Data fetching has been a critical aspect of web development since the early days of the internet. Initially, data fetching involved simple HTTP requests, evolving over time to more sophisticated methods like AJAX and REST APIs.
Despite these advancements, traditional data fetching methods often lead to over-fetching or under-fetching data, which can impact the performance and user experience of web applications. This is where GraphQL steps in, offering a more efficient and flexible approach to data fetching.
GraphQL, a query language for APIs developed by Facebook, allows developers to request exactly the data they need, and nothing more. This article will explore how GraphQL revolutionizes data fetching in React applications, providing a comprehensive guide to its implementation and optimization.
>> Read more:
- gRPC vs GraphQL: Choosing the Right API Technology
- React Suspense for Mastering Asynchronous Data Fetching
Understanding GraphQL
Definition and Key Concepts of GraphQL
GraphQL is a query language for your API, as well as a runtime for executing those queries by using a type system you define for your data. It enables clients to request exactly what they need, reducing the problems of over-fetching and under-fetching data.
Key concepts of GraphQL include:
- Schema: Defines the types and structure of the data available in your API.
- Query: Requests data from the server.
- Mutation: Modifies data on the server.
- Subscription: Allows clients to receive real-time updates from the server.
GraphQL vs REST APIs: Key Differences
GraphQL differs from REST by using a single endpoint to retrieve all required data, even from multiple resources. This consolidation simplifies the development process and improves efficiency. In contrast, REST often requires multiple endpoints and requests to gather related data, leading to inefficiency.
Feature | GraphQL | REST |
---|---|---|
Data Fetching | Client-driven, flexible queries | Predefined endpoints, fixed data structures |
Network Efficiency | Reduces over-fetching and under-fetching | Potential for multiple requests, data redundancy |
API Structure | Single endpoint, schema-driven | Multiple endpoints, resource-based |
Data Format | JSON | JSON (commonly used) |
Development Complexity | Increased upfront schema design, but more efficient in the long run | Simpler to implement initially, but can become complex with evolving data requirements |
Real-Time Updates | Supports subscriptions for real-time data | Limited to polling or websockets |
Benefits of Using GraphQL in React Projects
- Efficiency: Fetch only the data you need in a single request, reducing network overhead.
- Flexibility: Easily adapt queries as your data requirements change without modifying the server.
- Strong Typing: Provides clear documentation and validation through the schema, reducing errors.
- Real-time Capabilities: Subscriptions allow for real-time data updates, enhancing the responsiveness of your applications.
Setting Up GraphQL with React
Initial Setup of a React Project with GraphQL
To get started, create a new React project and install the necessary dependencies. Here's a basic setup using Create React App:
npx create-react-app graphql-react-demo
cd graphql-react-demo
npm install @apollo/client graphql
Configuring Apollo Client for State Management
Apollo Client is a comprehensive state management library for JavaScript that enables you to manage both local and remote data with GraphQL. Here's how to configure Apollo Client in your React project:
// src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { ApolloProvider, InMemoryCache, ApolloClient } from '@apollo/client';
const client = new ApolloClient({
uri: '<https://your-graphql-endpoint.com/graphql>',
cache: new InMemoryCache(),
});
ReactDOM.render(
<ApolloProvider client={client}>
<App />
</ApolloProvider>,
document.getElementById('root')
);
Setting Up a GraphQL Server with Node.js and Express
For a full-stack setup, you might need to create a GraphQL server. Here's a simple example using Node.js and Express:
// server.js
const express = require('express');
const { ApolloServer, gql } = require('apollo-server-express');
const typeDefs = gql`
type Query {
hello: String
}
`;
const resolvers = {
Query: {
hello: () => 'Hello, world!',
},
};
const server = new ApolloServer({ typeDefs, resolvers });
const app = express();
server.applyMiddleware({ app });
app.listen({ port: 4000 }, () =>
console.log(`Server ready at http://localhost:4000${server.graphqlPath}`)
);
Implementing GraphQL Queries and Mutations in React Components
To fetch data using GraphQL in your React components, you can define and execute queries directly within your component code. Here's an example:
// src/components/DataComponent.js
import React from 'react';
import { useQuery, gql } from '@apollo/client';
const GET_DATA = gql`
query {
hello
}
`;
const DataComponent = () => {
const { loading, error, data } = useQuery(GET_DATA);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return <div>{data.hello}</div>;
};
export default DataComponent;
In this example, the useQuery
hook from Apollo Client is used to execute the GET_DATA
query. The query retrieves a simple "hello" string from the server. When the component renders, it handles different states—loading, error, and successful data retrieval—displaying appropriate messages or data accordingly.
useQuery(GET_DATA)
: Executes theGET_DATA
query when the component is rendered.loading
: Boolean indicating whether the query is still in progress.error
: Provides details if the query fails.data
: Contains the result of the query when it succeeds.
This pattern is essential for integrating GraphQL into React components, allowing for efficient data fetching and seamless user experiences.
Optimizing Data Fetching with GraphQL
Techniques for Optimizing GraphQL Queries to Enhance Performance
- Reduce Complexity: Focus on fetching only the data your components require. Avoid including unnecessary fields and deeply nested queries to streamline data retrieval and reduce server load.
