Server-Side Rendering (SSR) has become a fundamental aspect of modern web development, providing enhanced performance, better SEO, and a more efficient user experience. While popular frameworks like Next.js simplify SSR implementation, the concept is not limited to any single technology stack.
This article explores SSR in a broader context, covering its role in various stacks, how it differs from Client-Side Rendering (CSR), and the distinct advantages it brings to web applications. We’ll also dive into optimization strategies such as code-splitting, lazy loading, and caching, while showcasing real-world examples of how SSR enhances the user experience in popular frameworks like Next.js, Remix, Razzle, and Gatsby.
What is Server-Side Rendering?
Server-Side Rendering (SSR) is a technique where web pages are rendered on the server and sent to the client as fully-formed HTML. This means the HTML generated by the server is complete, including the content, which allows the browser to display the page immediately after the initial request.
For example, consider an eCommerce website where a user wants to view a product page. With SSR, when the user requests the product page, the server retrieves the product data (like images, descriptions, prices, etc.), renders the HTML for the full page, and sends it to the browser. The user sees the entire page content immediately without waiting for further loading or JavaScript execution.
React Client-Side Rendering vs Server-Side Rendering: Key Differences
In Client-Side Rendering (CSR), only a minimal HTML shell is sent to the client, while the browser's JavaScript fetches and renders the content. On the other hand, Server-Side Rendering (SSR) provides significant advantages, especially in terms of SEO and initial page load speeds, by rendering the HTML on the server before sending it to the browser.
Feature | Client-Side Rendering (CSR) | Server-Side Rendering (SSR) |
---|---|---|
Initial Load Time | Slower; content is generated in the browser. | Faster; pre-rendered HTML is served. |
SEO Optimization | Challenging; search engines may struggle with JavaScript-heavy content. | Excellent; fully-rendered HTML aids in crawling. |
Performance | Delayed interaction as JS loads first. | Faster initial interaction. |
JavaScript Dependency | Heavily reliant on client-side JavaScript. | Minimal; server handles initial rendering. |
Data Fetching | Handled on the client-side after the page loads. | Performed on the server before sending to the client. |
User Experience | Slower due to delayed content rendering. | Smoother and faster initial interaction. |
Initial Load Time: CSR shifts the content rendering to the browser, causing delays, whereas SSR generates the page content on the server, resulting in faster initial load times.
SEO Optimization: In CSR, search engines may face challenges indexing JavaScript-heavy content. Contrastly, SSR enhances SEO by serving fully-formed HTML for search engines to crawl easily.
Performance: CSR might suffer from initial delays as the browser waits for JavaScript to load and render the page, while SSR delivers a more immediate user interaction experience.
