Golang (or Go) is a statically typed, compiled programming language designed at Google. It is known for its simplicity, efficiency, and strong support for concurrent programming. Concurrency is a key feature that allows Go to handle multiple tasks simultaneously, making it ideal for modern applications that require high performance and responsiveness.
In this blog, we will explore the concept of concurrency, how it is implemented in Go, and why it is crucial for modern software development. We will cover the basics of Goroutines and Channels, the building blocks of concurrency in Go, and demonstrate how to use them effectively. Additionally, we will discuss synchronization techniques, error handling, and performance considerations to help you write robust and efficient concurrent programs in Go.
>> Read more:
- An In-Depth Guide for Using Go sync.Map with Code Sample
- Cloud Success Blueprint: Why Go Is A Perfect Match?
- The Ultimate Guide to Golang Structs with Code Example
- A Complete Guide to Debugging Go Applications with Go Tracing
What is Concurrency?
Concurrency is the ability of a system to handle multiple tasks simultaneously by interleaving their execution. This means that while tasks may not be executed at the same exact moment, they can progress independently and be managed in such a way that they appear to run simultaneously. Concurrency allows a program to be more efficient and responsive by making better use of available resources.
Why Concurrency in Golang?
Traditional threading models, such as those in Java and C++, have several limitations. Managing threads is complex and error-prone, leading to issues like deadlocks, race conditions, and synchronization challenges.
Threads consume significant system resources, requiring large memory stacks, which can result in high memory usage and poor scalability. As the number of threads increases, the overhead of context switching can degrade performance. Debugging multithreaded applications is also difficult due to non-deterministic execution.
Golang takes a different approach to concurrency with lightweight threads called Goroutines. These Goroutines have a small memory footprint, allowing developers to create a large number of them without significant overhead. This simplicity and efficiency empower developers to write concurrent programs that can handle multiple tasks effectively, improving overall responsiveness and performance.
Go's concurrency model is simple and intuitive, making it easier to write and maintain concurrent code. It scales well with modern multicore processors, handling thousands or even millions of Goroutines. Built-in tools like the race detector further enhance reliability, making Go an excellent choice for high-performance, concurrent applications.
Goroutines
Goroutines are the cornerstone of concurrency in Go. They are lightweight threads managed by the Go runtime, and enable concurrent execution of functions. Unlike traditional threads, Goroutines have a small memory footprint and can be created in large numbers with minimal overhead. The Go runtime includes an efficient scheduler that multiplexes Goroutines onto CPU cores, reducing context switching overhead and optimizing resource use.
How to Create and Manage Goroutines?
Creating a Goroutine is simple. You prepend the go
keyword to a function call. Once started, the function runs concurrently with the calling code. The Go runtime handles the scheduling and management of Goroutines, allowing developers to focus on the logic rather than the intricacies of threading.
package main
import (
"fmt"
"time"
)
func printNumbers() {
for i := 1; i <= 5; i++ {
fmt.Println(i)
time.Sleep(1 * time.Second)
}
}
func main() {
go printNumbers() // Start a Goroutine
fmt.Println("Goroutine started")
// Wait for user input to prevent main from exiting immediately
fmt.Scanln()
}
In this example, the printNumbers
function runs concurrently with the main function. The go
keyword starts printNumbers
as a Goroutine.
Goroutine Scheduling and Potential Blocking Scenarios
Goroutines are scheduled non-deterministically by the Go runtime. This means their execution order is not guaranteed, which can lead to different execution paths in different runs. The Go scheduler is efficient, but it’s crucial to handle potential blocking scenarios, where a Goroutine might be waiting for an operation to complete.
For instance, if a Goroutine is waiting on a channel operation or a resource, it can block the execution. Proper synchronization mechanisms, such as channels and the sync
package, are essential to manage these scenarios and ensure smooth concurrency.
package main
import (
"fmt"
"time"
)
func main() {
done := make(chan bool)
go func() {
time.Sleep(2 * time.Second)
fmt.Println("Goroutine finished")
done <- true
}()
fmt.Println("Waiting for Goroutine...")
<-done
fmt.Println("Main function finished")
}
The main function waits for the Goroutine to finish using a channel. This ensures that the main function does not exit before the Goroutine completes its execution.
Goroutines, with their simplicity and efficiency, make concurrent programming in Go powerful and accessible. Proper management and understanding of their scheduling and potential blocking scenarios are crucial for writing robust concurrent applications.
