The programming landscape in 2025 continues to evolve rapidly. Of all them, Rust and Go remains two of the most popular modern languages for systems and web development. This technical comparison provides an in-depth analysis of Rust vs Go across essential dimensions including performance, memory management, concurrency models, and use cases. So, you can make informed decisions based on your project requirements.
Quickly check the comparison table below:
Feature | Rust | Go |
Design Philosophy | Safety, control, zero-cost abstractions | Simplicity, speed, ease of use |
Memory Management | Ownership model (no GC) | Automatic garbage collection |
Performance | Faster execution, low-level optimization | Faster compilation and iteration |
Concurrency | Safe threads, async/await, compile-time checks | Goroutines + channels |
Learning Curve | Steep (ownership, lifetimes, traits) | Gentle (minimal syntax, fewer concepts) |
Error Handling | Result type, enforced handling | Return-value-based, explicit checks |
Syntax & Features | Rich (traits, macros, match) | Minimal (single loop, structural typing) |
Tooling & Ecosystem | Cargo, Clippy, expanding crates.io | gofmt, strong standard library, mature tooling |
Best For | Systems programming, performance-critical apps | Web servers, microservices, cloud tools |
Executable Output | Standalone binaries | Standalone binaries |
Popularity (2025) | Highly loved (84% want to keep using it) | Broadly adopted (especially in cloud/devops) |
Language Design Philosophy
Rust and Go were created with fundamentally different philosophies, shaping their capabilities and ideal use cases.
Go: Simplicity and Efficiency
Go, developed at Google, was designed with simplicity and pragmatism as core values. The language prioritizes developer productivity and readability, making it accessible for teams of varying experience levels. Go's design philosophy embraces minimalism, with a clean syntax and straightforward approach to coding that facilitates rapid development cycles.
This simplicity-first approach makes Go particularly suitable for large-scale development environments where multiple developers need to collaborate efficiently. Google backs Go specifically because it fits their fast-paced, complex infrastructure needs. Go maintains an accessible learning curve that doesn't suffer from developer turnover issues.
Rust: Safety and Control
Contrary to Go's focus on simplicity, Rust prioritizes safety and performance without compromising productivity. Born out of Mozilla's Research division, Rust was designed to eliminate common programming errors at compile time, particularly those related to memory management.
Rust's philosophy centers on providing developers with high control over system resources while preventing memory-related vulnerabilities through its ownership model. This approach makes Rust particularly valuable for applications where performance and security are top concerns. The language follows strict safety guidelines that, while initially more challenging to work with, ultimately produce more robust software.
Memory Management and Safety
Memory management represents one of the most significant differences between these 2 languages, affecting everything from performance to development experience.
Rust's Ownership Model
Rust implements memory safety through its ownership system. This system integrates concepts of ownership, borrowing, and lifetimes to facilitate efficient memory allocation and deallocation. It uses a built-in borrow checker in the compiler that ensures data references don't outlive their corresponding data in memory.
The ownership model eliminates entire classes of bugs at compile time rather than runtime, including:
- Use-after-free errors
- Double-free errors
- Data races in concurrent code
- Null pointer dereferences
Importantly, Rust achieves this safety without relying on garbage collection, resulting in predictable performance and reduced overhead. However, Rust programs can still have memory safety vulnerabilities even when no code is marked as "unsafe". But, these scenarios are significantly less common than in languages without Rust's safety guarantees.
This example shows Rust's move semantics. Once s
is moved into the function, it can't be used again. This ensures memory safety by design.
fn main() {
let s = String::from("hello");
takes_ownership(s); // s is moved here
// println!("{}", s); // Error: value borrowed here after move
}
fn takes_ownership(some_string: String) {
println!("{}", some_string);
}
Go's Garbage Collection
Go utilizes an automated garbage collector to manage memory, prioritizing simplicity and developer productivity over the fine-grained control provided by Rust. This approach frees developers from manual memory management concerns but introduces some performance overhead from garbage collection pauses.