graph TD
A[User Request] -->|CSR| B[Server sends HTML shell]
B -->|CSR| C[Browser fetches JS]
C -->|CSR| D[Browser renders content]
A -->|SSR| E[Server renders content]
E -->|SSR| F[Server sends fully-rendered HTML]
F --> G[Browser displays content]
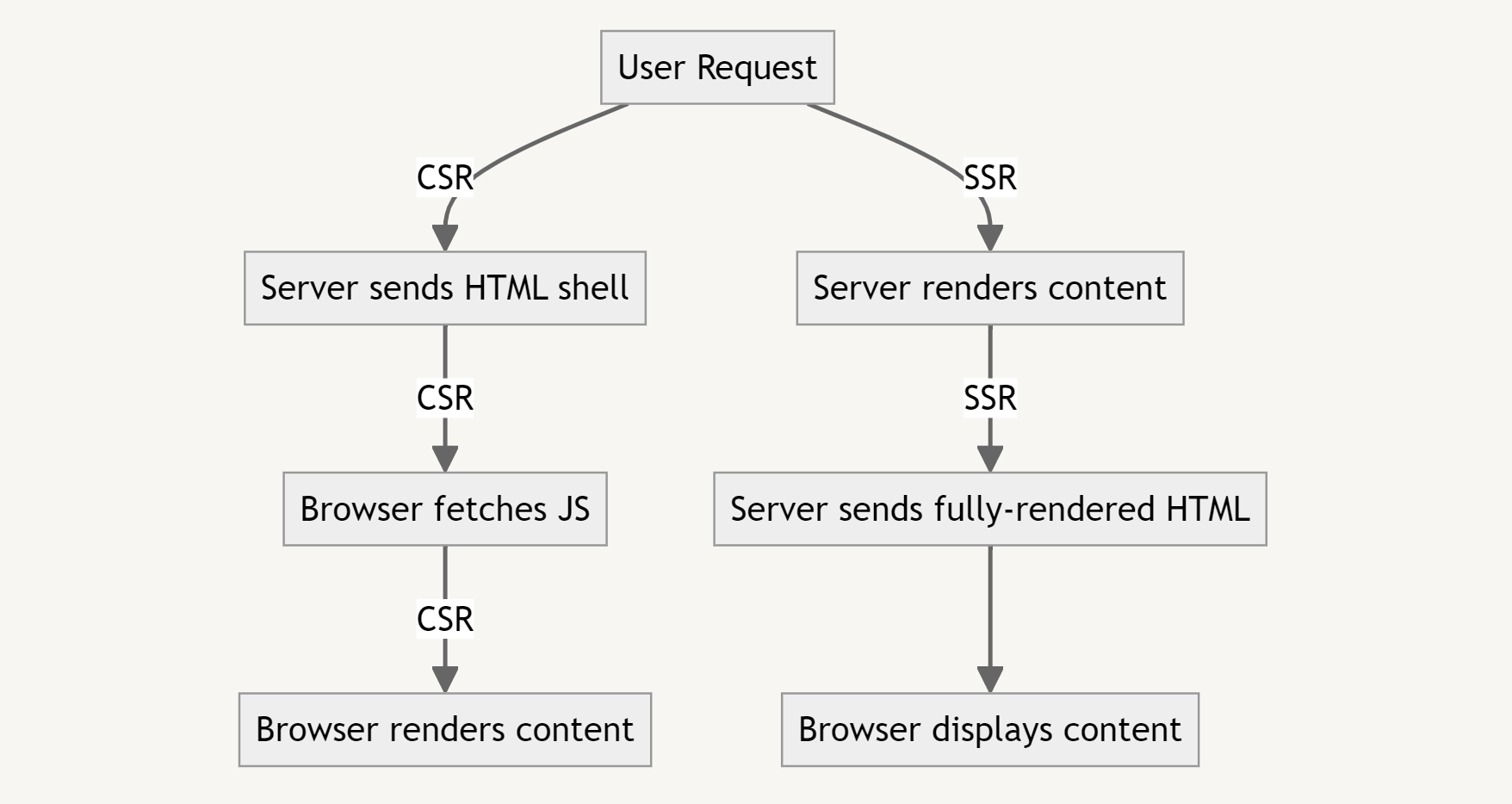
In this diagram, the CSR flow shows the browser waiting for JavaScript execution before rendering the page, causing delays in the user experience. Conversely, the SSR flow demonstrates how the server pre-renders content, sending fully-formed HTML to the client, which significantly reduces the time it takes for the content to appear.
Benefits of Implementing Server-Side Rendering in React
Faster Initial Load Time
A major challenge in web development is slow page loads on unreliable networks or devices. SSR solves this by pre-rendering the HTML on the server and sending it to the client, allowing users to view content almost immediately. This approach is particularly useful for improving the perceived performance on slower networks, as the content appears quickly even if additional JavaScript features take longer to load.
Better SEO
Search engines, like Google, prioritize pre-rendered content for better indexing. With SSR, search engines receive fully-formed HTML that includes all necessary content, improving the crawlability of the site. This is especially critical for websites relying on organic traffic from search engines, as fully rendered pages offer better ranking potential and visibility.
Enhanced User Experience
With Server-Side Rendering (SSR), pages load faster because content is pre-rendered on the server. This is especially useful for users on mobile devices or slower connections, who can see the page content almost immediately. This faster load time reduces bounce rates and enhances user satisfaction, leading to higher engagement.
Improved Social Sharing
When users share your content on platforms like Facebook or Twitter, SSR ensures that the open graph meta tags and other metadata needed for social previews are included in the pre-rendered HTML. This results in more accurate and visually appealing previews, improving the likelihood of clicks and shares.
Faster Time to Interactive (TTI)
SSR enables the browser to display the pre-rendered HTML quickly, while JavaScript files are still loading in the background. This improves the time to interactive (TTI), ensuring that users can engage with the content sooner, which is especially important for highly interactive applications.
Progressive Enhancement
With SSR, even users with older browsers or those who have JavaScript disabled can still access the core content of your site, as it is pre-rendered on the server. This ensures that your application works across a broader range of devices and browser environments, making it more inclusive.
Better Performance on Low-Powered Devices
Devices with limited computational power, such as older smartphones, often struggle to load large JavaScript files. SSR shifts the rendering burden to the server, ensuring that these devices can load and display the content more efficiently, improving performance.
