As your React application grows, ensuring that every component, hook, and API call works seamlessly becomes crucial. Enter unit testing—your first line of defense against unexpected bugs and performance issues. Whether you're testing a component's UI rendering or verifying complex custom hooks with Jest, mastering these React unit testing best practices will boost your application's stability and scalability.
In this guide, we will focus on how to unit test React components, custom hooks, and API fetch calls, along with snapshot testing for renderers. These techniques will help you build robust tests that keep your code clean and prevent bugs from sneaking into production.
>> Read more:
- Mastering React Context API for Streamlined Data Sharing
- Demystifying React Redux for Centralized State Management
Why Unit Testing Matters in React Development?
Key benefits of unit testing React applications:
- Early detection of bugs: Unit tests allow you to catch issues in React components and custom hooks early in the development cycle before they affect users.
- Maintainable codebase: By adding tests, you safeguard your project from regressions during refactoring code or adding new features.
- Confidence in refactoring: Well-tested code makes it easier to update React components and custom hooks without the fear of breaking functionality.
- Scalability of testing: As projects grow, unit testing scales by isolating components, ensuring each part functions independently, which keeps the system modular and easier to maintain.
When working on a React project, especially one that scales with multiple components, hooks, and APIs, unit tests become critical. They help maintain the quality of your application by ensuring that new features or bug fixes don't introduce regressions.
Setting Up React Unit Tests for React Applications
Installing Jest and React Testing Library
To begin unit testing in React, install Jest and React Testing Library to run tests and simulate user interactions efficiently.
npm install --save-dev jest @testing-library/react
Update the package.json
to include a test script:
"scripts": {
"test": "jest"
}
With this configuration, you’re now set to write and run tests for your React components, hooks, and API fetch calls, ensuring all parts of your application work as expected.
Organizing Test Files in Your React Project
To keep your tests organized and maintainable, store them close to the code they test. This structure makes it easier to locate and maintain both your code and tests.
Example folder structure:
src/
components/
Button.js
Button.test.js
For larger projects, consider grouping tests by feature or using a dedicated tests/
directory for better scalability. Keeping tests accessible and aligned with your code structure ensures that your project remains easy to manage as it grows.
Unit Testing for React Components
When testing React components, focus on what the user sees rather than internal logic. Tests should verify how a component behaves based on props, state, and user interactions, rather than how the component is implemented internally.
Example: Testing Component UI and Props
import { render, screen } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders component with correct text', () => {
render(<MyComponent text="Hello World" />);
expect(screen.getByText(/Hello World/i)).toBeInTheDocument();
});
This test ensures that the component renders the expected output based on its props. By focusing on the rendered UI, you ensure that your test will remain stable even if internal logic changes.
Unit Testing for React Hooks
Hooks often drive the business logic of React components, making them critical to test. If your hook’s logic is independent of the component, it’s better to test it directly using Jest and React Testing Library’s renderHook
from its hooks testing extension.
Example: Testing Custom Hook Logic
import { renderHook, act } from '@testing-library/react-hooks';
import { useMyCustomHook } from './useMyCustomHook';
test('should increment value', () => {
const { result } = renderHook(() => useMyCustomHook());
act(() => {
result.current.increment();
});
expect(result.current.count).toBe(1);
});
In this case, we’re testing a custom hook that increments a counter. The act function ensures that state changes are applied immediately, allowing us to verify the logic synchronously.
>> Read more: Mastering Asynchronous Testing with React Testing Library
Mermaid Decision Tree:
graph TD
A[Do You Need to Test Hook Behavior?]
A -->|Yes| B[Is Hook Logic Independent?]