Go's approach is particularly advantageous for:
- Web services and microservices where predictable latency is more important than absolute performance
- Applications where development speed takes priority over resource efficiency
- Scenarios where the complexity of manual memory management would increase development time and bug potential
While Go's garbage collector is highly optimized and continues to improve, it still introduces some overhead compared to Rust's zero-cost abstractions.
package main
import "fmt"
func main() {
s := "hello"
fmt.Println(s) // s can still be used freely
}
In Go, you don't have to think about ownership. The runtime handles allocation and cleanup, so variables like s
are accessible after being passed around.
Performance
Performance considerations vary widely by use case, but benchmarks provide insights into general capabilities.
Rust: Raw Execution Speed
In most benchmark comparisons, Rust consistently outperforms Go in raw execution speed. For tested algorithms, optimized Rust code was at least 30 percent faster than the most optimized Go code. The Benchmarks Game project shows Rust outperforming Go across multiple test categories including n-body simulation, spectral-norm calculation, and fannkuch-redux.
Rust's performance advantages stem from:
- Zero-cost abstractions that enable high-level programming without runtime overhead
- Lack of garbage collection pauses
- Ability to optimize at the hardware level
- Fine-grained memory control
Take a look at the example below:
fn main() {
let mut sum = 0;
for i in 1..=1_000_000 {
sum += i;
}
println!("Sum: {}", sum);
}
This tight loop runs very efficiently in Rust, with no runtime overhead or GC interference.
Go: Compilation and Development Speed
Go prioritizes fast compilation and development cycles over maximum runtime performance. This design choice creates significant developer productivity benefits, particularly in environments requiring rapid iteration. Go's compiler is extremely fast, often completing in seconds even for large projects, compared to Rust's more thorough but time-consuming compilation process.
As the same example, Go version is clean and readable, but may involve minor overhead due to background memory management.
package main
import "fmt"
func main() {
sum := 0
for i := 1; i <= 1000000; i++ {
sum += i
}
fmt.Println("Sum:", sum)
}
Both languages produce standalone binaries that don't require runtime environments, making deployment straightforward across various platforms.
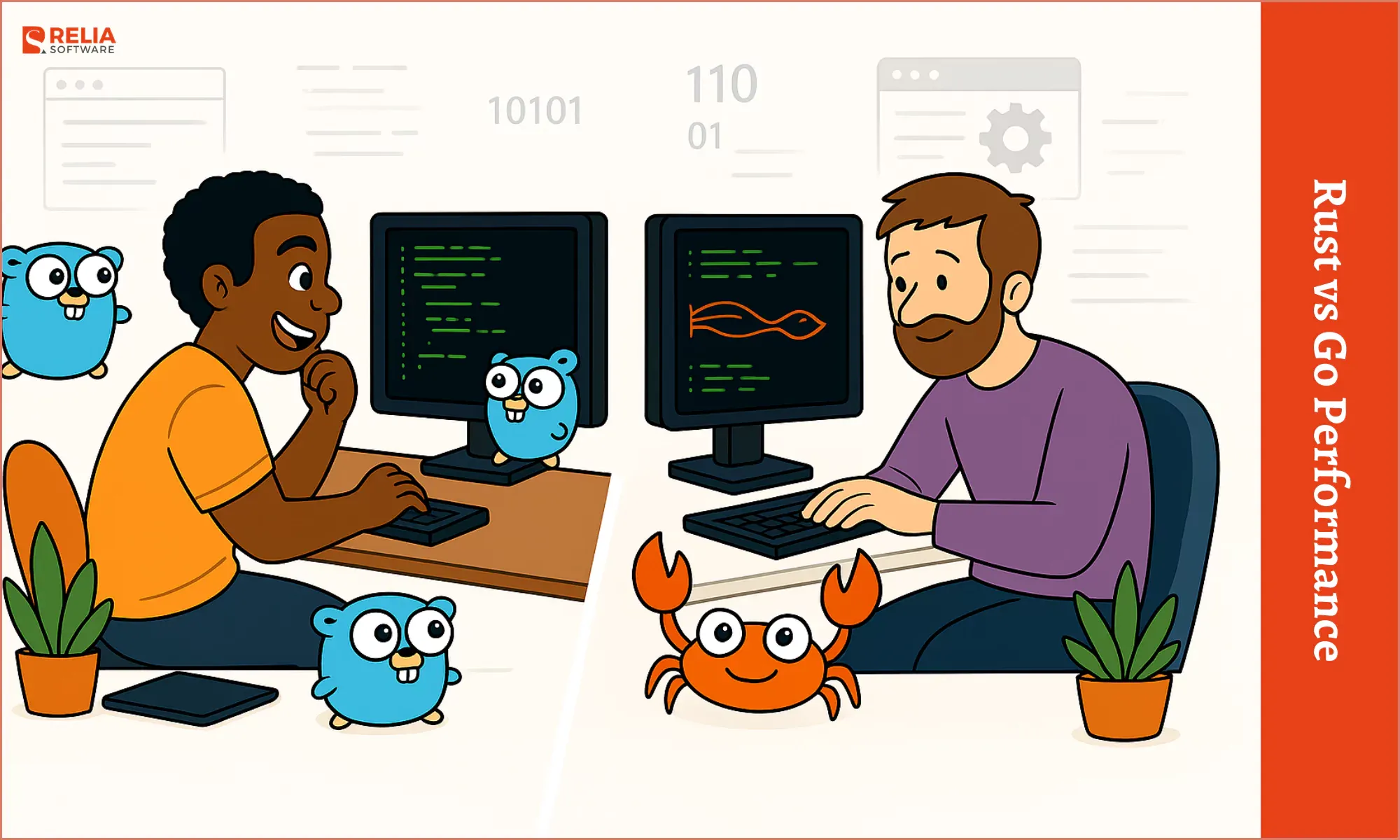
Concurrency Models
Both languages excel at concurrency but take different approaches that reflect their core philosophies.
Rust's Thread Safety Guarantees
Rust approaches concurrency with its core principle of memory safety firmly intact. The language guarantees thread safety through its ownership and type systems, eliminating data races at compile time.
