Table of Contents
Due to its simplicity and efficiency, Golang is gaining popularity across industries. Many tech giants and startups are using Go for its reliability and performance. Golang makes scaling programs easy with built-in concurrency.
Mastering Golang requires hands-on projects. Develop your Go programming skills by applying theory and Golang project ideas to real-world situations.
>> Read more about technical blogs of Golang coding:
-
Practical SOLID in Golang: Single Responsibility Principle
In this blog, we will describe beginner-friendly to advanced Golang project ideas to help you improve your abilities and unleash your creativity in Go programming. Check it out!
Golang Project Ideas for Beginners
From designing a music player to a rudimentary web server, we'll cover basic Golang project ideas for beginners in this part. Each project lets you learn the Golang programming language while having fun and making something useful.
Command-Line To-Do List
The to-do list application will help you comprehend the notion of Golang. This project allows you to add tasks, mark them as completed, and delete them. You will grasp how the data flows, which will be useful for future projects.
It introduces you to fundamental Go features such as variables, data structures (lists), control flow (loops), and user input/output.
Learning Goals:
-
Working with variable and data kinds.
-
Using loops to iterate over tasks.
-
Using conditional expressions to add, remove, and mark completed jobs.
-
Understanding user input and output functionality.
Code examples:
package main
import (
"fmt"
"bufio"
"os"
)
func main() {
scanner := bufio.NewScanner(os.Stdin)
tasks := []string{}
for {
fmt.Println("Enter command (add, remove, complete, list, or exit):")
userInput := scanner.Scan()
if !userInput {
break
}
command := scanner.Text()
switch command {
case "add":
fmt.Println("Enter task to add:")
task := scanner.Text()
tasks = append(tasks, task)
fmt.Println("Task added successfully!")
case "remove":
fmt.Println("Enter task number to remove:")
taskNumber, err := strconv.Atoi(scanner.Text())
if err != nil {
fmt.Println("Invalid input. Please enter a number.")
continue
}
if taskNumber < 1 || taskNumber > len(tasks) {
fmt.Println("Task number out of range.")
continue
}
tasks = append(tasks[:taskNumber-1], tasks[taskNumber:]...)
fmt.Println("Task removed successfully!")
case "complete":
fmt.Println("Enter task number to mark complete:")
taskNumber, err := strconv.Atoi(scanner.Text())
if err != nil {
fmt.Println("Invalid input. Please enter a number.")
continue
}
if taskNumber < 1 || taskNumber > len(tasks) {
fmt.Println("Task number out of range.")
continue
}
fmt.Printf("Task '%s' marked complete.\n", tasks[taskNumber-1])
tasks[taskNumber-1] = "**COMPLETED** - " + tasks[taskNumber-1]
case "list":
if len(tasks) == 0 {
fmt.Println("You don't have any tasks yet.")
} else {
fmt.Println("Your tasks:")
for i, task := range tasks {
fmt.Printf("%d. %s\n", i+1, task)
}
}
case "exit":
fmt.Println("Exiting...")
return
default:
fmt.Println("Invalid command.")
}
}
}
Web Scraper
The Golang Web Scraper project seeks to provide a reliable and quick way to retrieve data from websites. It's a popular Golang project for beginners. Web scraping is the process of obtaining information from websites for use in various applications such as data analysis, monitoring, and content aggregation.
In this project, we will use the Go programming language to construct a versatile web scraper that uses concurrency to improve speed and efficiency. The scraper will be intended to handle various website structures while providing a modular and extensible architecture for future upgrades.
Building a simple web scraper is an excellent approach to learning how to make HTTP queries in Golang and interact with HTML data.
Learning Goals:
-
Understand HTTP requests and responses.
-
Parsing HTML texts with libraries such as Goquery.
-
Extracting specific data from websites.
Code examples:
package main
import (
"fmt"
"net/http"
"github.com/gocolly/colly"
)
func main() {
// Define the URL to scrape
url := "https://golang.org/doc/install"
// Create a new Colly collector
c := colly.NewCollector()
// On request response, extract title from the page
c.OnRequest(func(r *colly.Request) {
fmt.Println("Visiting", r.URL)
})
c.OnResponse(func(r *colly.Response) {
// Parse the HTML document
doc := r.Body
title := doc.Find("title").Text()
fmt.Println("Title:", title)
})
// Visit the URL
err := c.Visit(url)
if err != nil {
fmt.Println("Error:", err)
}
}
Music Player
Multimedia applications offer both challenges and rewards. The Music Player project challenges you to create a simple music player with GoLang. You will gain practical experience processing multimedia files, designing a user-friendly interface, and implementing basic playing controls. This project combines creative and technical skills, making it ideal for beginners.