Channels
Channels in Go are powerful concurrency primitives that facilitate communication between Goroutines. They allow Goroutines to send and receive data, making synchronization straightforward and avoiding the complexities of shared memory. Channels ensure that data is passed safely between Goroutines, reducing the risk of race conditions and improving the overall reliability of concurrent programs.
2 Types of Channels: Unbuffered and Buffered
- Unbuffered Channels: Unbuffered channels are the default type of channel in Go. They provide a synchronous way of communication, meaning that both the sending and receiving Goroutines must be ready to perform the operation. The sender blocks until the receiver is ready, and vice versa.
- Buffered Channels: Buffered channels allow a specified number of elements to be stored in the channel. They provide asynchronous communication, meaning the sender can continue execution without waiting for the receiver, as long as the buffer is not full. Similarly, the receiver can continue execution without waiting for the sender, as long as the buffer is not empty.
Sending and Receiving Data using Channels
Channels are created using the make
function and can be used to send and receive values of a specified type. The <-
operator is used for both sending and receiving data.
package main
import "fmt"
func main() {
ch := make(chan int)
go func() {
ch <- 42 // Send data to the channel
}()
value := <-ch // Receive data from the channel
fmt.Println("Received value:", value)
}
In this example, a Goroutine sends the value 42
to the channel, and the main function receives it.
package main
import "fmt"
func main() {
ch := make(chan string, 2)
ch <- "Hello"
ch <- "World"
fmt.Println(<-ch) // Receive first value
fmt.Println(<-ch) // Receive second value
}
Here, the channel is buffered with a capacity of 2, allowing two values to be sent without requiring an immediate receiver.
package main
import (
"fmt"
"time"
)
func worker(id int, ch chan string) {
time.Sleep(time.Second)
ch <- fmt.Sprintf("Worker %d finished", id)
}
func main() {
ch := make(chan string)
for i := 1; i <= 3; i++ {
go worker(i, ch)
}
for i := 1; i <= 3; i++ {
fmt.Println(<-ch)
}
}
Three worker Goroutines send messages to the channel after completing their tasks. The main function receives and prints these messages.
Channels, whether unbuffered or buffered, are essential tools for managing concurrency in Go. They simplify communication and synchronization between Goroutines, leading to more readable and maintainable concurrent code.
Select Statement
The select
statement in Go is a powerful control structure that allows a Goroutine to wait on multiple communication operations. It is similar to a switch statement but is specifically designed for channels. The select
statement blocks until one of its cases can proceed, making it an essential tool for handling multiple channels concurrently.
The select
statement enables a Goroutine to listen on multiple channels and execute the case that is ready first. This is particularly useful for managing timeouts, multiplexing channels, and handling different types of communication simultaneously. By using select
, you can efficiently manage the flow of data between multiple Goroutines and channels.
Using select for Timeout
package main
import (
"fmt"
"time"
)
func main() {
ch := make(chan string)
go func() {
time.Sleep(3 * time.Second)
ch <- "Completed"
}()
select {
case msg := <-ch:
fmt.Println("Received:", msg)
case <-time.After(2 * time.Second):
fmt.Println("Timeout!")
}
}
Here, the select
statement is used to implement a timeout. If the Goroutine does not send a message to the channel within 2 seconds, the timeout case is executed.
Using select with Default Case
package main
import (
"fmt"
"time"
)
func main() {
ch := make(chan string)
timeout := time.After(1 * time.Second)
go func() {
time.Sleep(2 * time.Second)
ch <- "Task Completed"
}()
for {
select {
case msg := <-ch:
fmt.Println("Received:", msg)
return
case <-timeout:
fmt.Println("Operation timed out")
return
default:
fmt.Println("Waiting for tasks to complete...")
time.Sleep(500 * time.Millisecond)
}
}
}
In this example, the default
case allows the select
statement to proceed immediately if no other case is ready. This can be used for non-blocking channel operations or performing other tasks while waiting.
The select
statement enhances the flexibility and efficiency of concurrent programs in Go. By allowing a Goroutine to wait on multiple channels, handle timeouts, and perform non-blocking operations, it becomes a powerful tool for managing complex Golang concurrency patterns.
Synchronization
In concurrent programming, synchronization is essential to ensure that multiple Goroutines can safely access shared resources without causing data races, inconsistencies, or other errors. Proper synchronization mechanisms help coordinate the execution of Goroutines, making sure they work together harmoniously without stepping on each other's toes.