Rust offers several approaches to concurrency:
- System threads with guaranteed safety through the ownership model
- Async/await syntax for asynchronous programming
- The "rayon" crate for parallel iterations
- Message-passing concurrency similar to Go's channels
Example:
use std::thread;
fn main() {
let handle = thread::spawn(|| {
for _ in 0..3 {
println!("From spawned thread");
}
});
for _ in 0..3 {
println!("From main thread");
}
handle.join().unwrap();
}
Go's Goroutines and Channels
Go's concurrency model centers around goroutines (lightweight threads) and channels (for communication), making concurrent programming remarkably accessible. Goroutines are extremely lightweight, requiring only a few kilobytes of memory, allowing programs to spawn thousands without significant performance impact.
The goroutine model implements concurrency through the following mechanisms:
- Lightweight threads managed by the Go runtime rather than the operating system
- Channel-based communication between goroutines
- The
go
keyword for simple goroutine spawning - Built-in synchronization primitives like
WaitGroup
This approach is particularly well-suited for applications that must handle many independent operations simultaneously, such as web servers and distributed systems.
>> Read more: Mastering 6 Golang Concurrency Patterns to Level Up Your Apps
This example runs two functions concurrently. The go
keyword spawns a new goroutine, letting both print loops run in parallel.
package main
import (
"fmt"
"time"
)
func say(msg string) {
for i := 0; i < 3; i++ {
fmt.Println(msg)
time.Sleep(100 * time.Millisecond)
}
}
func main() {
go say("Goroutine")
say("Main")
}
While Rust's concurrency model requires more upfront consideration than Go's, it provides stronger safety guarantees and helps prevent subtle bugs that might otherwise only appear under specific timing conditions.
Learning Curve and Development Speed
The learning experience and development velocity differ significantly between these languages.
Rust's Steeper Learning Path
Rust has a notably steeper learning curve, primarily due to its ownership system and more complex type system. Developers must understand concepts like ownership, borrowing, lifetimes, and traits before becoming productive. It often requires weeks or months to gain comfort with the language.
The learning investment provides significant returns in the form of:
- Fewer runtime bugs and crashes
- Better performance without manual optimization
- More confidence when making changes to concurrent code
- Earlier detection of architectural issues
According to the Stack Overflow Developer Survey, 84% of Rust developers want to continue using it (compared to 60% for Go), suggesting that despite the initial difficulty, developers find the language rewarding once mastered.