Learning Goals:
-
Learn how to access and manipulate audio files using Golang functionalities.
-
Explore libraries like go-audio or opus to handle audio playback.
-
Learn how to accept user commands (e.g., play, pause) and provide feedback through the console or a UI (user interface).
-
If using a GUI library, you'll gain experience with building a simple graphical interface for user interaction.
Code examples:
package main
import (
"fmt"
"github.com/hajimehos/go-audio"
"os"
)
func main() {
// Prompt user for file path
fmt.Println("Enter the path to your audio file:")
var filePath string
fmt.Scanln(&filePath)
// Open the audio file
f, err := os.Open(filePath)
if err != nil {
fmt.Println("Error opening file:", err)
return
}
defer f.Close()
// Decode audio data using go-audio
d, err := audio.NewDecoder(f)
if err != nil {
fmt.Println("Error decoding audio:", err)
return
}
defer d.Close()
// Create a new audio player
p := audio.NewPlayer()
// Start playback
play := true
for play {
out := p.Play(d.Next())
if out.Err != nil {
fmt.Println("Error playing audio:", out.Err)
break
}
// Handle user input for pause/resume/stop (optional)
fmt.Println("Enter 'p' to pause, 'r' to resume, or 's' to stop:")
var command string
fmt.Scanln(&command)
switch command {
case "p":
p.Pause()
case "r":
p.Resume()
case "s":
play = false
default:
fmt.Println("Invalid command. Please enter 'p', 'r', or 's'.")
}
}
fmt.Println("Playback finished.")
}
Basic Web Server
In this interesting project, you will learn how to develop a small web service using the Golang programming language and Java. By completing this project, you will learn how to develop web service endpoints that handle web requests. You will also be able to view and delete URL Query string request parameters.
Learning Goals:
-
Learn the fundamentals of HTTP servers in Golang.
-
Implementing routing to handle various queries.
-
Serving static content (HTML, CSS, and JavaScript language).
Code examples:
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, World!")
}
func main() {
http.HandleFunc("/", handler)
fmt.Println("Server listening on port 8080")
http.ListenAndServe(":8080", nil)
}
File Encryption Tool
This project creates a program for encrypting and decrypting files using symmetric encryption techniques such as AES. This project introduces newcomers to file handling, encryption mechanisms, and error handling in Go.
Learning Goals:
-
Working with Golang files (read and write).
-
Understanding fundamental cryptographic ideas (symmetric encryption).
-
Using encryption/decryption packages (such as crypto/aes).
Code examples:
package main
import (
"crypto/aes"
"crypto/cipher"
"crypto/rand"
"encoding/base64"
"fmt"
"io/ioutil"
)
func encryptFile(key []byte, filepath string) error {
plaintext, err := ioutil.ReadFile(filepath)
if err != nil {
return err
}
block, err := aes.NewCipher(key)
if err != nil {
return err
}
// Use CBC mode with random initialization vector (IV)
iv := make([]byte, aes.BlockSize)
if _, err := rand.Read(iv); err != nil {
return err
}
cfb := cipher.NewCBCEncrypter(block, iv)
ciphertext := cfb.CryptBlocks(make([]byte, aes.BlockSize), plaintext)
ciphertext = append(iv, ciphertext...)
encodedCiphertext := base64.StdEncoding.EncodeToString(ciphertext)
return ioutil.WriteFile(filepath+".enc", []byte(encodedCiphertext), 0644)
}
func decryptFile(key []byte, filepath string) error {
ciphertext, err := ioutil.ReadFile(filepath)
if err != nil {
return err
}
decodedCiphertext, err := base64.StdEncoding.DecodeString(string(ciphertext))
if err != nil {
return err
}
block, err := aes.NewCipher(key)
if err != nil {
return err
}
iv := decodedCiphertext[:aes.BlockSize]
ciphertext = decodedCiphertext[aes.BlockSize:]
cfb := cipher.NewCBCDecrypter(block, iv)
plaintext := cfb.CryptBlocks(make([]byte, aes.BlockSize), ciphertext)
return ioutil.WriteFile(filepath+".dec", plaintext, 0644)
}
func main() {
// Replace with your desired password
password := "your_secret_password"
key := []byte(password)
// Specify the file to encrypt/decrypt
filepath := "your_file.txt"
// Choose between encryption or decryption
action := "encrypt" // or "decrypt"
if action == "encrypt" {
err := encryptFile(key, filepath)
if err != nil {
fmt.Println("Error encrypting file:", err)
return
}
fmt.Println("File encrypted successfully!")
