Vite has become a cornerstone of modern web development, offering a faster experience compared to traditional bundlers. Vite, meaning "fast" in French, lives up to its name by leveraging native ES modules during development and Rollup for bundling production code.
We will explore how Vite's innovative approach to development processes allows developers to create high-performance web applications with unparalleled speed and ease. I'll also break down how to create React app using Vite with simple steps.
What is Vite?
Vite is a modern JavaScript build tool that provides a faster and more efficient development experience compared to traditional bundlers like Webpack. Vite's speed comes from a combination of architectural choices that optimize both the development and production build processes. Here are some its strong features:
- Native ES Modules During Development
Instead of bundling the entire application during development, Vite leverages native ES modules, which are supported by modern browsers. This allows the browser to request individual modules as needed, resulting in much faster server startup times and quicker updates. Essentially, it only processes code that is actually needed at that moment.
-
esbuild
Vite utilizes esbuild, an extremely fast JavaScript bundler written in Go, for dependency pre-bundling. esbuild's performance is significantly better than traditional JavaScript-based bundlers like Webpack, especially for large projects. This speeds up the process of resolving and transforming dependencies.
-
On-Demand Compilation
During development, Vite only compiles the code that is actively being used in the browser. This "on-demand" approach avoids unnecessary processing of the entire codebase, leading to faster initial loads and updates.
-
Optimized Production Builds with Rollup
For production builds, Vite uses Rollup, a highly efficient JavaScript bundler that focuses on producing optimized and lean bundles. This ensures that the final production code is as small and performant as possible.
These factors combine to create a development experience that is significantly faster and more responsive than traditional build tools.
4 Steps to Setup a Vite React App
Prerequisites
First, we will use Node.js for a demo. Vite supports Node.js version 18 or later. Besides, we also need a node package management tool such as npm, yarn or pnpm.
Step 1: Create the Vite Project
Next, open a terminal and navigate to the directory where we want to create our React project. Then, run this command:
yarn create vite your-project-name -- --template react
It will automatically generate for us an initial React source with a vite.config.js
or vite.config.ts
file in our project's root directory that allows us to customize Vite's behavior.
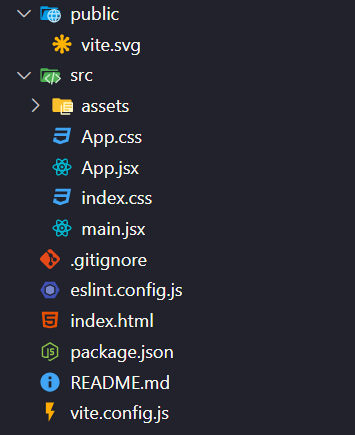
Step 2: Install Dependencies
Next step, to make the initial project work, we need to install all necessary packages. Then run:
yarn install
Step 3: Run the Development Server
Now, everything is prepared for running the app. Start the development server with the command:
yarn dev
Vite will start a development server, and you'll see a message in your terminal indicating the local URL where your app is running (usually http://localhost:5173). Try to access that URL, this is something you would see.
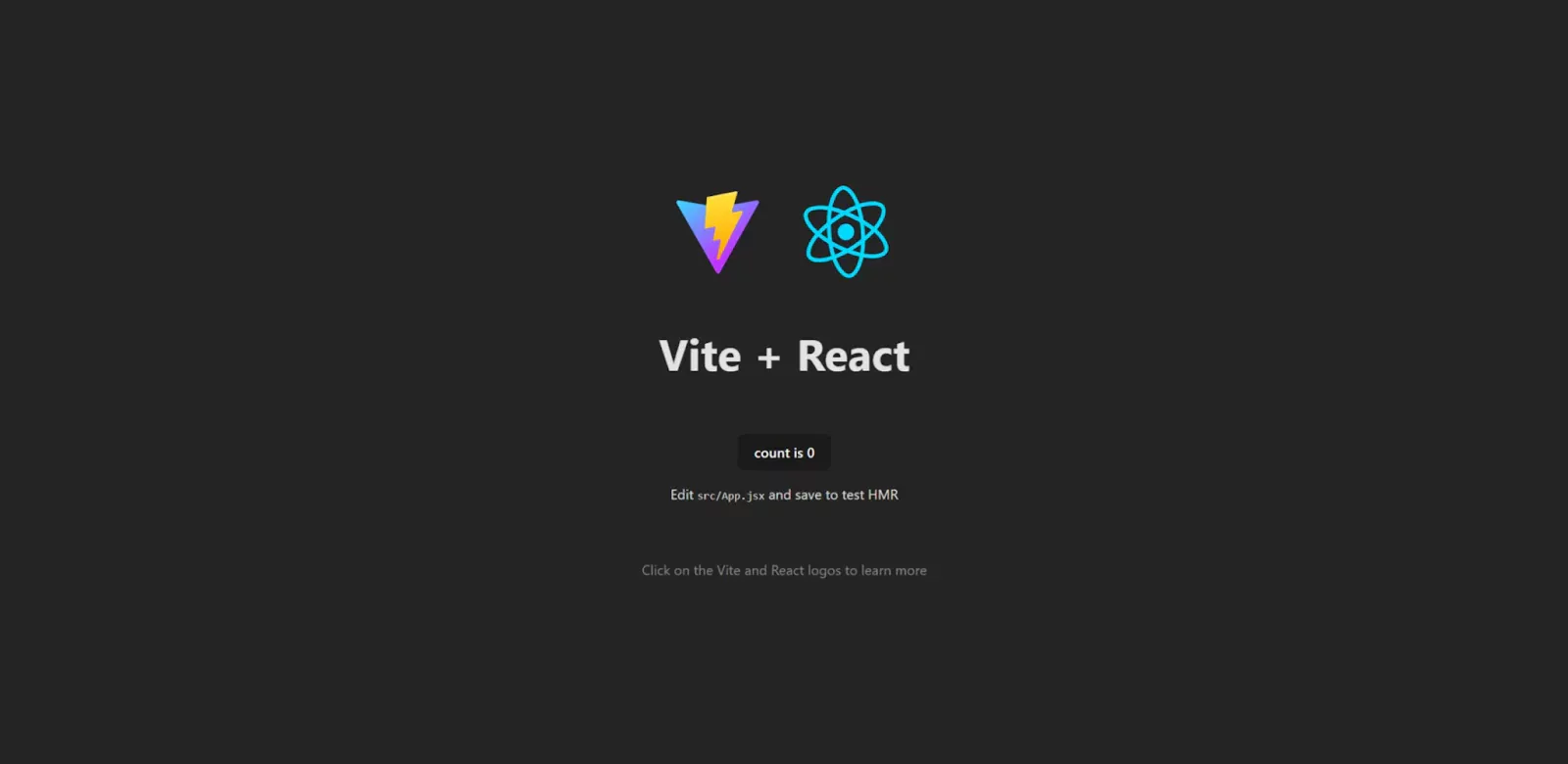
Step 4: Start coding
Seems everything is set up successfully. We can start modifying the code and building your React application. Vite's hot module replacement (HMR) will automatically update the browser as you make changes.