Go's Accessibility
Go was designed to be easily learned and quickly productive, with a deliberately limited set of features and straightforward syntax. Most developers can become productive with Go in a matter of days or weeks, making it particularly suitable for teams with varied experience levels.
Go's simplicity comes from:
- Minimal syntax with few keywords
- Straightforward error handling through return values
- Limited ways to solve problems (reducing "too many ways to do it" confusion)
- Excellent documentation and standardized formatting (via
gofmt
)
This simplicity translates to better code readability, which becomes increasingly important as teams and codebases grow.
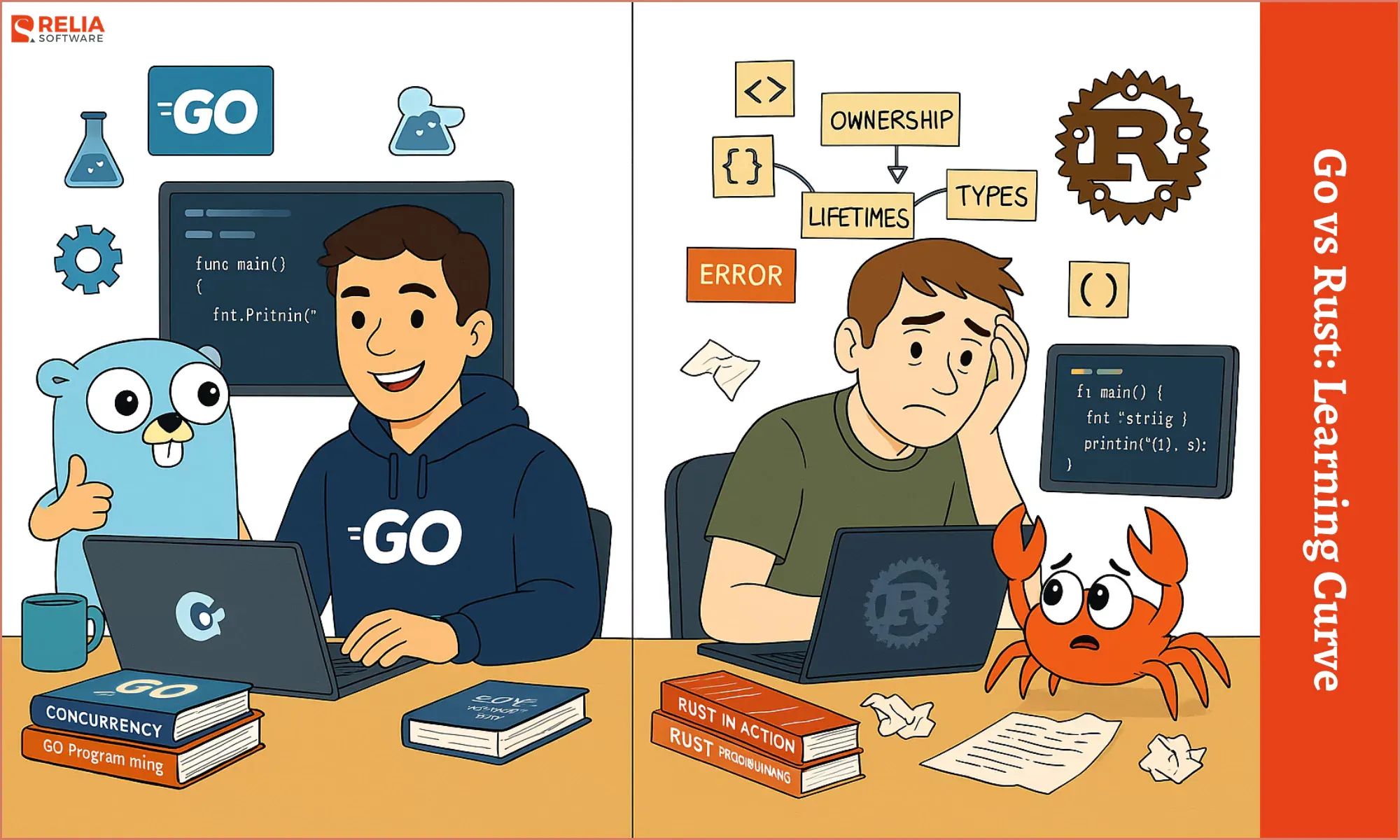
Features and Syntax
Both languages provide modern features but differ in their approach to language design.