A -->|No| E[Test Component Behavior]
B -->|Yes| C["Test Hook Directly (renderHook)"]
B -->|No| D[Test via Component]
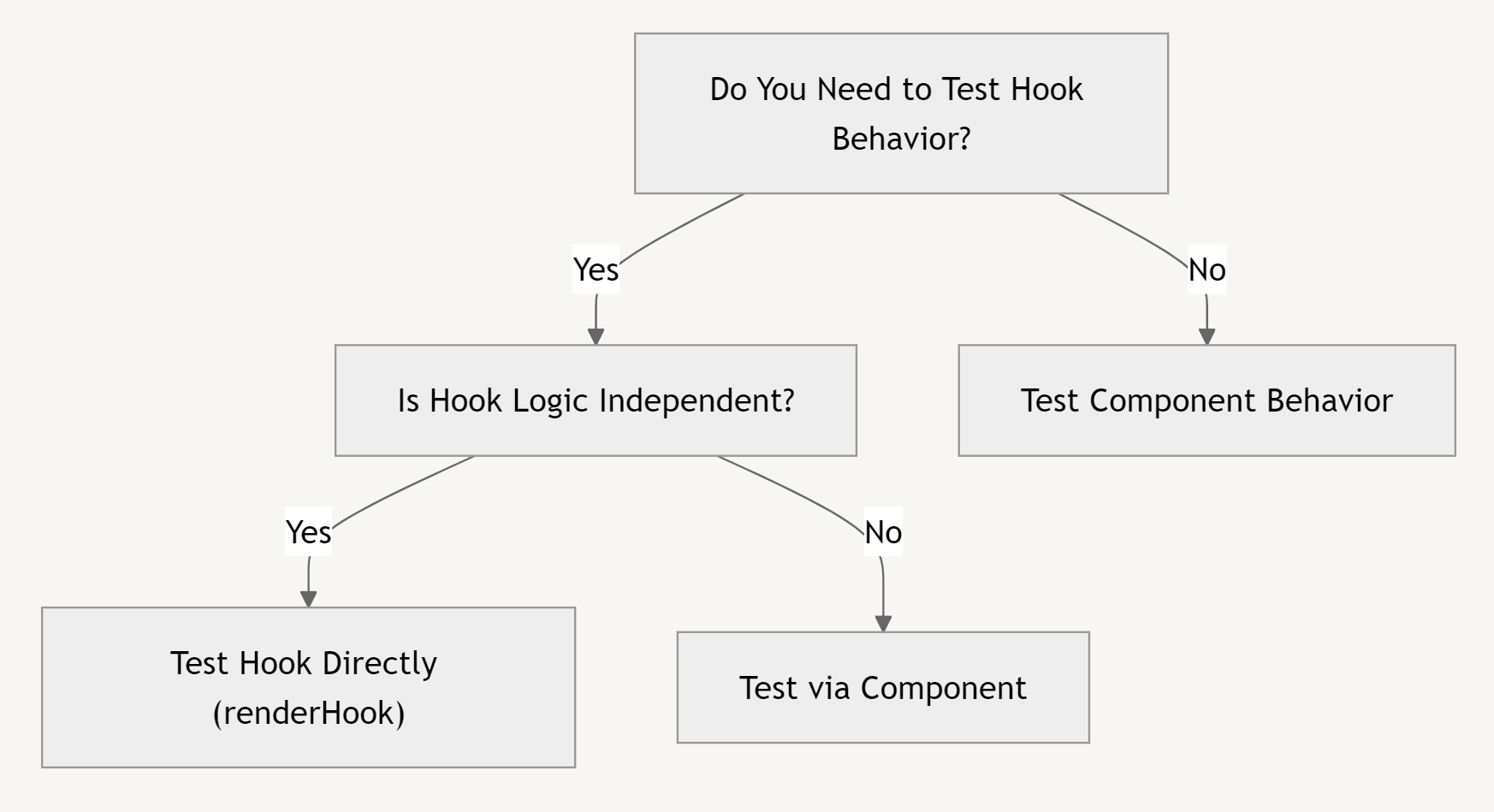
Mocking Fetch Calls in React Unit Tests
Many React applications rely on API fetch calls to display dynamic data. Mocking these API calls is essential for keeping your tests fast, reliable, and independent of external services.
jest.mock('./api', () => ({
fetchData: jest.fn(() => Promise.resolve({ data: 'mockData' }))
}));
By mocking the API with Jest, you ensure that your tests aren’t affected by network conditions or external data changes. You can simulate different responses to test how your component handles both success and failure scenarios.
Snapshot Testing for Component Renderers
Snapshot testing is particularly useful for verifying the UI output of a React component at a given point in time. Snapshots compare the current rendered output with a saved version to ensure no unexpected changes occur.import { render } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders component correctly', () => {
const { asFragment } = render(<MyComponent />);
expect(asFragment()).toMatchSnapshot();
});
Snapshots are ideal for static components where the output is consistent across renders. However, use snapshots cautiously for dynamic or frequently updated UI components, as changes to props or data may make snapshots more difficult to maintain.
Ensuring Coverage and Performance of React Unit Testing with Jest
Test Coverage in Jest
Jest provides built-in tools to measure test coverage, ensuring your unit tests cover critical parts of your React application. Coverage metrics include statements, branches, functions, and lines, offering a detailed view of which areas of your code are tested.
jest --coverage
Balancing Test Speed and Depth
As your application grows, it's essential to balance comprehensive testing with performance. Increasing test depth—covering more edge cases—can slow down execution.