- Utilize Aliases: Improve query readability and prevent potential conflicts by renaming fields using aliases. This makes your queries clearer and easier to manage.
- Leverage Arguments: Make your queries more dynamic by using variables to filter, sort, and manage data based on user interaction or application state, ensuring you retrieve only what's needed.
Caching Strategies with Apollo Client
Apollo Client's InMemoryCache enables efficient data caching. You can configure caching policies based on specific needs:
- Cache-and-Network: Fetches data from the cache first and simultaneously makes a network request to update the cache with the latest data.
- Cache-First: Prioritizes cached data, making network requests only if the data is unavailable in the cache.
- Network-Only: Bypasses the cache and fetches data directly from the server.
Here's an example demonstrating basic caching configuration:
const client = new ApolloClient({
uri: '<https://your-graphql-endpoint.com/graphql>',
cache: new InMemoryCache({
typePolicies: {
Query: {
fields: {
hello: {
read(existingData) {
return existingData || 'Cached Hello';
},
},
},
},
},
}),
});
Using Fragments and Batch Requests Efficiently
Fragments: Use fragments as reusable building blocks in your queries. They promote code maintainability and reduce repetition by allowing you to define common fields once and reuse them across multiple queries.
Batch Requests: Combine multiple independent queries into a single request to minimize network round trips and enhance performance. While Apollo Client supports automatic batching for certain operations, manual batching may be necessary for more complex scenarios to achieve optimal efficiency.
Here is a code example with fragment and batch request:
// src/components/DataComponent.js
import { gql, useQuery } from '@apollo/client';
const USER_FRAGMENT = gql`
fragment UserFragment on User {
id
name
email
}
`;
const GET_USERS = gql`
query {
users {
...UserFragment
}
}
${USER_FRAGMENT}
`;
const DataComponent = () => {
const { loading, error, data } = useQuery(GET_USERS);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<div>
{data.users.map(user => (
<div key={user.id}>{user.name}</div>
))}
</div>
);
};
export default DataComponent;
Handling Real-Time Updates with GraphQL
Implementing Subscriptions for Real-Time Updates
GraphQL subscriptions enable real-time data updates, and Apollo Client supports these via WebSocket. Here's how you can set it up:
// src/index.js
import { ApolloClient, InMemoryCache, ApolloProvider, split } from '@apollo/client';
import { WebSocketLink } from '@apollo/client/link/ws';
import { HttpLink } from '@apollo/client/link/http';
import { getMainDefinition } from '@apollo/client/utilities';
const httpLink = new HttpLink({ uri: '<https://your-graphql-endpoint.com/graphql>' });
const wsLink = new WebSocketLink({
uri: `ws://your-graphql-endpoint.com/graphql`,
options: {
reconnect: true,
},
});
const splitLink = split(
({ query }) => {
const definition = getMainDefinition(query);
return (
definition.kind === 'OperationDefinition' &&
definition.operation === 'subscription'
);
},
wsLink,
httpLink,
);
const client = new ApolloClient({
link: splitLink,
cache: new InMemoryCache(),
});
ReactDOM.render(
<ApolloProvider client={client}>
<App />
</ApolloProvider>,
document.getElementById('root')
);
Practical Examples of Real-Time Data Handling in React
Here's an example of implementing a subscription to handle real-time updates:
// src/components/SubscriptionComponent.js
import React from 'react';
import { useSubscription, gql } from '@apollo/client';
const SUBSCRIBE_TO_DATA = gql`
subscription {
dataUpdated {
id
content
}
}
`;
const SubscriptionComponent = () => {
const { data, loading } = useSubscription(SUBSCRIBE_TO_DATA);
if (loading) return <p>Loading...</p>;
return (
<div>
{data.dataUpdated.map(item => (
<div key={item.id}>{item.content}</div>
))}
</div>
);
};
export default SubscriptionComponent;
Explanation:
- WebSocketLink: Used to establish a WebSocket connection for handling subscriptions.
- splitLink: Decides whether to use the HTTP or WebSocket link based on the type of operation.
- useSubscription: Hook that subscribes to real-time updates and automatically updates your UI as data changes.
This setup allows your React components to efficiently handle real-time updates using GraphQL subscriptions.
>> Read more:
- Mastering Axios in React.js for Effective API Management
- Demystifying React Redux for Centralized State Management
Advanced Features of GraphQL with React
Using Middleware for Enhanced GraphQL Capabilities
Middleware can enhance GraphQL capabilities by handling tasks such as authentication, logging, and error handling. Apollo Server supports middleware for these purposes:
// server.js
const { ApolloServer } = require('apollo-server-express');
const express = require('express');
const server = new ApolloServer({
typeDefs,
resolvers,
context: ({ req }) => {
const token = req.headers.authorization || '';
// Validate token and add user to context
return { user };
},
});
const app = express();
server.applyMiddleware({ app });
app.listen({ port: 4000 }, () =>
console.log(`Server ready at http://localhost:4000${server.graphqlPath}`)
);
Combining GraphQL with Other React Features Like Hooks and Context
Integrate GraphQL with React hooks and context API to manage state and provide data across your application efficiently. Combining these features enhances the flexibility and maintainability of your application.