Rust's Rich Feature Set
Rust offers a more extensive feature set, providing greater flexibility and control. Notable features include:
- A sophisticated trait system (similar to interfaces but more powerful)
- Pattern matching and algebraic data types
- Macros for metaprogramming
- Zero-cost abstractions
- Fine-grained control over memory layout
Rust's error handling uses the Result
type and the ?
operator, offering more ergonomic error propagation than Go's explicit checking. The language provides three distinct loop constructs (for
, while
, and loop
), giving developers more options for expressing iteration patterns.
Rust also makes it easier to call C/C++ code compared to Go, enhancing interoperability with existing systems.
Go's Minimalist Approach
Go's feature set is intentionally limited, focusing on simplicity and consistency. Key language features include:
- Interfaces for polymorphism (without inheritance)
- Strong built-in concurrency primitives
- Generics (added in Go 1.18)
- A robust standard library
- Structural typing
Go uses explicit error checking rather than exceptions, requiring errors to be handled directly at call sites. The language provides a single looping construct (for
) that can be used to implement various loop patterns, further emphasizing simplicity.
Popularity
Both languages benefit from strong communities and growing ecosystems, though with different characteristics.
Rust's Growing Community
Rust's ecosystem continues to grow rapidly, with particularly strong representation in systems programming, WebAssembly, and performance-critical applications. Notable ecosystem components include:
- Cargo, Rust's package manager and build system
- Crates.io, hosting a growing collection of libraries
- Strong tooling including Rustfmt, Clippy, and Rustup
- Active community development of libraries across domains
Go's Mature Ecosystem
Go has developed a mature ecosystem particularly strong in cloud infrastructure, microservices, and networking. Key ecosystem aspects include:
- Robust tooling integrated into the language (testing, formatting, documentation)
- Strong adoption in Kubernetes and container ecosystems
- Excellent standard library covering most common tasks
- Consistent package management through Go modules
Go's ecosystem is especially well-developed for web services, DevOps tools, and cloud-native applications.
According to recent surveys, both Go and Rust have similar overall popularity levels (around 13%), though Rust shows higher desirability among developers who haven't yet used it.
When to Use Rust vs Go?
The different strengths of these languages make them suitable for different domains.
Rust
Rust finds its strongest applications in areas demanding maximum performance, security, and resource efficiency:
- Systems programming (operating systems, device drivers)
- Performance-critical applications
- Embedded systems with resource constraints
- Game engines and real-time applications
- Security-critical infrastructure
- WebAssembly applications
Rust is increasingly adopted in environments where memory safety vulnerabilities would be particularly costly, with major tech companies integrating it into security-critical components.
Go
Go excels in scenarios requiring rapid development of scalable network services:
- Cloud infrastructure and microservices
- Web APIs and services
- DevOps and infrastructure tools
- Distributed systems
- Command-line utilities
Many major companies use Go for backend services where simplicity, deployment ease, and built-in concurrency offer significant advantages. Go's memory efficiency and fast startup times make it particularly well-suited for containerized deployment models like Kubernetes (which itself is written in Go).
Conclusion
The choice between Rust and Go depends significantly on project requirements, team expertise, and development priorities. Both languages continue to evolve and expand their capabilities in 2025, with each maintaining distinct advantages in their target domains.
Go remains the preferred choice when development speed, simplicity, and built-in concurrency are paramount. Its approachable syntax and excellent tooling make it ideal for web services, cloud infrastructure, and scenarios where rapid iteration and deployment are essential.
Rust provides exceptional performance, memory safety, and fine-grained control, making it the superior option for systems programming, performance-critical applications, and scenarios where security vulnerabilities would be particularly damaging.
Many organizations benefit from using both languages strategically: Go for service-oriented architecture and developer productivity, and Rust for performance bottlenecks and security-critical components. Understanding the strengths and limitations of each empowers developers to make informed technology choices aligned with project goals and constraints.
>>> Follow and Contact Relia Software for more information!
- golang
- coding
- web development