Flexibility with Incremental Static Regeneration (ISR)
In frameworks like Next.js, SSR can be combined with Incremental Static Regeneration (ISR), where static pages are generated but updated incrementally as new data becomes available. This blend of SSR and Static Site Generation (SSG) offers flexibility for applications needing a balance between static performance and dynamic content updates.
React Server-Side Rendering in Different Technology Stacks
While Next.js is the most widely recognized framework for implementing Server-Side Rendering (SSR) in React applications, it’s not the only option. Developers can still implement React Server-Side Rendering without Next.js by leveraging other powerful frameworks like Remix, Razzle, and Gatsby. Let’s explore these prominent frameworks, their SSR capabilities, and when to consider each one:
Next.js
Next.js has gained widespread popularity in the React ecosystem due to its developer-friendly API and built-in SSR support. By default, Next.js enables developers to use both static generation and server-side rendering, depending on the use case. This flexibility makes Next.js ideal for large-scale applications where some pages can be pre-rendered while others require dynamic data fetching at request time.
Pros:
- Out-of-the-box SSR support, requiring minimal configuration.
- Supports Incremental Static Generation (ISR), allowing pages to be statically pre-rendered and updated incrementally.
- Strong community backing and extensive documentation.
- Built-in optimizations that enhance performance and SEO.
Cons:
- The higher-level abstraction in Next.js may limit control over more complex SSR implementations.
Example Use Case: E-commerce Website
For an e-commerce platform with thousands of products, Next.js can pre-render category pages using static generation, while employing server-side rendering for dynamic product pages where stock levels or prices change frequently.
Remix
Remix offers a newer, more sophisticated approach to SSR, emphasizing efficient data loading, seamless page transitions, and server-side logic management. While Next.js excels at combining static and server-rendered content, Remix shines in its ability to unify SSR with rich client-side interactivity.
Pros:
- Superior management of data flows between the client and server.
- Optimized for handling forms, mutations, and server-side actions.
- Provides efficient management of GET/POST operations via built-in API routes.
Cons:
- Still maturing as a framework, with less community support compared to Next.js.
Example Use Case: SaaS Application
For a SaaS platform where complex user interactions (like form handling or dynamic routes) are crucial, Remix offers precise control over data flow between the server and client, ensuring minimal re-renders and smooth transitions.
Razzle
Razzle provides an alternative to Next.js, offering more flexibility in terms of libraries and server-side setup. Designed to be unopinionated, Razzle allows developers to configure SSR with multiple libraries, such as React, Preact, or Vue, while maintaining low-level control over the server’s behavior.
Pros:
- Simplified SSR setup with flexibility to avoid rigid, opinionated configurations.
- Allows for greater control over bundling and server-side rendering logic.
- Works seamlessly with React and Preact, making it adaptable to different tech stacks.
Cons:
- Requires more manual configuration for features like routing and data fetching, unlike frameworks like Next.js.
>> Read more:
- Mastering React Server Actions for Optimizing Data Fetching
- React Suspense for Mastering Asynchronous Data Fetching
- Unlock the Power of GraphQL with React to Master Data Fetching
Example Use Case: Custom React Apps
For development teams needing more control over their SSR setup, Razzle provides flexibility while allowing developers to fine-tune the bundling and server-side rendering processes without rigid framework constraints.
Gatsby
Gatsby is a popular framework primarily focused on Static Site Generation (SSG) rather than SSR. However, it can also leverage SSR-like features to improve performance in certain dynamic applications. Gatsby excels at building fast, static websites and is ideal for content-heavy sites, especially those integrated with CMS platforms.
Pros:
- Blazing fast performance due to static page generation at build time.
- Extensive plugin ecosystem that makes integrating services (like GraphQL or CMS systems) easy.
- Built-in optimizations for performance, such as pre-fetching linked pages and image optimization.
- Strong community support and thorough documentation.
Cons:
- Primarily focused on SSG, so implementing true SSR may require additional setup.
- Requires build-time generation, which can be time-consuming for sites with a large number of pages or frequent content updates.
Example Use Case: Content-Driven Sites
For a blogging platform or content-heavy site that doesn’t need frequent updates, Gatsby’s static generation ensures that all pages are generated at build time, allowing for fast page loads with minimal server interaction.
