobx: ^2.0.2
dev_dependencies:
build_runner: ^2.1.7
mobx_codegen: ^2.0.4
- Create a
counter.dart
file:
import 'package:mobx/mobx.dart';
// Include generated file
part 'counter.g.dart';
// This is the class used by rest of your codebase
class Counter = _Counter with _$Counter;
// The store-class
abstract class _Counter with Store {
@observable
int count = 0;
@action
void increment() {
count++;
}
}
- Run the build runner to generate the necessary code:
flutter pub run build_runner build
- Update the
main.dart
file:
import 'package:flutter/material.dart';
import 'package:flutter_mobx/flutter_mobx.dart';
import 'counter.dart'; // Ensure this path is correct based on your project structure
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: CounterPage(),
);
}
}
class CounterPage extends StatelessWidget {
final Counter counter = Counter();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Counter App with MobX'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Observer(
builder: (_) => Text(
'${counter.count}',
style: Theme.of(context).textTheme.headline4,
),
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: counter.increment,
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
Benefits of MobX:
- Improved Developer Experience: The reactive approach simplifies state management, reducing boilerplate code and making UI updates more intuitive.
- Testability: Observables and actions are easily isolated for testing, ensuring the reliability of your state management logic.
- Scalability: MobX can handle complex state management scenarios effectively.
However, MobX might have a slightly steeper learning curve compared to Provider and GetX, especially for basic applications. Developers unfamiliar with reactive programming concepts may face challenges.
Bloc
Bloc is suitable for projects requiring a more structured and predictable approach to state management. It adheres to a unidirectional data flow architecture, ensuring clear separation between presentation logic (UI) and business logic (data handling).
Here's a breakdown of Bloc:
- Bloc Pattern: This architectural pattern defines a clear separation between UI, business logic, and state management.
- Bloc Components:
- Events: These are user interactions or system events that trigger state changes.
- States: These represent the current state of your application data.
- Bloc: This component acts as a mediator, processing events and emitting new states based on the current state and business logic.
- Streams: Events and states are represented as streams, enabling asynchronous data handling and efficient updates.
Code example:
- Add
flutter_bloc
andbloc
to yourpubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
flutter_bloc: ^8.1.0
bloc: ^8.0.3
- Create a
counter_cubit.dart
file:
import 'package:bloc/bloc.dart';
class CounterCubit extends Cubit<int> {
CounterCubit() : super(0);
void increment() => emit(state + 1);
}
- Update the
main.dart
file:
import 'package:flutter/material.dart';
import 'package:flutter_bloc/flutter_bloc.dart';
import 'counter_cubit.dart'; // Ensure this path is correct based on your project structure
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: BlocProvider(
create: (_) => CounterCubit(),
child: CounterPage(),
),
);
}
}
class CounterPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Counter App with Bloc'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
BlocBuilder<CounterCubit, int>(
builder: (context, count) {
return Text(
'$count',
style: Theme.of(context).textTheme.headline4,
);
},
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: () => context.read<CounterCubit>().increment(),
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
Benefits of Bloc:
- Testability: Bloc components (events, states, Bloc) are easily isolated and tested.
- Scalability: The unidirectional data flow promotes clean architecture Flutter and simplifies handling complex state logic.
- Predictability: Bloc ensures clear separation of concerns, leading to more predictable state management.
However, Bloc might have a steeper learning curve compared to other solutions. Additionally, setting up Bloc can involve more boilerplate code for basic scenarios.
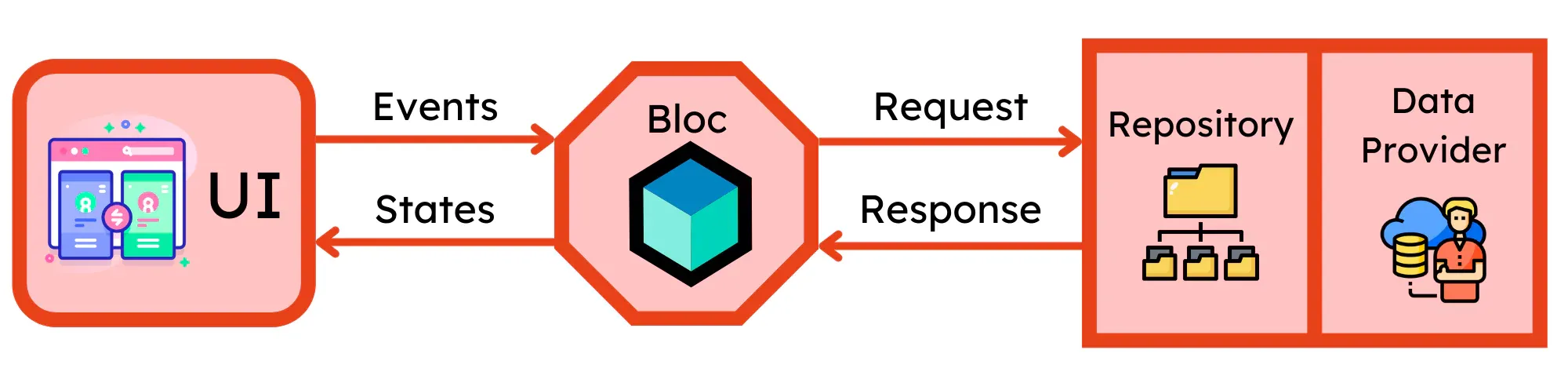
Redux
As your Flutter projects scale in complexity, managing application state across various screens and components becomes increasingly challenging. Redux steps in as a powerful solution for centralized state management, offering a predictable and unidirectional data flow.