Using sync
package: WaitGroups, Mutexes, and Once
Go provides the sync
package, which includes several synchronization primitives to help manage concurrency effectively
- WaitGroups: WaitGroups are used to wait for a collection of Goroutines to finish executing. They provide a simple way to block the main Goroutine until all other Goroutines have completed their tasks.
package main
import (
"fmt"
"sync"
"time"
)
func worker(id int, wg *sync.WaitGroup) {
defer wg.Done() // Notify the WaitGroup that this Goroutine is done
fmt.Printf("Worker %d starting\n", id)
time.Sleep(time.Second)
fmt.Printf("Worker %d done\n", id)
}
func main() {
var wg sync.WaitGroup
for i := 1; i <= 3; i++ {
wg.Add(1) // Increment the WaitGroup counter
go worker(i, &wg)
}
wg.Wait() // Wait for all Goroutines to finish
fmt.Println("All workers done")
}
WaitGroups are used to wait for three worker Goroutines to complete their tasks before allowing the main function to exit.
- Mutexes: Mutexes (Mutual Exclusion Locks) are used to protect shared resources from concurrent access. By locking a resource, only one Goroutine can access it at a time, preventing data races and ensuring consistency.
package main
import (
"fmt"
"sync"
)
var (
counter int
mutex sync.Mutex
)
func increment(wg *sync.WaitGroup) {
defer wg.Done()
mutex.Lock()
counter++
mutex.Unlock()
}
func main() {
var wg sync.WaitGroup
for i := 0; i < 5; i++ {
wg.Add(1)
go increment(&wg)
}
wg.Wait()
fmt.Println("Final counter value:", counter)
}
This example demonstrates the use of a Mutex to protect a shared counter variable from concurrent access by multiple Goroutines.
- Once: The
sync.Once
type is used to ensure that a piece of code is executed only once, even if called from multiple Goroutines. This is useful for tasks like initializing shared resources.
package main
import (
"fmt"
"sync"
)
var once sync.Once
func initialize() {
fmt.Println("Initializing...")
}
func worker(wg *sync.WaitGroup) {
defer wg.Done()
once.Do(initialize) // Ensure initialization happens only once
fmt.Println("Worker executing")
}
func main() {
var wg sync.WaitGroup
for i := 0; i < 3; i++ {
wg.Add(1)
go worker(&wg)
}
wg.Wait()
fmt.Println("All workers done")
}
In this example, the sync.Once
type is used to ensure that the initialization function is called only once, even though multiple Goroutines may attempt to call it.
Error Handling in Concurrent Programs
Concurrent programming in Go presents several challenges, such as race conditions, deadlocks, resource contention, and synchronization errors. Proper error handling and synchronization are crucial to ensure reliable and efficient concurrent programs.
Synchronization Mechanisms
- Channels can be used to propagate errors from Goroutines to a central handler
- The
defer
keyword ensures cleanup and error handling code is executed appropriately. - To avoid shared state and minimize race conditions, it's best to use channels for communication between Goroutines.
Synchronization Primitives
Synchronization primitives from the sync
package, such as WaitGroups, Mutexes, and Once, help coordinate Goroutine execution and protect shared resources. For instance:
- WaitGroups ensure that all Goroutines complete before the main program continues.
- Mutexes prevent simultaneous access to shared resources
- Once ensures a block of code is executed only once.
Deadlock Prevention
Potential deadlocks, caused by Goroutines waiting indefinitely for each other, can be mitigated by:
- Acquiring locks in a consistent order;
- Using timeouts and
select
statements to prevent indefinite waits; - Releasing locks promptly.
By adhering to these practices and leveraging Go's synchronization tools, you can manage concurrency challenges effectively and build robust concurrent applications.