} else if action == "decrypt" {
err := decryptFile(key, filepath+".enc")
if err != nil {
fmt.Println("Error decrypting file:", err)
return
}
fmt.Println("File decrypted successfully!")
} else {
fmt.Println("Invalid action. Please choose 'encrypt' or 'decrypt'.")
}
}
Intermediate Golang Projects
Ready to improve Golang? These intermediate project ideas will challenge you and solidify your understanding. These intermediate projects will provide a rewarding journey as you expand your Golang expertise.
RESTful API Development
Modern web development includes API development, therefore a consistent technique to design internet-connected online services is needed. REST is an architectural style that emphasizes stateless client-server communication, standardized interfaces, and established actions to manage resources. Scalability, client-friendliness, and flexibility make RESTful APIs ideal for distributed systems, mobile apps, and web services.
Building a RESTful API with database integration allows you to experiment with web service development and data interaction.
Learning Goals:
-
Understanding the RESTful API principles and creating endpoints.
-
Working with databases such as PostgreSQL and MySQL via Golang drivers.
-
Implementing CRUD operations (Create, Read, Update, and Delete) for data manipulation.
Code examples:
package main
import (
"database/sql"
"encoding/json"
"fmt"
"net/http"
_ "github.com/lib/pq" // PostgreSQL driver
)
type Book struct {
ID int `json:"id"`
Title string `json:"title"`
Author string `json:"author"`
}
func main() {
// Connect to the database
db, err := sql.Open("postgres", "user=db_user password=db_password dbname=books_db sslmode=disable")
if err != nil {
panic(err)
}
defer db.Close()
// Define routes for API endpoints
http.HandleFunc("/books", getBooks)
http.HandleFunc("/books/", getBookByID)
fmt.Println("Server listening on port 8080")
http.ListenAndServe(":8080", nil)
}
func getBooks(w http.ResponseWriter, r *http.Request) {
// Fetch all books from the database
rows, err := db.Query("SELECT * FROM books")
if err != nil {
// Handle error
}
defer rows.Close()
var books []Book
for rows.Next() {
var book Book
err := rows.Scan(&book.ID, &book.Title, &book.Author)
if err != nil {
// Handle error
}
books = append(books, book)
}
// Encode books data as JSON
jsonData, err := json.Marshal(books)
if err != nil {
// Handle error
}
w.Header().Set("Content-Type", "application/json")
w.Write(jsonData)
}
// Implement functions for getBookByID and other API endpoints
Web Application with User Authentication
Web applications enable online engagement and task completion in browsers. Their range from HTML pages to complicated systems include social networking sites like Facebook and e-commerce sites like Amazon.
Web apps use numerous programming languages and frameworks to provide dynamic content and interaction and connect to databases to store and retrieve data. They simplify online shopping, social media, banking, and more. These have responsive design and server-side processing for seamless user experiences that form today's digital landscape.
Building a web-based app with authentication for users allows you to learn about user registration, login, and managing sessions.
Learning Goals:
-
Adding user registration and login functionality.
-
Secure storage and management of user sessions.
-
Protecting routes and resources based on user roles.
Code examples:
package main
import (
"fmt"
"net/http"
"github.com/gorilla/sessions"
)
var sessionStore *sessions.CookieStore
func main() {
// Configure session store
sessionStore = sessions.NewCookieStore([]byte("secret-key-here"))
// Define routes for login, register, and protected pages
http.HandleFunc("/login", loginHandler)
http.HandleFunc("/register", registerHandler)
http.HandleFunc("/protected", protectedHandler)
fmt.Println("Server listening on port 8080")
http.ListenAndServe(":8080", nil)
}
func loginHandler(w http.ResponseWriter, r *http.Request) {
// Validate user credentials
// ...