The solution is to mock expensive operations (like API calls or database interactions) while ensuring core logic is thoroughly tested. This approach helps maintain fast test execution without sacrificing test quality.
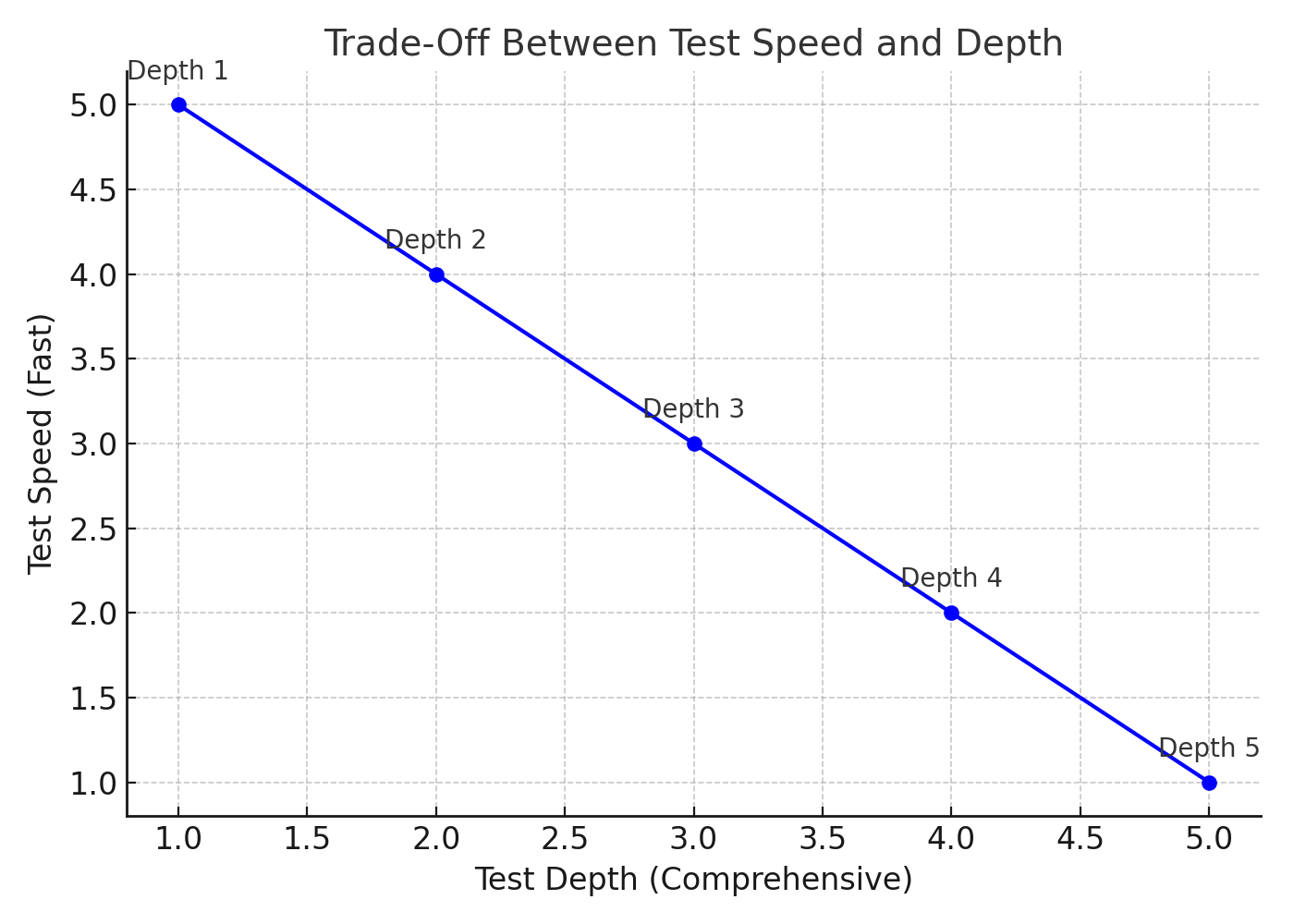
Conclusion
React unit testing with Jest and React Testing Library is crucial for ensuring the stability and scalability of your application. By focusing on testing components, hooks, API fetch calls, and rendered UI, you build a foundation for a robust and reliable codebase. Mocking API calls helps keep your tests efficient, while snapshot testing ensures UI consistency over time.
Start small and scale your testing strategy as your application grows. Consistency is key—regularly writing and maintaining tests will help ensure long-term code quality and reliability in your React projects.
>>> Follow and Contact Relia Software for more information
- testing
- Web application Development
- coding