The example below is showing how we use channels to handle error propagation:
package main
import (
"fmt"
"time"
)
func worker(id int, results chan<- string, errors chan<- error) {
defer close(results)
defer close(errors)
// Simulate work
time.Sleep(1 * time.Second)
if id%2 == 0 {
results <- fmt.Sprintf("Worker %d completed successfully", id)
} else {
errors <- fmt.Errorf("Worker %d encountered an error", id)
}
}
func main() {
results := make(chan string)
errors := make(chan error)
for i := 1; i <= 3; i++ {
go worker(i, results, errors)
}
for i := 1; i <= 3; i++ {
select {
case res := <-results:
fmt.Println(res)
case err := <-errors:
fmt.Println("Error:", err)
}
}
}
Besides that, we provide another example to illustrate using the defer
for cleanup and error handling:
package main
import (
"fmt"
"os"
"sync"
)
func writeFile(filename string, data string, wg *sync.WaitGroup, errors chan<- error) {
defer wg.Done()
file, err := os.Create(filename)
if err != nil {
errors <- err
return
}
defer file.Close()
_, err = file.WriteString(data)
if err != nil {
errors <- err
}
}
func main() {
var wg sync.WaitGroup
errors := make(chan error, 1)
wg.Add(1)
go writeFile("example.txt", "Hello, Go!", &wg, errors)
wg.Wait()
close(errors)
for err := range errors {
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("File written successfully")
}
}
}
And in the last example, we will show you how to avoid deadlocks:
package main
import (
"fmt"
"sync"
"time"
)
func main() {
var mu1, mu2 sync.Mutex
go func() {
mu1.Lock()
defer mu1.Unlock()
time.Sleep(1 * time.Second) // Simulate work
mu2.Lock()
defer mu2.Unlock()
fmt.Println("Goroutine 1 completed")
}()
go func() {
mu2.Lock()
defer mu2.Unlock()
mu1.Lock()
defer mu1.Unlock()
fmt.Println("Goroutine 2 completed")
}()
time.Sleep(3 * time.Second)
fmt.Println("Main function completed")
}
Performance Considerations
Profiling and optimizing concurrent Go programs are crucial for ensuring efficient execution and resource utilization. Go provides built-in tools like pprof
and trace
for collecting detailed performance profiles, including CPU and memory usage, Goroutine activity, and blocking operations. Benchmarking with the testing
package helps identify slow operations and evaluate optimizations. Third-party tools like Grafana and Prometheus offer real-time performance monitoring.
Common performance bottlenecks include:
- Excessive Goroutine creation;
- Inefficient synchronization;
- Blocking operations (e.g., waiting for I/O);
- Memory leaks.
- Load imbalance.
Here are some ways for optimizing performance to avoid bottlenecks above:
- Limit Goroutine creation as possible;
- Use channels for efficient communication between Goroutines;
- Handle I/O operations asynchronously;
- Manage memory properly
- Ensure even work distribution among Goroutines;
- Regularly profile your code to identify and address performance bottlenecks.
By following the example below, you can enable the pprof that built-in by Go, and then you can try to access http://localhost:6060/debug/pprof/
in your web browser.
package main
import (
"log"
"net/http"
_ "net/http/pprof"
)
func main() {
go func() {
log.Println(http.ListenAndServe("localhost:6060", nil))
}()
// Your application code here
select {}
}
>> Read more:
- Practical SOLID in Golang: Single Responsibility Principle
- Mastering the Open/Closed Principle in Golang
- Mastering the Liskov Substitution Principle in Golang
- Mastering The Interface Segregation Principle in Golang
- Detailed Code Examples of Dependency Inversion Principle in Go
- Best Practices For Dependency Inversion in Golang
Conclusion
In this blog, we explored the concept of concurrency in Go, covering the basics of Goroutines and Channels, synchronization mechanisms, error handling, and performance considerations. We discussed how Goroutines and Channels simplify concurrent programming, making it more efficient and manageable.
We also highlighted the importance of proper synchronization using WaitGroups, Mutexes, and Once, and how to handle errors effectively in concurrent programs. Additionally, we touched on profiling tools and techniques to optimize the performance of Go applications.
Concurrency in Go offers significant benefits, including improved performance, scalability, and resource utilization. However, it also presents challenges such as race conditions, deadlocks, and synchronization complexities. By understanding and applying best practices, developers can harness the power of Go’s concurrency model to build robust and efficient applications.
We encourage you to explore and experiment with concurrency in your Go projects. By doing so, you can gain a deeper understanding of concurrent programming principles and leverage Go’s powerful tools and constructs to create high-performance software. Dive into Go’s concurrency features, profile your applications, and continually refine your approach to building concurrent systems.
>>> Follow and Contact Relia Software for more information!
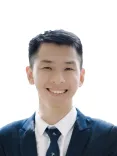
The Author
- golang
- coding
- mobile applications
- Share
Table of Contents