Here's a breakdown of Redux:
- Centralized Store: Redux utilizes a single source of truth for your application state. This store holds all the data that influences the UI.
- Actions: These are plain JavaScript objects that describe what happened in the application. They represent events or user interactions.
- Reducers: These are pure functions that take the current state and an action as arguments. Reducers return the new state based on the action type and the current state.
- Dispatch: When an event occurs, you dispatch an action to the store. The store then uses the corresponding reducer to update the state.
Code example:
- Add
flutter_redux
andredux
to yourpubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
flutter_redux: ^0.8.2
redux: ^5.0.0
- Create a
counter_state.dart
file:
class CounterState {
final int count;
CounterState(this.count);
}
- Create a
counter_actions.dart
file:
class IncrementAction {}
- Create a
counter_reducer.dart
file:
import 'counter_state.dart';
import 'counter_actions.dart';
CounterState counterReducer(CounterState state, dynamic action) {
if (action is IncrementAction) {
return CounterState(state.count + 1);
}
return state;
}
- Update the
main.dart
file:
import 'package:flutter/material.dart';
import 'package:flutter_redux/flutter_redux.dart';
import 'package:redux/redux.dart';
import 'counter_state.dart';
import 'counter_actions.dart';
import 'counter_reducer.dart';
void main() {
final store = Store<CounterState>(
counterReducer,
initialState: CounterState(0),
);
runApp(MyApp(store: store));
}
class MyApp extends StatelessWidget {
final Store<CounterState> store;
MyApp({required this.store});
@override
Widget build(BuildContext context) {
return StoreProvider<CounterState>(
store: store,
child: MaterialApp(
home: CounterPage(),
),
);
}
}
class CounterPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Counter App with Redux'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
StoreConnector<CounterState, int>(
converter: (store) => store.state.count,
builder: (context, count) {
return Text(
'$count',
style: Theme.of(context).textTheme.headline4,
);
},
),
],
),
),
floatingActionButton: StoreConnector<CounterState, VoidCallback>(
converter: (store) {
return () => store.dispatch(IncrementAction());
},
builder: (context, callback) {
return FloatingActionButton(
onPressed: callback,
tooltip: 'Increment',
child: Icon(Icons.add),
);
},
),
);
}
}
Benefits of Redux:
- Predictability: The unidirectional data flow ensures predictable state changes.
- Testability: Actions and reducers are pure functions, making them easy to test in isolation.
- Debuggability: Redux offers developer tools for inspecting state changes and identifying potential issues.
However, Redux might introduce some complexity for smaller projects. Setting up the store, actions, and reducers can require more initial effort compared to simpler solutions. Additionally, managing complex state logic within reducers can become challenging for very intricate applications.
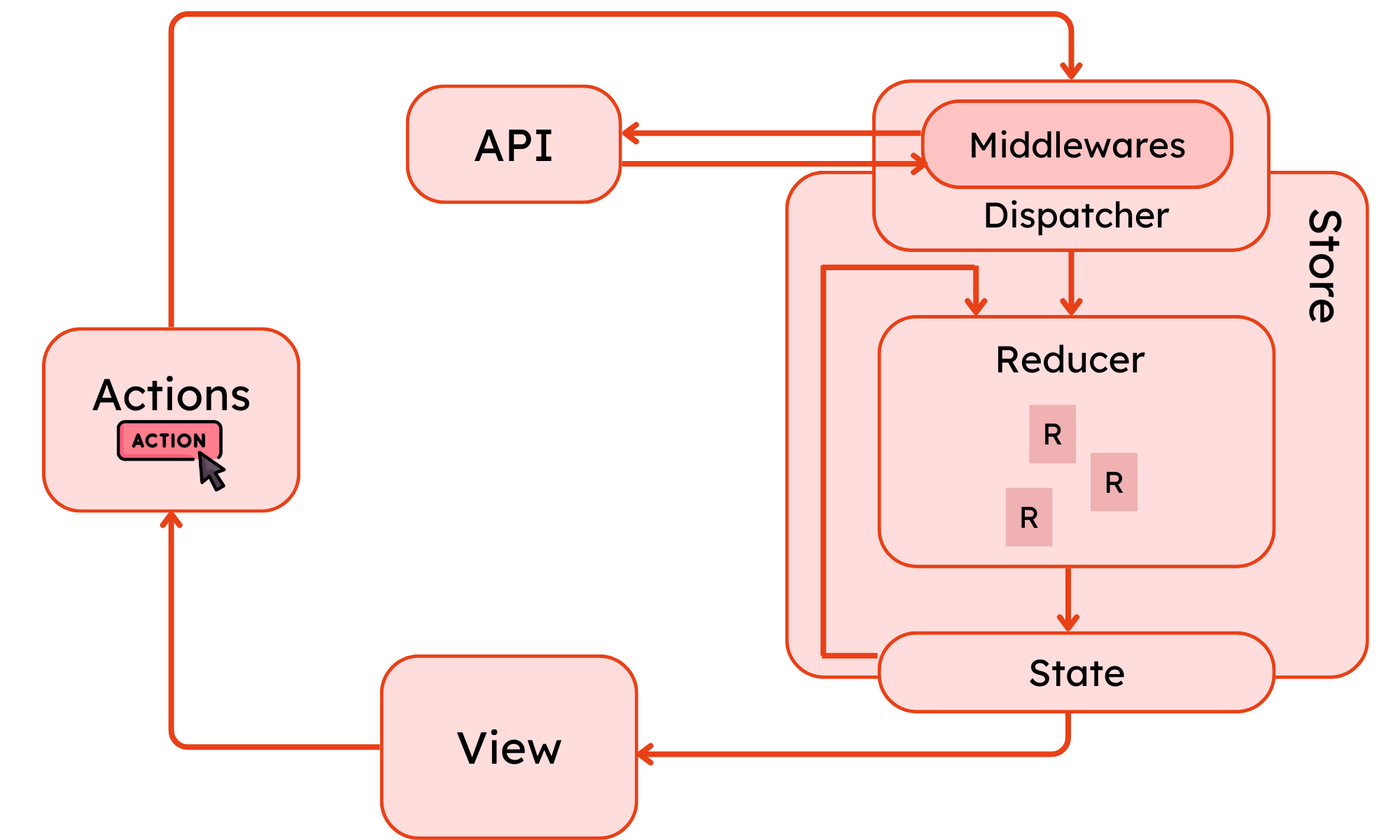
Here's a comparison table summarizing the 7 common approaches for state management in Flutter:
Approach |
Complexity |
Learning Curve |
Data Flow |
Key Strengths |
Use Cases |
Stateful Widgets |
Basic |
Lowest |
Manual |
Simplest solution for managing state within a widget or a small group of widgets. |
Small, isolated UI components with minimal state management |
Provider |
Basic - Intermediate |
Moderate |