// Create user session on successful login
session, err := sessionStore.Get(r, "user-session")
if err != nil {
// Handle error
>> Read more: An Ultimate Guide To Web Application Security for Businesses
Chat Application
The chat software allows real-time conversation. It uses Golang's concurrency features to handle numerous users and provide real-time messaging. WebSocket enables real-time server-client communication for web-based chat applications, delivering messages instantly.
This project will teach you the foundations of networking in GoLang. With this simple program, you'll learn how to connect two or more devices, send and receive messages, and handle communication protocols. It will expand your networking knowledge, concurrency, and real-time communication techniques.
Learning Goals:
-
Understanding concurrency patterns (such as channels and goroutines) in real-time communication.
-
Implementing message broadcasting to linked users.
-
Exploring permanent connection technologies such as WebSockets.
Code examples:
package main
import (
"fmt"
"sync"
)
type message struct {
Content string
User string
}
var messages chan message
var mutex sync.Mutex
func main() {
messages = make(chan message)
go broadcaster()
// Handle user connections and message sending
// ...
fmt.Println("Chat server started")
}
func broadcaster() {
for {
msg := <-messages
mutex.Lock()
// Broadcast message to all connected users
mutex.Unlock()
}
}
func sendMessage(msg message) {
mutex.Lock()
messages <- msg
mutex.Unlock()
}
File Sharing Tool
Develop a file-sharing platform that enables users to securely upload and retrieve files. Incorporate functionalities such as access control, expiration links, and file encryption. Go is utilized for web development, file management, and security procedures in this endeavor.
This initiative enables the investigation of functionalities such as:
-
Files are uploaded to a server.
-
Performing file downloads from the server.
-
Authorization and authentication of users.
-
Secure storage of files.
Learning Goals:
-
Using HTTP to send and receive messages.
-
Adding the ability to share and download files.
-
Keeping user information and maybe even file details in a database.
-
Looking into ways to authenticate and authorize users.
Code examples:
package main
import (
"fmt"
"io/ioutil"
"net/http"
"github.com/gorilla/mux"
)
type File struct {
ID string `json:"id"`
Filename string `json:"filename"`
ContentType string `json:"content_type"`
// ... add other relevant file metadata (optional)
}
var files []File // Store uploaded files (replace with database later)
func uploadHandler(w http.ResponseWriter, r *http.Request) {
// Parse uploaded file
file, header, err := r.FormFile("file")
if err != nil {
fmt.Println("Error parsing uploaded file:", err)
w.WriteHeader(http.StatusBadRequest)
return
}
defer file.Close()
// Read file content
fileBytes, err := ioutil.ReadAll(file)
if err != nil {
fmt.Println("Error reading uploaded file:", err)
w.WriteHeader(http.StatusInternalServerError)
return
}
// Store file information (replace with database storage later)
newFile := File{
ID: fmt.Sprintf("file-%d", len(files)),
Filename: header.Filename,
ContentType: header.Header.Get("Content-Type"),
}
files = append(files, newFile)
// Save file data (replace with secure storage later)
err = ioutil.WriteFile("uploads/"+newFile.ID, fileBytes, 0644)
if err != nil {
fmt.Println("Error saving uploaded file:", err)
w.WriteHeader(http.StatusInternalServerError)
return
}
fmt.Fprintf(w, "File uploaded successfully: %s", newFile.Filename)
}
func downloadHandler(w http.ResponseWriter, r *http.Request) {
vars := mux.Vars(r)
fileID := vars["id"]
// Find file by ID (replace with database lookup later)
var foundFile *File
for _, file := range files {
if file.ID == fileID {
foundFile = &file
break
}
}
if foundFile == nil {
fmt.Fprintf(w, "File not found: %s", fileID)
w.WriteHeader(http.StatusNotFound)
return
}
// Read file data (replace with secure storage retrieval later)
fileBytes, err := ioutil.ReadFile("uploads/" + fileID)
if err != nil {
fmt.Println("Error reading file:", err)
w.WriteHeader(http.StatusInternalServerError)
return
}
// Set headers for download
w.Header().Set("Content-Disposition", fmt.Sprintf("attachment; filename=%s", foundFile.Filename))
w.Header().Set("Content-Type", foundFile.ContentType)
w.Write(fileBytes)
}
func main() {
router := mux.NewRouter()
router.HandleFunc("/upload", uploadHandler).Methods(http.MethodPost)
router.HandleFunc("/download/{id}", downloadHandler).Methods(http.MethodGet)
fmt.Println("Server listening on port 8080")
http.ListenAndServe(":8080", router)
}
Work Schedule
Create a scheduler that runs jobs at set intervals. Users can create, schedule, and track tasks. The project covers Go concurrency, scheduling techniques, and task management. Explore features like:
-
Create and manage employee profiles.