// DataContext.js
import React, { createContext, useContext, useState } from 'react';
const DataContext = createContext();
export const DataProvider = ({ children }) => {
const [data, setData] = useState(null);
return (
<DataContext.Provider value={{ data, setData }}>
{children}
</DataContext.Provider>
);
};
export const useData = () => useContext(DataContext);
// DataComponent.js
import React, { useEffect } from 'react';
import { useData } from './DataContext';
import { useQuery, gql } from '@apollo/client';
const GET_DATA = gql`
query {
hello
}
`;
const DataComponent = () => {
const { data, setData } = useData();
const { loading, error, data: queryData } = useQuery(GET_DATA);
useEffect(() => {
if (queryData) {
setData(queryData.hello);
}
}, [queryData, setData]);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return <div>{data}</div>;
};
export default DataComponent;
Leveraging Schema Stitching and Federation
Schema stitching and federation are advanced techniques for combining multiple GraphQL schemas. This allows you to build more scalable and modular APIs.
// federatedSchema.js
const { ApolloServer } = require('apollo-server');
const { buildFederatedSchema } = require('@apollo/federation');
const gql = require('graphql-tag');
const typeDefs = gql`
type Query {
hello: String
}
`;
const resolvers = {
Query: {
hello: () => 'Hello from federated schema',
},
};
const server = new ApolloServer({
schema: buildFederatedSchema([{ typeDefs, resolvers }]),
});
server.listen({ port: 4001 }).then(({ url }) => {
console.log(`Federated server ready at ${url}`);
});
Best Practices for Using GraphQL with React
Security Considerations
Ensure that your GraphQL API is secure by validating inputs and implementing proper authentication and authorization mechanisms. This helps protect your application from common security vulnerabilities such as unauthorized access and injection attacks.
// server.js (with authentication)
const { ApolloServer } = require('apollo-server-express');
const express = require('express');
const server = new ApolloServer({
typeDefs,
resolvers,
context: ({ req }) => {
const token = req.headers.authorization || '';
// Validate token and add user to context
return { user: validateToken(token) };
},
});
const app = express();
server.applyMiddleware({ app });
app.listen({ port: 4000 }, () =>
console.log(`Server ready at http://localhost:4000${server.graphqlPath}`)
);
Error Handling and Retry Mechanisms
Implement robust error handling and retry mechanisms to manage data fetching failures gracefully. These mechanisms ensure that your application can recover from errors and maintain a smooth user experience.
// Retry mechanism example
const fetchData = async (query, retries = 3) => {
try {
const data = await fetchDataFromDatabase(query);
return data;
} catch (error) {
if (retries > 0) {
return fetchData(query, retries - 1);
} else {
throw error;
}
}
};
Minimizing Server Load and Optimizing Server Responses
To enhance performance and scalability, employ strategies such as:
- Caching: Utilize caching strategies (in-memory, distributed) to reduce database load and improve response times.
- Load Balancing: Distribute traffic across multiple servers to handle increased load.
- Database Optimization: Optimize database queries, indexes, and schema for efficient data retrieval.
- Code Optimization: Write efficient GraphQL queries and resolve data efficiently on the server.
These techniques help minimize server load and reduce latency, ensuring faster and more reliable data fetching.
Troubleshooting and Debugging
Effective troubleshooting and debugging are essential for maintaining a reliable GraphQL and React application.
Common Issues in GraphQL with React and Their Solutions
- Network errors: Implement retry logic, exponential backoff, and offline support.
- Server timeouts: Increase server-side timeout settings, optimize query performance, and implement client-side retry mechanisms.
- Data inconsistencies: Verify data integrity, implement data validation, and use optimistic updates for UI responsiveness.
Debugging Tools and Techniques
Use various tools and techniques to debug React server actions efficiently. Popular tools include Chrome DevTools, Postman, and server-side logging frameworks like Winston or Morgan. Implement structured logging to capture detailed information about requests and responses, which helps diagnose issues quickly.
// server.js (using Morgan for logging)
const morgan = require('morgan');
app.use(morgan('combined'));
Conclusion
Optimizing data fetching with GraphQL can significantly enhance the performance and responsiveness of your React applications. By understanding the concepts, setting up GraphQL, implementing advanced techniques, and following best practices, you can create efficient, scalable, and reliable data fetching solutions. Embrace these strategies to take your React applications to the next level.
For further reading and resources, consider these additional links:
- React Official Documentation
- GraphQL Official Documentation
- Apollo Client Documentation
- GraphQL Subscriptions
- GraphQL Security Best Practices
By integrating these advanced techniques and best practices into your React projects, you'll be well-equipped to handle data fetching efficiently and effectively. Happy coding! 😊💻✨
>>> Follow and Contact Relia Software for more information!
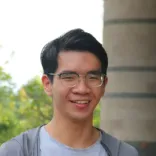
The Author
- Web application Development
- development
- Share
Table of Contents