Manual |
Good balance between ease of use and flexibility. |
Projects with moderate state management requirements. |
Riverpod |
Basic - Intermediate |
Moderate |
Manual |
Similar to Provider, potentially simpler syntax. |
Intermediate projects seeking potential boilerplate reduction. |
GetX |
Intermediate |
Moderate |
Automatic |
Lightweight, efficient, offers routing and dependency injection. |
Intermediate projects with focus on performance and ease of use. |
MobX |
Intermediate - Complex |
Steeper (Reactive) |
Reactive |
Automatic updates, clean syntax (after learning curve). |
Complex scenarios benefiting from reactive state management. |
Bloc |
Complex |
Steeper |
Unidirectional |
Predictable, testable, good for complex business logic. |
Large-scale applications with intricate state management needs. |
Redux |
Highly Complex |
Steeper |
Unidirectional |
Centralized store, predictable state changes. |
Very complex applications requiring centralized state management. |
Best Practices for Effective State Management in Flutter
State management plays a vital role in crafting a responsive and efficient Flutter app. Here are some key best practices to keep your app in top shape:
- Choose Wisely: Select the state management approach that aligns with your project's complexity. For basic needs, built-in widgets might suffice, while complex apps might benefit from solutions like Bloc or Redux.
- Separation of Concerns: Maintain a clear distinction between your UI (presentation) and business logic (data handling). This promotes cleaner code and easier maintenance.
- Minimize State: Only manage data that's truly dynamic and essential for your UI. Avoid unnecessary state updates that can impact performance.
- Leverage Stateless Widgets: Whenever possible, utilize stateless widgets for UI components that don't require state management. This simplifies your code and improves efficiency.
- Immutable Data: When feasible, strive to use immutable data structures (like those that can't be changed). This enhances predictability and simplifies reasoning about your app's state.
- Effective Testing: Integrate state management testing practices into your development workflow. This ensures your state management logic functions as expected.
By following these practices, you can build a Flutter app with a well-structured and efficient state management system, laying the foundation for a responsive and maintainable user experience.
>> Read more: Top 9 Flutter App Development Companies
Final Thoughts
State management in Flutter is the art of keeping your app's data and UI in perfect harmony. By choosing the right approach, following best practices, and keeping your state lean, you can build responsive, efficient, and maintainable Flutter applications. From simple built-in widgets to robust solutions like Bloc and Redux, there's a state management approach waiting to streamline your development journey. So, dive in, experiment, and empower your Flutter apps with the power of effective state management!
Struggling with Flutter development? Relia Software offers comprehensive end-to-end Flutter development services. Our skilled developers are experts in various state management approaches, ensuring your app delivers a seamless user experience. Let's discuss your project and bring your Flutter vision to life!
>>> Follow and Contact Relia Software for more information!
- coding
- Mobile App Development
- Web application Development