-
Set start/end times and recurrent patterns for shifts.
-
Setting employee timetables.
-
Implementing a UI for interaction.
Learning Goals:
-
Using maps or slices to manage employee and schedule data.
-
Learning time manipulation libraries like time packages for scheduling.
-
Building a tool RESTful API with Gin or Echo.
Code examples:
package main
import (
"fmt"
"time"
)
type Employee struct {
ID string `json:"id"`
Name string `json:"name"`
// ... add other relevant employee information
}
type Shift struct {
Start time.Time `json:"start"`
End time.Time `json:"end"`
Weekday time.Weekday `json:"weekday"` // optional for recurring schedules
// ... add other relevant schedule information (e.g., break times)
}
var employees map[string]Employee = make(map[string]Employee)
var schedules map[string]Shift = make(map[string]Shift)
var employeeSchedules map[string][]string = make(map[string][]string) // Employee ID -> list of assigned schedule IDs
func addEmployee(employee Employee) {
employees[employee.ID] = employee
}
func createShift(shiftID string, shift Shift) {
schedules[shiftID] = shift
}
func assignSchedule(employeeID, shiftID string) error {
if _, ok := employees[employeeID]; !ok {
return fmt.Errorf("employee with ID %s not found", employeeID)
}
if _, ok := schedules[shiftID]; !ok {
return fmt.Errorf("shift with ID %s not found", shiftID)
}
employeeSchedules[employeeID] = append(employeeSchedules[employeeID], shiftID)
return nil
}
func getEmployeeSchedule(employeeID string) ([]Shift, error) {
if _, ok := employees[employeeID]; !ok {
return nil, fmt.Errorf("employee with ID %s not found", employeeID)
}
var assignedShifts []Shift
for _, shiftID := range employeeSchedules[employeeID] {
assignedShifts = append(assignedShifts, schedules[shiftID])
}
return assignedShifts, nil
}
func main() {
// Add sample employees and schedules (replace with persistent storage)
addEmployee(Employee{ID: "emp-1", Name: "John Doe"})
createShift("shift-1", Shift{Start: time.ParseHour("15:00", "15:00"), End: time.ParseHour("18:00", "18:00"), Weekday: time.Monday})
// Assign schedule to employee
err := assignSchedule("emp-1", "shift-1")
if err != nil {
fmt.Println("Error assigning schedule:", err)
return
}
// Get employee schedule
assignedShifts, err := getEmployeeSchedule("emp-1")
if err != nil {
fmt.Println("Error getting employee schedule:", err)
return
}
fmt.Println("Employee Schedule:")
for _, shift := range assignedShifts {
fmt.Printf(" - Start: %s, End: %s\n", shift.Start.Format("15:04"), shift.End.Format("15:04"))
}
}
Advanced Golang Project Ideas
Ready for a challenge after mastering the basics? These sophisticated Golang project ideas will challenge you and introduce new ideas. These tasks require extensive expertise yet give great learning and the satisfaction of creating something amazing.
Customer Relationship Management System (CRM)
Have you heard of GOCRM, a customer relationship management system? You can create your own enterprise-level customer relationship management system. GOCRM is a paid platform, hence the template and code are not publicly available online. Avoid replicating existing features or concepts and instead aim to create something unique.
Learning Goals:
-
Learning complicated system design principles by developing a CRM multi-layered architecture.
-
Practice Golang database drivers for your database skills.
-
Build RESTful APIs for CRM functionality using Golang web frameworks.
-
(Optional) Building a user interface with web frameworks lets you create a dynamic and interactive experience.