Here is a comparison table of aforementioned React-based SSR frameworks:
Framework | Performance | Flexibility | Ease of Use | Best For |
Next.js | High | Supports both static & SSR | Very easy | Large apps, eCommerce |
Remix | High | Great control over data flows | Moderate | SaaS with complex data |
Razzle | Moderate | Highly flexible, unopinionated | Moderate | Custom React setups |
Gatsby | Extremely fast | Best for static sites | Easy for SSG | Content-heavy, static sites |
Best Practices for Optimizing Server-Side Rendering
Code-Splitting and Lazy Loading
Even though SSR reduces initial load times, large JavaScript bundles can still slow down interactions on the client side. By applying code-splitting and lazy loading, you can ensure only the essential parts of the page are loaded upfront, with larger components dynamically loaded later as needed. This further enhances performance by minimizing the amount of JavaScript required during the initial page load.
import dynamic from 'next/dynamic';
const DynamicComponent = dynamic(() => import('../components/LargeComponent'), {
suspense: true,
});
export default function HomePage() {
return (
<div>
<h1>Home Page</h1>
<React.Suspense fallback={<div>Loading...</div>}>
<DynamicComponent />
</React.Suspense>
</div>
);
}
In this example, dynamic imports ensure that LargeComponent is only loaded when needed, reducing the initial page load time for the user.
graph TD
A[User visits page] --> B[Server sends HTML and small JS bundle]
B -->|User interacts| C[LargeComponent is dynamically loaded]
This diagram illustrates how SSR and code-splitting can be combined to send minimal JavaScript on initial page load and then dynamically load larger components as needed.
Caching Strategies
SSR can be taxing on server resources, especially for high-traffic applications. Implement caching mechanisms to reduce server load. Use stale-while-revalidate or server-side caching to serve cached content and revalidate it in the background.
export default function handler(req, res) {
res.setHeader('Cache-Control', 's-maxage=60, stale-while-revalidate=59');
res.json({ message: 'This is a cached response.' });
}
This caching strategy ensures users get quick responses without sacrificing data freshness.
Data Fetching Optimization
For applications that rely heavily on external APIs, consider parallelizing API calls to speed up data fetching on the server. Use Promise.all
to fetch data concurrently.
export async function getServerSideProps() {
const [res1, res2] = await Promise.all([
fetch('<https://api.example.com/data1>'),
fetch('<https://api.example.com/data2>'),
]);
const data1 = await res1.json();
const data2 = await res2.json();
return { props: { data1, data2 } };
}
By parallelizing API requests, you reduce the total wait time for the server to fetch all necessary data, resulting in a faster response.
FAQs
When should developers choose SSR over Static Site Generation (SSG) or Client-Side Rendering (CSR) in React applications?
- SSR is best when dynamic content needs to be rendered on the fly (e.g., user-specific dashboards, eCommerce with changing prices).
- SSG is ideal for static, content-heavy sites (e.g., blogs, landing pages) that don’t need frequent updates.
- CSR is best for highly interactive applications where user interactions are a priority after the page loads (e.g., single-page applications with complex UI).
In what scenarios would a hybrid approach between SSR and CSR be beneficial in React applications?
A hybrid approach (SSR + CSR) works well in cases where static content should load immediately, but client-side interactivity is required afterward. For instance:
- E-commerce sites: Product pages can be server-rendered for fast load times and SEO, while user-specific interactions (like adding items to cart) can be handled on the client side.
- News platforms: Articles load quickly using SSR, but interactive features like live comments or polls can be handled using CSR.
How does SSR influence the choice of hosting environments for React applications?
SSR requires a hosting environment that can handle server-side logic and execute JavaScript on the server, so you need platforms that support Node.js (e.g., Vercel, AWS Lambda, Heroku). Additionally, scaling must be considered, as SSR increases server load compared to CSR or SSG. Managed platforms like Vercel and Netlify can handle SSR and scaling automatically.
>> Read more: Top 12 Best Javascript Frameworks for Your Projects
Conclusion
Server-Side Rendering remains a critical tool for building high-performance, SEO-optimized web applications across various technology stacks. Whether you are working with Next.js, Remix, or Razzle, understanding the best practices for caching, code splitting, and data optimization will ensure your applications deliver a smooth, fast user experience while minimizing server load.
Key Takeaways:
- Use SSR to improve initial load times and SEO.
- Implement caching to reduce server strain and improve performance.
- Apply code-splitting and lazy loading to keep client-side bundles efficient.
- Consider multiple SSR frameworks depending on your stack.
By following these best practices, you’ll be able to create modern, scalable, and high-performing web applications that can compete in today’s demanding web environment.
>>> Follow and Contact Relia Software for more information!
- web development
- Web application Development
- coding