Vite configuration
We already started a Vite app successfully, but one very important thing we need to know is Vite configuration.
The initial Vite configuration file vite.config.js that Vite generated for us is like this:
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
// https://vite.dev/config/
export default defineConfig({
plugins: [react()],
})
Let’s try to remove everything here, only export an empty configuration.
export default {}
The server is still accessible, but nothing was rendered. If we open the browser console, we can see the error “Uncaught ReferenceError: React is not defined”. This is because this React app is configured to use Vite for bundling.
Anyway, let’s test some settings to understand what we can do with Vite.
Base URL path for the app
In some complex projects, we would like to run our app at a specific base path. For example, our React app is built as an admin dashboard, so we want the app to always be located at the URL path /admin. We can define like this:
export default {
base: '/admin/'
}
Restart the app, we can see
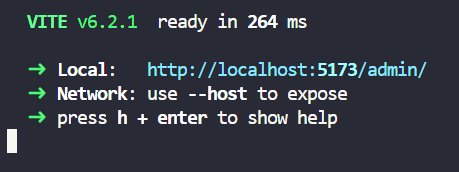
Now, our React app’s routes are on /admin base URL.
Built-in function defineConfig()
Similar to “base” settings, we also have lots of others such as “envPrefix
”, “server
”, “css
”, “preview
”, “logLevel
”, “envDir
”, etc., but it’s hard to remember all. Vite package already has a built-in function defineConfig()
that officially helps us set up Vite more easily.
Instead of the way we configured “base” above, we can write like this, pass a config object to defineConfig()
function. The app still works the same.
import { defineConfig } from 'vite'
export default defineConfig({
base: '/admin'
})
The defineConfig()
actually has other overrides for lots of use cases. It can be used by passing into a callback function.
export default defineConfig(({command, mode, isSsrBuild}) => {
console.log('Server starting:', {command, mode, isSsrBuild})
return {}
})
This code introduces 3 inputs: command
, mode
and isSsrBuild
. Here is the result including a log in the startup period if we run “yarn dev
”.
Based on these overrides, we can customize the Vite configuration for each input set. For instance, against the mode “production”, we will not set up the base URL, but regarding the mode “development”, the base URL should be “/staging
”.
Remember that we always need to return a config object for all cases.
Furthermore, defineConfig()
also accepts async callbacks.
A sample Vite configuration for the React app
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
import path from 'path'
export default defineConfig({
plugins: [react()],
resolve: {
alias: {
'@': path.resolve(__dirname, './src'), // Example: Alias '@' to the 'src' directory
'@components': path.resolve(__dirname, './src/components'), // Example: Alias '@components' to the components directory
'@pages': path.resolve(__dirname, './src/pages'), // Example: Alias '@pages' to the pages directory
'@assets': path.resolve(__dirname, './src/assets'), // Example: Alias '@assets' to the assets directory
'@hooks': path.resolve(__dirname, './src/hooks'), // Example: Alias '@hooks' to the hooks directory
'@utils': path.resolve(__dirname, './src/utils'), // Example: Alias '@utils' to the utils directory
'@services': path.resolve(__dirname, './src/services'), // Example: Alias '@services' to the services directory
},
},
server: {
port: 3000, // Change the development server port if needed
open: true, // Automatically open the browser on server start]
},
build: {
outDir: 'dist', // Output directory for the production build
sourcemap: true, // Generate sourcemaps for debugging production builds
},
})
>> Read more: Top 12 Best Javascript Frameworks for Your Projects
Conclusion
In conclusion, Vite significantly enhances the development and build processes for modern web applications. Its focus on on-demand compilation and optimized production builds translates to a faster and more efficient developer experience.
By demonstrating how to create and configure a Vite React app, we've highlighted its ease of use and flexibility. Ultimately, Vite's speed and efficiency make it a powerful tool for building high-performance web applications, streamlining workflows and accelerating development cycles.
- coding
- web development