Code examples:
package main
import (
"encoding/json"
"fmt"
"net/http"
"github.com/gorilla/mux"
"github.com/jinzhu/gorm"
)
type User struct {
gorm.Model
Username string `json:"username"`
Password string `json:"password"` // hashed password should be stored
Role string `json:"role"` // e.g., "admin", "sales_rep"
}
func registerUser(w http.ResponseWriter, r *http.Request) {
// Parse incoming user data from request body
var newUser User
err := json.NewDecoder(r.Body).Decode(&newUser)
if err != nil {
fmt.Println("Error decoding user data:", err)
w.WriteHeader(http.StatusBadRequest)
return
}
// Hash password before storing (implement hashing logic)
// ...
// Connect to database (replace with your connection details)
db, err := gorm.Open("postgres", "user=your_user password=your_password dbname=crm_db sslmode=disable")
if err != nil {
fmt.Println("Error connecting to database:", err)
w.WriteHeader(http.StatusInternalServerError)
return
}
defer db.Close()
// Create new user record in the database
result := db.Create(&newUser)
if result.Error != nil {
fmt.Println("Error creating user:", result.Error)
w.WriteHeader(http.StatusInternalServerError)
return
}
// Send successful registration response
w.WriteHeader(http.StatusCreated)
fmt.Println("User registered successfully!")
}
func main() {
router := mux.NewRouter()
router.HandleFunc("/register", registerUser).Methods(http.MethodPost)
// ... add other routes for login, etc.
fmt.Println("Server listening on port 8080")
http.ListenAndServe(":8080", router)
}
Real-Time Data Processing Application
Starting with real-time data stream processing, continuous data streams are analyzed, processed, and responded to immediately. Go real-time data stream processing can be implemented using Gorilla WebSocket for web socket connections, Go channels for concurrency, and Kafka or NATS for message brokering.
Building a real-time data processing application teaches you about streaming data pipelines and distributed processing frameworks.
Learning Goals:
-
Understanding streaming data principles (such as Apache Kafka and Apache Pulsar).
-
Setting up data pipelines for real-time processing and analysis.
-
Exploring distributed processing frameworks such as Apache Flink and Apache Spark.
Code examples:
package main
import (
"fmt"
"github.com/segmentio/kafka-go"
)
func main() {
// Configure Kafka consumer connection
reader := kafka.NewReader(kafka.ReaderConfig{
Brokers: []string{"localhost:9092"},
Topic: "my-topic",
GroupID: "my-group",
ClientID: "my-consumer",
})
for {
msg, err := reader.ReadMessage()
if err != nil {
fmt.Println("Error reading message:", err)
break
}
fmt.Printf("Message received: key = %s, value = %s\n", string(msg.Key), string(msg.Value))
}
reader.Close()
}
>> Read more: Type Conversion in Golang: How To Convert Data Types in Go?
Artificial Intelligence Bot
With the help of Go, making an artificial intelligence (AI) bot will help you improve your skills. You will learn how to use Microservices, API interaction, and JSON in Go through this project.
Building a basic machine-learning platform and AI framework allows you to experiment with essential machine-learning/AI techniques and model training in Golang.
Learning Goals:
-
Understanding key machine learning algorithms (such as linear regression and decision trees).
-
Creating data structures to represent and manipulate training data.
-
Machine learning models are trained and evaluated using Golang.
Code examples:
package main
import (
"fmt"
"strings"
)
var greetings = []string{
"Hi there!",
"Hello!",
"How can I help you today?",
}
var responses map[string][]string = map[string][]string{
"hello": greetings,
"hi": greetings,
"how are you?": {
"I'm doing well, thanks for asking!",
"I'm here to assist you. What can I do?",
},
}
func main() {
for {
var userInput string
fmt.Println("You: ")
fmt.Scanln(&userInput)
userInput = strings.ToLower(userInput)
var response string
// Check for predefined keywords
for keyword, options := range responses {
if strings.Contains(userInput, keyword) {
response = options[rand.Intn(len(options))]
break
}
}
// Handle no match (optional, add more responses)
if response == "" {
response = "Sorry, I don't understand. Try asking something else."
}
fmt.Println("Chatbot: ", response)
}
}
>> Read more:
-
Top 12 Best Free AI Chatbots for Businesses in 2024
-
What is Generative AI? 5 Best Generative AI Tools in 2024
Blockchain Implementation
Building a blockchain from scratch including proof-of-work consensus, transaction validation, and peer-to-peer networking. This project explores encryption, distributed systems, and Go-based decentralized application development.
Learning Goals:
-
Solid grasp of blockchain hashing, consensus techniques, and distributed ledgers.
-
Exploring crypto/sha256 for hashing and digital signature libraries.
-
Implementing blockchain data structures and algorithms.
Code examples:
package main
import (
"crypto/sha256"
"encoding/hex"
"time"
)
type Block struct {
Index int `json:"index"`
Timestamp time.Time `json:"timestamp"`
Data []byte `json:"data"`
Hash string `json:"hash"`
PrevHash string `json:"prevHash"`
}
func (b *Block) CalculateHash() {
hash := sha256.Sum256(append([]byte(fmt.Sprintf("%d", b.Index)), []byte(b.PrevHash), b.Data...))
b.Hash = hex.EncodeToString(hash[:])
}
func CreateBlock(prevBlock *Block, data []byte) *Block {
newBlock := &Block{
Index: prevBlock.Index + 1,
Timestamp: time.Now(),
Data: data,
PrevHash: prevBlock.Hash,
}
newBlock.CalculateHash()
return newBlock
}
>> Read more:
-
Top 9 Best Full-Stack Project Ideas for 2024
How To Successfully Develop Golang Project Ideas?
Writing code is not the only need for successfully implementing a Go project. Here are some suggestions to help you manage the process effectively:
-
Start with defined objectives: To keep your project focused and on track, define defined goals and functionalities.
-
Break it down: Divide your project into smaller, smaller tasks and tackle them one at a time.
-
Libraries and frameworks: Utilize existing libraries and frameworks to accelerate development and assure dependability.
-
Test continuously: Use automated testing to detect defects early on and assure project stability.
-
Document your code: Make sure your code is adequately documented so that you and others can understand and maintain it.
-
Seek comments: Communicate your progress to others and ask for comments to identify areas for growth.
-
Stay persistent: Complex initiatives may meet obstacles, but perseverance pays dividends.
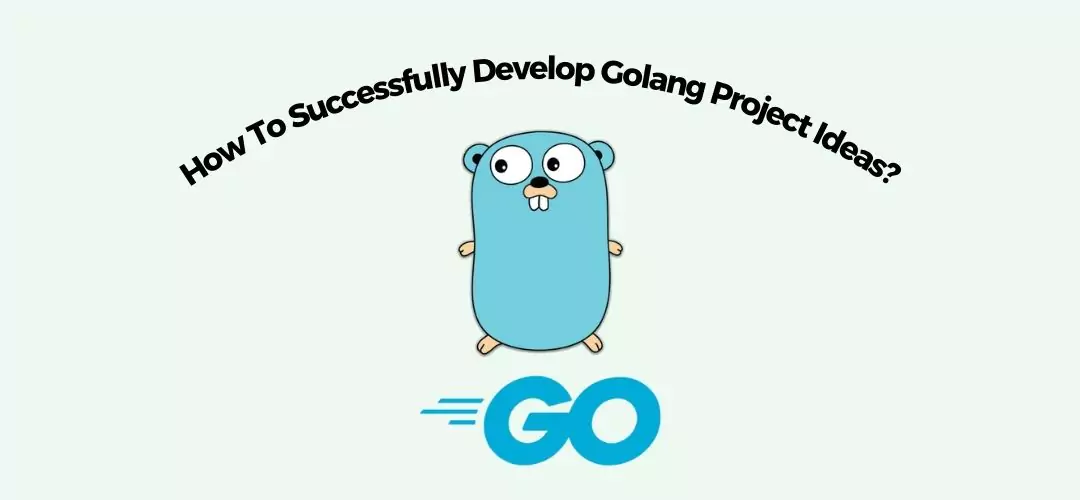
>> Read more about technical blogs of Golang coding:
-
Detailed Code Examples of Dependency Inversion Principle in Go
-
Comprehending Arrays and Slices in the Go Programming Language
-
What's New in Go 1.21 Release? Exploring Three Handy Functions
-
Practical Guide to Dependency Injection in Go: Hands-On Implementation
Conclusion
Golang project ideas offer a rewarding journey for programmers at all skill levels. Golang projects foster innovation, creativity, and skill development for beginners and experienced developers. Hands-on learning, problem-solving, and teamwork can help you learn Golang and advanced software development.
Let's get in, explore, and turn your project concepts into practical solutions that enrich programming!
>>> Follow and Contact Relia Software for more information!
- development